Question
Assignment You will be writing a program to read album information from a file and output two report files: one containing a formatted table of
Assignment
You will be writing a program to read album information from a file and output two report files: one containing a formatted table of album counts and the second containing a histogram of album counts. Your program will prompt the user for an input filename containing artist and album data and two output filenames to save the results. No other output should be printed to the console window.
Console User Interaction
You must ask the user on the console for an input filename and output filenames. The following prompts must be used:
"Enter a filename containing album data: " "Enter a table output filename: " "Enter a histogram output filename: "
You should check that each file is able to be opened, and on failure prompt the user for a filename again.
Input File Format
Each entry in the input file will be contained within 2 lines in the following format:
- The artist name may contain spaces and consist of multiple words.
- The number of albums must be an integer value.
Table Output File Format
You will output a formatted two column table containing each artist name in the first column and their associated album counts in the second column with the following restrictions:
- The first line should contain column headers "Artist" and "Albums"
- The second line should use hyphen '-' characters to separate the header row from the table data rows. You may not use a loop and must use setfill and setw.
- The width of the first column should be equal to the length of the longest artist name with the text aligned to the left.
- The width of the second column should be 6 with album counts aligned to the right.
- Separate each column with the string " | " (space pipe space).
Histogram Output File Format
You will output a two column histogram with artist names on the left side and a series of asterisks corresponding to the corresponding number of albums to the right with the following restrictions:
- You do not need a header. The first line should contain data corresponding the first artist read from the input file.
- The width of the first column should be equal to one plus the length of the longest artist name with the text aligned to the right.
- The second column should be left aligned and contain a number of asterisks that matches the album count. You may not use a loop and must use setfill and setw.
- Separate each column with a single space.
Restrictions / Hints
- You may assume input files are properly formatted. However, you must check for valid input and output filenames.
- You may only read through the input file once. Store data from input files into vectors to read from when writing to output files.
- Use getline to read the input file.
- Use a stringstream to convert the album counts stored in a string to an integer value.
- You must use I/O manipulators to set the field justifications (right and/or left).
- You must use I/O manipulators to set the column widths (setw).
- If the input file is empty, you should not output anything to either specified output file.
- Do not add any file extensions to filenames for the user. Input and output files do not have to end with ".txt" or even have any file extension at all.
- You may not create any functions (if you know how).
Example
Valid input example:
Enter a filename containing album data: albums.txt Enter a table output filename: table.txt Enter a histogram output filename: histogram.txt
albums.txt file contains:
Iron Maiden 16 Opeth 13 Metallica 12 Megadeth 15 Amon Amarth 11 Slayer 12 Death 7 Black Sabbath 19 Dream Theater 14 Judas Priest 18 Nightwish 10 Children of Bodom 10 Blind Guardian 11 Dark Tranquillity 11 Agalloch 5
table.txt file is created and contains after running:
Artist | Albums -------------------------- Iron Maiden | 16 Opeth | 13 Metallica | 12 Megadeth | 15 Amon Amarth | 11 Slayer | 12 Death | 7 Black Sabbath | 19 Dream Theater | 14 Judas Priest | 18 Nightwish | 10 Children of Bodom | 10 Blind Guardian | 11 Dark Tranquillity | 11 Agalloch | 5
histogram.txt file is created and contains after running:
Iron Maiden **************** Opeth ************* Metallica ************ Megadeth *************** Amon Amarth *********** Slayer ************ Death ******* Black Sabbath ******************* Dream Theater ************** Judas Priest ****************** Nightwish ********** Children of Bodom ********** Blind Guardian *********** Dark Tranquillity *********** Agalloch *****
Missing/unable to open file example:
Enter a filename containing album data: nonexistent.txt Enter a filename containing album data: alsomissing Enter a filename containing album data: albums.txt Enter a table output filename: . Enter a table output filename: .. Enter a table output filename: my_table Enter a histogram output filename: / Enter a histogram output filename: my_graph.ext
*PLEASE WRITE IN C++ this is due in roughly 24 hours*
Step by Step Solution
There are 3 Steps involved in it
Step: 1
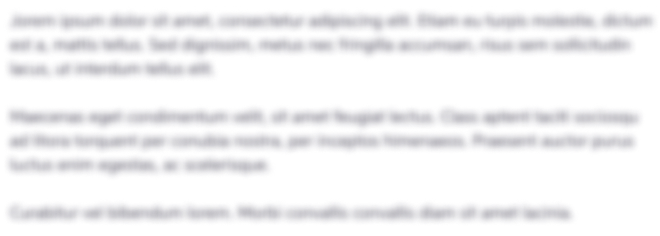
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started