Question
Assignment: Using C, Write a program that manages a linked list of records stored in structs. 1) You must use typedef. This is one of
Assignment:
Using C, Write a program that manages a linked list of records stored in structs.
1) You must use typedef. This is one of the main points of using linked list.
2) Structs cannot store the string in a fixed length array. This is not reconmended fo this project.
3) The strings has to be sorted out in alphabetical order. You could sort strings by ordering the *pointers* without copying the strings anywhere. This saves on storage and has improved performance as well.
A rough example should look like the program below:
Please be sure to use "typedef".
typedef struct node{
char *myString;
struct node* next;
}
Each "node" will manage a string, and a pointer to the next node in the list.
Once youve removed a node from the list, you need to return the memory (using free()), or youll have a leak.
Cook up a menu like so:
void main_menu()
{
char char_choice[3];
int int_choice = 0;
do
{
printf(" ");
printf("Main Menu: ");
printf("1. XXX ");
printf("2. YYY ");
printf("3. Etc... ");
scanf("%s", char_choice);
int_choice = atoi(char_choice);
switch (int_choice)
{
case 1:
function_XXX();
break;
case 2:
function_YYY();
break;
case 3:
function_Etc();
break;
default:printf("Wrong choice. Enter Again");
break;
}
}while(int_choice !=99);
}
The menu should allow you to add a string, modify a string, delete a string, list the strings, or quit.
This will give you enough practice with linked list.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
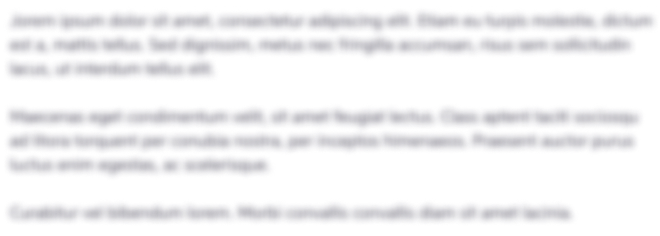
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started