Question
Attached is the code used for case Study Exercise 8.1 and an example output of the new code for this exercise (9.1) Exercise 9.1 Modify
Attached is the code used for case Study Exercise 8.1 and an example output of the new code for this exercise (9.1)
Exercise 9.1 Modify GUI and Graphics Case Study Exercise 8.1 to include a JLabel as a status bar that displays counts representing the number of each shape displayed. Class DrawPanel should declare a method that returns a String containing the status text. In main, first create the DrawPanel, then create the JLabel with the status text as an argument to the JLabels constructor. Attach the JLabel to the SOUTH region of the JFrame, as shown in Fig. 9.14.
In addition to what the problem asks for, do the following:
Create another class named MyShape. Make this a superclass. Its instance variables should be the x1, y1, x2, y2, and color values that are shared by all subclasses. MyShape should include the following methods:
A no-argument constructor.
A constructor that takes the arguments necessary to initialize its instance variables.
set and get methods for all instance variables.
A toString () method that generates and returns a String representation of a MyShape object.
Make MyLine, MyRectangle, and MyOval subclasses of MyShape. The fill flag of MyRectangle and MyOval will remain in those subclasses. Delete all other instance variables and methods possible that are in those subclasses (i.e. because they have been moved up to the superclass).
*****************************************************************************************
// File MyLine.java - create lines
package draw;
import java.awt.Color; import java.awt.Graphics;
public class MyLine
{ private int x1; //first x coordinate private int y1; //first y coordinate private int x2; //second x coordinate private int y2; //second y coordinate private Color myColor; //color of shape
private boolean flag; //determine if shape is a filled shape
//constructor with arguments for initializing instance variables public MyLine() { this.x1 = 0; //set first x coordinate to 0 this.y1 = 0; //set first y coordinate to 0 this.x2 = 0; //set second x coordinate to 0 this.y2 = 0; //set second y coordinate to 0
myColor = Color.BLACK; //set the color of the shape
flag = false; }
//constructor with input values public MyLine(int x1, int y1, int x2, int y2, Color color, boolean flag) { setX1(x1); //set first x coordinate setY1(y1); //set first y coordinate setX2(x2); //set second x coordinate setY2(y2); //set second y coordinate setMyColor(color);//set the color of the shape setFilled(flag); //set the filling of the shape }
// Draw the rectangle in the specified color public void draw(Graphics g) { if (getFilled()) g.setColor(myColor); else g.setColor(Color.BLACK); g.drawLine(getUpperLeftX(), getUpperLeftY(), getWidth(), getHeight()); }
//gets the upper left of X coordinate public int getUpperLeftX() { return Math.min(this.x1, this.x2); }
//gets the upper left of Y coordinate public int getUpperLeftY() { return Math.min(this.y1, this.y2); }
//gets the width public int getWidth() { return Math.abs(this.x1 - this.x2); }
//gets the height public int getHeight() { return Math.abs(this.y1 - this.y2); }
//setter methods for the instance variables public void setX1(int x1) { if (x1 >= 0) this.x1 = x1; else this.x1 = 0; }
public void setY1(int y1) { if (y1 >= 0) this.y1 = y1; else this.y1 = 0; }
public void setX2(int x2) { if (x2 >= 0) this.x2 = x2; else this.x2 = 0; }
public void setY2(int y2) { if (y2 >= 0) this.y2 = y2; else this.y2 = 0; }
public void setMyColor(Color myColor) { this.myColor = myColor; }
public void setFilled(boolean flag) { this.flag = flag; }
public boolean getFilled() { return this.flag; } } //end class MyLine
***************************************
// File MyRectangle.java - create rectangles
package draw;
import java.awt.Color; import java.awt.Graphics;
public class MyRectangle { private int x1; //first x coordinate private int y1; //first y coordinate private int x2; //second x coordinate private int y2; //second y coordinate private Color myColor; //color of shape
private boolean flag; //determine if this shape is a filled shape
//constructor without input values public MyRectangle() { this.x1 = 0; //set first x coordinate to 0 this.y1 = 0; //set first y coordinate to 0 this.x2 = 0; //set second x coordinate to 0 this.y2 = 0; //set second y coordinate to 0 myColor = Color.BLACK; //set the color of the shape
flag = false; }
// constructor with input values public MyRectangle(int x1, int y1, int x2, int y2, Color color, boolean flag) { setX1(x1); //set first x coordinate setY1(y1); //set first y coordinate setX2(x2); //set second x coordinate setY2(y2); //set second y coordinate setMyColor(color);//set the color of the shape setFilled(flag); //set the filling of the shape }
// Draw the rectangle in the specified color public void draw(Graphics g) { g.setColor(myColor); if (getFilled()) { g.fillRect(getUpperLeftX(), getUpperLeftY(), getWidth(), getHeight()); } else { g.drawRect(getUpperLeftX(), getUpperLeftY(), getWidth(), getHeight()); } }
//gets the upper left of X coordinate public int getUpperLeftX() { return Math.min(this.x1, this.x2); }
//gets the upper left of Y coordinate public int getUpperLeftY() { return Math.min(this.y1, this.y2); }
//gets the width public int getWidth() { return Math.abs(this.x1 - this.x2); }
//gets the height public int getHeight() { return Math.abs(this.y1 - this.y2); }
// the setter methods for the instance variables public void setX1(int x1) { if (x1 >= 0) this.x1 = x1; else this.x1 = 0; }
public void setY1(int y1) { if (y1 >= 0) this.y1 = y1; else this.y1 = 0; }
public void setX2(int x2) { if (x2 >= 0) this.x2 = x2; else this.x2 = 0; }
public void setY2(int y2) { if (y2 >= 0) this.y2 = y2; else this.y2 = 0; }
public void setMyColor(Color myColor) { this.myColor = myColor; }
public void setFilled(boolean flag) { this.flag = flag; }
public boolean getFilled() { return this.flag; } } //end class MyRectangle
*******************************************
// File MyOval.java - create ovals // Author: Arnel Cunanan
package draw;
import java.awt.Color; import java.awt.Graphics;
public class MyOval
{ private int x1; //set first x coordinate private int y1; //set first y coordinate private int x2; //set second x coordinate private int y2; //set second y coordinate private Color myColor; // color of the shape
private boolean flag; //determine if this shape is a filled shape
// constructor without input values public MyOval() { this.x1 = 0; //set first x coordinate to 0 this.y1 = 0; //set first y coordinate to 0 this.x2 = 0; //set second x coordinate to 0 this.y2 = 0; //set second y coordinate to 0 myColor = Color.BLACK; //set the color of the shape
flag = false; }
// constructor with input values public MyOval(int x1, int y1, int x2, int y2, Color color, boolean flag) { setX1(x1); //set first x coordinate setY1(y1); //set first y coordinate setX2(x2); //set second x coordinate setY2(y2); //set second y coordinate setMyColor(color);//set the color of the shape setFilled(flag); //set the filling of the shape }
//Draw the line in the specified color public void draw(Graphics g) { g.setColor(myColor); if (getFilled()) { g.fillOval(getUpperLeftX(), getUpperLeftY(), getWidth(), getHeight()); } else { g.drawRect(getUpperLeftX(), getUpperLeftY(), getWidth(), getHeight()); } }
//gets the upper left of X coordinate public int getUpperLeftX() { return Math.min(this.x1, this.x2); }
//gets the upper left of Y coordinate public int getUpperLeftY() { return Math.min(this.y1, this.y2); } //gets the width public int getWidth() { return Math.abs(this.x1 - this.x2); } //gets the height public int getHeight() { return Math.abs(this.y1 - this.y2); }
//the setter methods for the instance variables public void setX1(int x1) { if (x1 >= 0) this.x1 = x1; else this.x1 = 0; } public void setY1(int y1) { if (y1 >= 0) this.y1 = y1; else this.y1 = 0; } public void setX2(int x2) { if (x2 >= 0) this.x2 = x2; else this.x2 = 0; } public void setY2(int y2) { if (y2 >= 0) this.y2 = y2; else this.y2 = 0; } public void setMyColor(Color myColor) { this.myColor = myColor; } public void setFilled(boolean flag) { this.flag = flag; } public boolean getFilled() { return this.flag; } } //end class MyOval
**************************************************
// File DrawPanel.java - handles creating the shapes
package draw;
import java.awt.Color; import java.awt.Graphics; import java.util.Random; import javax.swing.JPanel;
//DrawPanel Panel that draws all the three shapes public class DrawPanel extends JPanel { private Random randomNumbers = new Random(); private MyLine lines[]; //array of lines private MyRectangle rectangles[]; //array of rectangles private MyOval ovals[]; //array of ovals
//constructor, creates a panel with random shapes public DrawPanel() { setBackground(Color.WHITE);
lines = new MyLine[randomNumbers.nextInt(5)]; rectangles = new MyRectangle[randomNumbers.nextInt(5)]; ovals = new MyOval[randomNumbers.nextInt(5)];
//create lines lines for (int count = 0; count
//generate a random color Color color = new Color(randomNumbers.nextInt(256), randomNumbers.nextInt(256), randomNumbers.nextInt(256));
boolean flag = randomNumbers.nextBoolean();
//add the line to the list of lines to be displayed lines[count] = new MyLine(x1, y1, x2, y2, color, flag); } //end create lines
//create rectangles for (int count = 0; count
//generate a random color Color color = new Color(randomNumbers.nextInt(256), randomNumbers.nextInt(256), randomNumbers.nextInt(256));
boolean flag = randomNumbers.nextBoolean();
//add the rectangle to the list of rectangles to be displayed rectangles[count] = new MyRectangle(x1, y1, x2, y2, color, flag); } //end create rectangles
//create ovals for (int count = 0; count
//generate random coordinates int x1 = randomNumbers.nextInt(300); int y1 = randomNumbers.nextInt(300); int x2 = randomNumbers.nextInt(300); int y2 = randomNumbers.nextInt(300); // generate a random color Color color = new Color(randomNumbers.nextInt(256), randomNumbers.nextInt(256), randomNumbers.nextInt(256));
boolean flag = randomNumbers.nextBoolean(); // add the oval to the list of ovals to be displayed ovals[count] = new MyOval(x1, y1, x2, y2, color, flag); } // end create ovals }
// for each shape array, draw the individual shapes public void paintComponent(Graphics g) { super.paintComponent(g); //draw the lines for (MyLine line : lines) line.draw(g);
// draw the rectangles for (MyRectangle rectangle : rectangles) rectangle.draw(g);
// draw the ovals for (MyOval oval : ovals) oval.draw(g); } } //end class DrawPanel
*********************************************************
// File TestDraw.java - Test method
package draw;
import javax.swing.JFrame;
public class TestDraw
{
//Main Method
public static void main(String args[])
{
DrawPanel panel = new DrawPanel();
JFrame app = new JFrame();
app.setTitle("Draw Random Shapes");
app.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
app.add(panel);
app.setSize(300, 300);
app.setVisible(true);
}
} //end class TestDraw
Step by Step Solution
There are 3 Steps involved in it
Step: 1
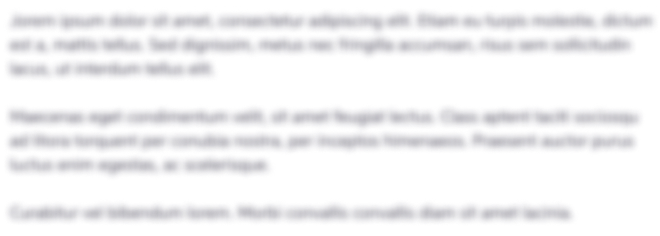
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started