Question
At the Welcome Window click Create New Project and call the project bank Download the starter files ( Account.java and BillPay.java ) from the course
At the Welcome Window click Create New Project and call the project bank
Download the starter files (Account.java and BillPay.java) from the course public folder public/6L
Account.java
/** * @author author * @version version */ public interface Account { /** deposit adds money to the account. * @param amount - the amount of the deposit, a nonnegative integer */ void deposit(int amount); /** withdraw deducts money from the account, if possible. * @param amount - the amount of the withdrawal, a nonnegative integer * @return true, if the the withdrawal was successful; * return false, otherwise. */ boolean withdraw(int amount); /** * * @return balance */ int getBalance(); }
BillPay.Java
/** * @author author * @version version */ public class BillPay { private Account account; private int payAmount; private int paymentsRemaining; /** * * @param account account to withdraw from * @param payAmount monthly payment amount * @param payments total number of payments */ public BillPay(Account account, int payAmount, int payments) { this.account = account; this.payAmount = payAmount; this.paymentsRemaining = payments; } /** * */ public void run() { System.out.println(String.format("Withdrawing $%d per month for %d payments...", this.payAmount, this.paymentsRemaining)); System.out.println(); for (int i = 0; i < this.paymentsRemaining; i++) { System.out.print(String.format("Attempting to withdraw payment %d: $%d", i + 1, this.payAmount)); if (this.account.withdraw(this.payAmount)) { System.out.println("...SUCCESS"); System.out.println("New balance is: $" + this.account.getBalance()); } else { System.out.println("...FAILED"); System.out.println("Insufficient funds"); break; } } } /** * * @param args args */ public static void main(String[] args) { CheckingAccount c = new CheckingAccount("1003899", "Ayesha Reynolds", 1528); BillPay bp = new BillPay(c, 1024, 4); System.out.println("Account Starting"); System.out.println("================"); System.out.println(c); System.out.println(); bp.run(); System.out.println(); System.out.println("Account Ending"); System.out.println("================"); System.out.println(c); } }
Do not modify the starter files
Account.java contains the interface Account that your checking account program will implement
BillPay.java can use for testing to see if you implemented your program correctly.
Save the downloaded files in your project into the folder called src
Create a new class called CheckingAccount in a file named CheckingAccount.java.
CheckingAccount Class Requirements
CheckingAccount implements the Account interface
Required instance variables are:
int for account balance
String for account id
String for account holder name
CheckingAccount has only one constructor with the following signature and assigns the parameter values to their corresponding instance variables
public CheckingAccount(String id, String name, int startingBalance) |
Implements the deposit method specified in the Account interface that increases the account balance by the amount of the passed in parameter
Implements the withdraw method specified in the Account interface that deducts the amount passed in the parameter from the balance if possible (meaning that the balance is greater than the funds to withdraw)
If successful, the method returns true
If not successful in withdrawing funds, the method returns false
Accessor methods for the appropriate instance variable
getBalance
getId
getName
Overrides the toString method in the Object class. Returns a string with the account id, name, and current balance with each value space separated. For example, if the account id is 1003899, the account holder's name is Ayesha Reynolds, and the current balance is 504, the string returned is:
1003899 Ayesha Reynolds $504 |
You can run the BillPay class against your implementation of CheckingAccount. BillPay is used in some of the Gradescope unit tests.
Submit CheckingAccout.java to Gradescope and confirm it passes all the tests
Step by Step Solution
There are 3 Steps involved in it
Step: 1
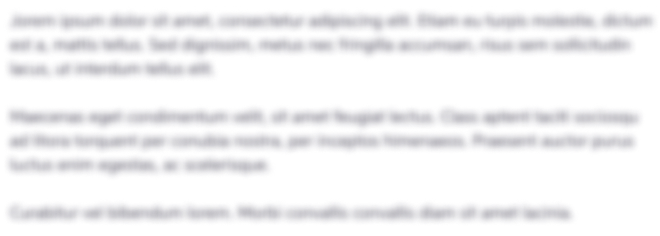
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started