Question
Assignment Three Details Forthisproject youaregoingtofurtherdevelop yourassignmenttwocode. For starter, implement a searching and sorting method in your completed assignment two program so user cansearchandsorttherecords.Youneedtoextend theassignmenttwomenuoptions to
Assignment Three Details
Forthisproject youaregoingtofurtherdevelop yourassignmenttwocode.
- For starter, implement a searching and sorting method in your completed assignment two program so user cansearchandsorttherecords.Youneedtoextend theassignmenttwomenuoptions to add searching and sorting option and then program searching, and sorting method linked to the new menuoptions. You shouldensure at leastone student's record has been entered and giveanappropriateerror messageifittherearenostudents recordenteredandforthesorting option you must ensure at least twostudent records have been entered.
- Search forastudent recordbasedontheirnameandID.Youcanjustuseasimple linearsearchwhichwillbecaseinsensitive.Ifthesearch issuccessful display the details aboutthe student record as below. Ifthe search is unsuccessful display an appropriate message.
- youwillsorttherecords alphabetically(ignorecase)bythestudent'snameandID, you can use any sorting algorithm which you like, do not use any in-built sort methods. Display the sorted list after the sort is finished.
- After finish codingsearching and sorting, identify three more functionalitiesthat may improve the grading system. For example, you may develop a unit/course/enrolment functionalitytolinkitwiththegrading system.Explain eachofthefunctionalitiesin details and what improvements would they bring to the system.
- Preparepseudocodefortheproposed threefunctionalities
- Basedonpseudocodebuiltjavacodetointegrate thesefunctionalitiesinyourassignmenttwo application. Forexample, anenrolment classcan be created that will allow studentsto record their enrolled units, status, and respective grade (if applicable). Please note, these new functionalities must be accessible by the users via themain menu of your application.
Thinkofnewfunctionalities
You willneed tothink ofagrademanagement systemofauniversity thatrequires furtherdetailslike enrolment, study progress, course information and payment status. These new functionalities ideally willrequire codeextensionlikeimplementingnewclass,methods, userinputandmewmenuoptions. Talk with your tutor if you are unsure of what scenario to use.
Yourextendedfunctionalitiesmustlinkwiththegradingsysteminassignmenttwo.
Somegenericguidelinesfornewfunctionalities-
Likeassignment-1,yourprogramwillneedtoreadinNentries whereNisthelargest digitofyour student ID, or single entries at a time in a menu system.
For each entry you need to read in at least a String or more if it is required and read in a number or numbers to perform a calculation. The calculation should be dependent on a series of if else statements which depends on a variation of any criteria such as. enrolment, grades or paid/unpaid fees. If itisjustacalculation perentrythen useifelsestatements toreport ontheresult, forexample: astudent is unabletoenroll to aunitifhe/shedidnotpass the prerequisiteunit. Anotherexample can be: a student's Moodle access will be restricted if he/she doesn't pay the tuition fees on time.
After the entries have been entered, you will need to report an average of some value (it could be the averageofgrades forexample). Forinstance, youmayshowinaunit (sayCOIT20245)whatpercentage of total students passed the unit, got HD etc.
Thesecouldbedisplayedinadisplay all optionifyouareusingamenuinterface.
Yourprogramneedstodisplaytheprinciplesofgoodprogrammingpractices:meaningfulidentifiers using camel notation, correct indentation and layout, constants used for numeric values and correct commenting including a header comment for all files if applicable.
Note: Forthisproject youaregoingtofurtherdevelop yourcompleted assignmenttwocode and you can find assignment two code given below:
Studen.java
public class Student { private String studentName; private String studentID; private double assignmentOneMarks; private double assignmentTwoMarks; private double projectMark; private double individualTotalMarks; private String grade; final int PASS = 50; final int CREDIT = 65; final int DISTINCTION = 75; final int HIGH_DISTINCTION = 85; public Student() { super(); } public Student(String studentName, String studentID, double assignmentOneMarks, double assignmentTwoMarks, double projectMark) { super(); this.studentName = studentName; this.studentID = studentID; this.assignmentOneMarks = assignmentOneMarks; this.assignmentTwoMarks = assignmentTwoMarks; this.projectMark = projectMark; } public void setStudentName(String studentName) { this.studentName = studentName; } public void setStudentID(String studentID) { this.studentID = studentID; } public void setAssignmentOneMarks(double assignmentOneMarks) { this.assignmentOneMarks = assignmentOneMarks; } public void setAssignmentTwoMarks(double assignmentTwoMarks) { this.assignmentTwoMarks = assignmentTwoMarks; } public void setProjectMark(double projectMark) { this.projectMark = projectMark; } public String getStudentName() { return studentName; } public String getStudentID() { return studentID; } public double getAssignmentOneMarks() { return assignmentOneMarks; } public double getAssignmentTwoMarks() { return assignmentTwoMarks; } public double getProjectMark() { return projectMark; } public double calculateIndividualTotalMarks() { this.individualTotalMarks = this.assignmentOneMarks + this.assignmentTwoMarks + this.projectMark; return individualTotalMarks; } public String calculateGrade() { calculateIndividualTotalMarks(); if (this.individualTotalMarks >= HIGH_DISTINCTION) { this.grade = "HD"; } else if (this.individualTotalMarks >= DISTINCTION) { this.grade = "D"; } else if (this.individualTotalMarks >= CREDIT) { this.grade = "C"; } else if (this.individualTotalMarks >= PASS) { this.grade = "P"; } else { this.grade = "F"; } return this.grade; } }
GradingSystemMenu.java
// TODO -- create header comments //package gradingsystem; // you need to include this in each .java file if you want to package them together. For example packaging Student.java and GradingSystemMenu.java import java.util.Scanner; // TODO -- create the Student class (separate file: Student.java) public class GradingSystemMenu { final int ENTER_STUDENT_RECORD = 1; final int DISPLAY_GRADES = 2; final int DISPLAY_STATISTICS = 3; final int EXIT = 4; // TODO -- declare any further constants final int ASSIGNMENT_ONE_MARK = 20; final int ASSIGNMNET_TWO_MARK = 30; final int FINAL_PROJECT_MARK = 50; // TODO -- declare array of Student objects Student[] array = new Student[10]; // TODO -- declare variable for the current record entered (integer) int currentRecord = 0; Scanner inputMenuChoice = new Scanner(System.in); private int getMenuItem() { System.out.println(" Please select from the following"); System.out.println(ENTER_STUDENT_RECORD + ". Enter student's record"); System.out.println(DISPLAY_GRADES + ". Display all students grade"); System.out.println(DISPLAY_STATISTICS + ". Display Statistics"); System.out.println(EXIT + ". Exit the application"); System.out.print("Enter choice==> "); String choice = inputMenuChoice.nextLine(); return Integer.parseInt(choice); } private void processingGradeingSystem() { int choice = getMenuItem(); while (choice != EXIT) { switch (choice) { case ENTER_STUDENT_RECORD: enterStudentRcord(); break; case DISPLAY_GRADES: displayAllRecordsWithGrade(); break; case DISPLAY_STATISTICS: displayStatistics(); break; default: System.out.println("ERROR choice not recognised"); } choice = getMenuItem(); } } private void enterStudentRcord() { // TODO -- check if maximum record has been reached (this need to be done after // getting the other functionality working) if (currentRecord >= 10) { System.out.println("Errro - maximum record to be entered has been reached"); } else { // TODO -- read in the student's name and student's number (as a string) String studentName = getStudentNameFromUser(); while (studentName == null) { System.out.println("Error - student name must be not null"); studentName = getStudentNameFromUser(); } String studentID = getStudentNumberFromUser(); while (studentID == null) { System.out.println("Error - student name must be not null"); studentID = getStudentNumberFromUser(); } // TODO -- create validation loop (this need to be done after getting the other // functionality working) // TODO -- read assessments marks as double double assignmentOneMarks = getAssignmentOneMarksFromUser(); while (assignmentOneMarks > ASSIGNMENT_ONE_MARK) { System.out.println("Error - Assignment one marks must be 0 - 20"); assignmentOneMarks = getAssignmentOneMarksFromUser(); } double assignmentTwoMarks = getAssignmentTwoMarksFromUser(); while (assignmentTwoMarks > ASSIGNMNET_TWO_MARK) { System.out.println("Error - Assignment one marks must be 0 - 30"); assignmentTwoMarks = getAssignmentTwoMarksFromUser(); } double projectMark = getProjectMarksFromUser(); while (projectMark > FINAL_PROJECT_MARK) { System.out.println("Error - Assignment one marks must be 0 - 50"); projectMark = getProjectMarksFromUser(); } // TODO -- create validation loop (this need to be done after getting the other // functionality working) // TODO -- read in the number of students // TODO -- create validation loop (this need to be done after getting the other // functionality working) Student obj = new Student(studentName, studentID, assignmentOneMarks, assignmentTwoMarks, projectMark); // TODO -- add the data to the array (use the new keyword and the parameterised // constructor in student class) array[currentRecord] = obj; // TODO -- display the student name, student number, assessments marks displayHeading(); displayRecordDetails(currentRecord); // TODO -- increment the current booking variable for the next entry currentRecord++; } } private String getStudentNameFromUser() { Scanner inputStudentName = new Scanner(System.in); System.out.print(" Please enter the student name ==> "); return inputStudentName.nextLine(); } private String getStudentNumberFromUser() { Scanner inputStudentNumber = new Scanner(System.in); System.out.print(" Please enter the student number ==> "); return inputStudentNumber.nextLine(); } private double getAssignmentOneMarksFromUser() { Scanner inputAssignmentOneMarks = new Scanner(System.in); System.out.print(" Please enter your assignment one marks including decimal values ==> "); return inputAssignmentOneMarks.nextDouble(); } private double getAssignmentTwoMarksFromUser() { Scanner inputAssignmentTwoMarks = new Scanner(System.in); System.out.print(" Please enter your assignment two marks including decimal values ==> "); return inputAssignmentTwoMarks.nextDouble(); } private double getProjectMarksFromUser() { Scanner inputTakeHomeExamMarks = new Scanner(System.in); System.out.print(" Please enter your Examination marks including decimal values ==> "); return inputTakeHomeExamMarks.nextDouble(); } private void displayHeading() { System.out.printf("%-15s%-20s%-20s%-20s%-15s", "Student Name", "Student number", "Assignment One", "Assignment Two", "Examination"); } private void displayRecordDetails(int index) { // TODO -- check if there has been an record entered (this need to be done after // getting the other functionality working) // TODO -- display all of the entries entered so far (just display the current // entries not the whole array, use the current booking variable as the // termination condition) System.out.println(); System.out.printf("%-15s%-20s%-20s%-20s%-15s", array[index].getStudentName(), array[index].getStudentID(), array[index].getAssignmentOneMarks(), array[index].getAssignmentTwoMarks(), array[index].getProjectMark()); } private void displayAllRecordsWithGrade() { // TODO -- check if there has been an record entered (this need to be done after // getting the other functionality working) if (currentRecord == 0) { System.out.println("Error - You must enter at least one record"); } else { // TODO -- display all of the records entered so far with grade (just display // the current entries not the whole array, use the current record variable as // the termination condition) System.out.printf("%-15s%-20s%-20s%-20s%-15s%-20s", "Student Name", "Student number", "Assignment One", "Assignment Two", "Examination", "Grade"); for (int i = 0; i < currentRecord; i++) { System.out.println(); System.out.printf("%-15s%-20s%-20s%-20s%-15s%-20s", array[i].getStudentName(), array[i].getStudentID(), array[i].getAssignmentOneMarks(), array[i].getAssignmentTwoMarks(), array[i].getProjectMark(), array[i].calculateGrade()); } } } private void displayStatistics() { // TODO -- check if there has been an record entered (this need to be done after // getting the other functionality working) if (currentRecord == 0) { System.out.println("Error - You must enter at least one record"); } else { // TODO -- loop though the current entries in the array and calculate and // display the statistics System.out.println("||Statistics for COIT20245 grading system T2-2021||"); System.out.println(); double totalMark = 0.0; int minMarkIndex = 0; int maxMarkIndex = 0; for (int i = 0; i < currentRecord; i++) { double temp = array[i].calculateIndividualTotalMarks(); ; totalMark = totalMark + temp; if (temp < array[minMarkIndex].calculateIndividualTotalMarks()) { minMarkIndex = i; } if (temp > array[maxMarkIndex].calculateIndividualTotalMarks()) { maxMarkIndex = i; } } System.out.println(array[maxMarkIndex].getStudentName() + " has the maximum total marks of " + array[maxMarkIndex].calculateIndividualTotalMarks() + " with grade " + array[maxMarkIndex].calculateGrade()); System.out.println(array[minMarkIndex].getStudentName() + " has the minimum total marks of " + array[minMarkIndex].calculateIndividualTotalMarks() + " with grade " + array[minMarkIndex].calculateGrade()); System.out.println("Average total marks of entire T1-2021 cohort is " + totalMark / (double) currentRecord); } } public static void main(String[] args) { GradingSystemMenu app = new GradingSystemMenu(); // Entry welcome message goes here System.out.println("||Welcome to the Grade Management System(GMS) of COIT20245||"); app.processingGradeingSystem(); // Exit thank you message goes here System.out.println("Thank you for using the Grading Management System"); System.out.println("Program written by Biplob Ray with student number S12345678"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
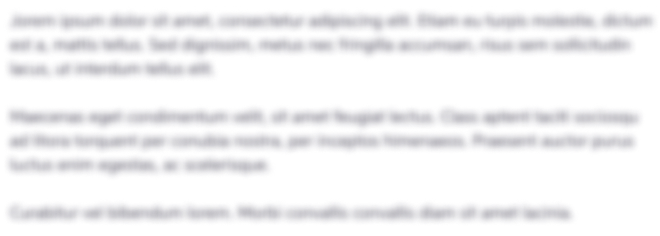
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started