Question
Assignment to be programmed in Java, and MUST use Arrays, NOT Arraylist The assignment is to create a standard deck of 52 cards, shuffle it,
Assignment to be programmed in Java, and MUST use Arrays, NOT Arraylist
The assignment is to create a standard deck of 52 cards, shuffle it, ask the user how many cards they want in their hand, and finally have the cards sorted in the hand by rank and value. The program must have 4 classes, the card class, deck class, hand class, and tester class.
The Card Class
Begin by creating a class to model a playing card. The Card class will have two private instance variables: (1) the face value (aka: rank) stored as ints 2..14 and (2) the suit (Spades, Hearts, Diamonds, Clubs).
Your Card class will have these methods:
- a proper constructor that creates a Card with a given rank and suit
- an accessor (get) method that returns the rank
- an accessor (get) method that returns the suit
- a toString method that returns a String representation of a card as shown in these examples: A, 10, 7, Q
Unicode characters: \u2660 (Spade) \u2663 (Club) \u2665 (Heart) \u2666 (Diamond)
The Deck Class
Create a class to model a standard 52-card deck. Your Deck class will have a private instance variable that is an Array of Card. (You may have additional instance vars although none are needed)
Your Deck class will have these methods:
- a proper constructor that creates a standard Deck of 52 cards
- a method that deals the top card. I.e. returns the Card object on top of the Deck
- a method that shuffles the deck. To get credit for this method you must use this algorithm:
For each card in the deck
remove it from the deck
insert it back at a random index.
The Hand Class
Create a class to model a hand of Cards. Your Hand class will have a private instance variable that is an Array-of-Card, and these methods:
- a proper constructor that creates an empty Hand
- a method to fill a Hand with however many cards the user request (ex 13 cards) Cards dealt from a Deck, with each one being inserted in its proper place in the Hand
- a toString method that returns a String representation of the Hand (Hint: call the Card class toString method for each Card in the Hand)
The Tester Class
The test class will have a main method that creates a Hand, calls the method that fills it, calls the toString method for the Hand, and prints the String returned. After each Hand is displayed, ask the user if she wants to see another. Also prompt the user to specify the size of each hand (assume correct input).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
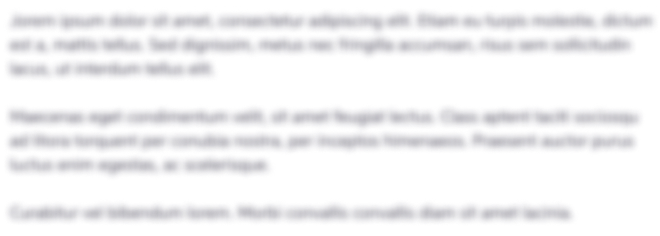
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started