Question
Assignment overview In this assignment, you will implement a simple game of Battleship. If you are unfamiliar with the game Battleship, there are tutorials online
Assignment overview
In this assignment, you will implement a simple game of Battleship. If you are unfamiliar with the game Battleship, there are tutorials online describing the game. In short, there are two players that each have a 10 by 10 grid where ships are placed. The players take turns taking shots at each others ships. A player wins when they have shot at all spaces on their opponents grid that are occupied by ship. To sink a ship, you must attack every cell that the ship occupies. In this assignment, we will create Battleship that any number of players (>= 2) can play, with any sized two dimensional grids. Do to this, you will implement the following the classes:
- Gameboard:
- Tile
- Game
Keep all of your methods short and simple. In a properly-written object-oriented program, the work is distributed among many small methods, which call each other. Most of the methods you write in this program should be less than 10 lines of code.
Unless specified otherwise, all instance variables must be private, and all methods listed should be public. You may write any private helper methods you deem appropriate to solve the problems. You should not need any more accessor or mutator methods than are listed.
Testing Your Coding
We have provided sample test files for each phase to help you see if code is working according to the assignment specifications. These files are starting points for testing your code. Part of developing your skills as a programmer is to think through additional important test cases and to write your own code to test these cases.
Phase 1: Gameboard and Tiles
First, implement two simple classes: a Tile class and a Gameboard class.
The Tile class is a representation of a single space on the board. The Tile class should have:
- Three instance variables: wasAttacked, hasShip, and displayLetter. Instance variable wasAttacked stores whether or not an attack was made on this Tile. Instance variable hasShip stores true if there is a ship placed on this Tile. Finally, displayLetter is the char potentially shown by the Tile. The way the tiles are displayed is described below.
- Constructor Tile() that sets displayLetter to the character ~, which represents a wave. Note that the constructor takes no parameters.
- Method setDisplayLetter(char letter). This is a mutator/setter method for displayLetter.
ASSIGNMENT 2: Multi-dimensional arrays COMP 1020 Winter 2019
Page 2 of 7
- A getDisplay(boolean isOpponent) instance method. The method returns the character, as a String, to display when showing this tile to the user. o If this gameboard is the players (that is, isOpponent == false) Show the character saved in displayLetter if it has not been attacked, and has a ship.
- Show a wave (~) if this tile has not been attacked, and does not have a ship.
- Show a splash (^) if this tile does not have a ship, and has been attacked.
- Show an explosion (*) if this tile has been attacked, and does have a ship.
- o If this gameboard belongs to the opponent. We do not show the ship locations for opponent boards. Show a wave (~) if this tile has not been attacked.
- Show a splash (^) if this tile does not have a ship, and has been attacked.
- Show an explosion (*) if this tile has been attacked, and does have a ship.
- Method attack() which sets wasAttacked to true.
- Method canPlaceShip(), that returns a boolean. The method returns true if a ship could be placed on this Tile. A ship can be placed if this tile has not been attacked, and does not have a ship placed on it already.
- Method activeShip(), that returns true if there is a ship on this tile, and it has not been attacked.
The Gameboard class is a two-dimensional array of Tile objects, creating a game board for a single player. Complete the following:
- Gameboard has two instance variables. A two-dimensional array of Tile objects for the gameboard, and a boolean isOpponent that stores whether or not this is a player board, or an opponent board.
- A Constructor that accepts three values: int row the number of rows, int column the number of columns, and boolean isOpponent true if this board is owned by your opponent.
- A toString() method which returns a string representation of the entire gameboard. If this is an opponents board, then the ship locations should not be shown.
- Method getRow(int rowNum), which fetches row rowNum from the board, returning it as a String. The returned String should not have a newline character in it. Use class Tiles getDisplay method to fetch the values from the Tiles, not showing the opponent ship locations to the user. This method is used to extract a single row from the gameboard for displaying to the user.
- Method doAttack(int row, int column). This method sets the isAttacked flag on the tile at index row, column.
Sample output from A2TestPhase1.java:
Should be a wave ~: ~
Should be a splash ^: ^
Should be a splash ^: ^
Should be a C: C
Should be a ~: ~
Should be an explosion *: *
Print a 2x2 board of waves ~:
~~
~~
Should have a splash ^ on second row, second column
~~
~^
Should be ^~ : ^~
Should be ~^ : ~^
Print splashes down the x=y axis of a 3x3 board:
^~~
~^~
~~^
public class A2TestPhase1 {
public static void main(String[] args) { Tile emptyTile = new Tile(); System.out.println("Should be a wave ~: " + emptyTile.getDisplay(false)); emptyTile.attack(); System.out.println("Should be a splash ^: " + emptyTile.getDisplay(false)); System.out.println("Should be a splash ^: " + emptyTile.getDisplay(true)); Tile tileWithShip = new Tile(); tileWithShip.setDisplayLetter('C'); System.out.println("Should be a C: " + tileWithShip.getDisplay(false)); tileWithShip.setDisplayLetter('~'); System.out.println("Should be a ~: " + tileWithShip.getDisplay(true)); tileWithShip.attack(); System.out.println("Should be an explosion *: " + tileWithShip.getDisplay(false)); Gameboard myBoard = new Gameboard(2, 2, false); System.out.println("Print a 2x2 board of waves ~:"); System.out.println(myBoard); myBoard.doAttack(1, 1); System.out.println("Should have a splash ^ on second row, second column"); System.out.println(myBoard); myBoard.doAttack(0, 0); System.out.println("Should be ^~ : " + myBoard.getRow(0) ); System.out.println("Should be ~^ : " + myBoard.getRow(1) ); Gameboard theirBoard = new Gameboard(3, 3, true); theirBoard.doAttack(0, 0); theirBoard.doAttack(1, 1); theirBoard.doAttack(2, 2); System.out.println("Print splashes down the x=y axis of a 3x3 board:"); System.out.println(theirBoard); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
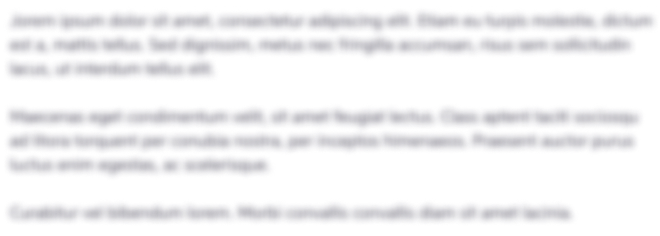
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started