Question
Attached the Screenshot of GroceryBill.java, Item.java, and Employee.java. Please also create a Main Program to test the code. Suppose a class GroceryBill keeps track of
Attached the Screenshot of GroceryBill.java, Item.java, and Employee.java.
Please also create a Main Program to test the code.
Suppose a class GroceryBill keeps track of a list of items being purchased at a market:
public GroceryBill(Employee clerk) | Constructs a grocery bill object for the given clerk |
public void add(Item i) | Adds the given item to this bill |
public double getTotal() | Returns the total cost of these items |
public void printReceipt() | Prints a list of items |
Grocery bills interact with Item objects, each of which has the public methods that follow. A candy bar item might cost 1.35 with a discount of 0.25 for preferred customers, meaning that preferred customers get it for 1.10. (Some items will have no discount, 0.0.) Currently the preceding classes do not consider discounts. Every item in a bill is charged full price, and item discounts are ignored.
public double getPrice() | Returns the price for this item |
public double getDiscount() | Returns the discount for this item |
Define a class DiscountBill that extends GroceryBill to compute discounts for preferred customers. Its constructor accepts a parameter for whether the customer should get the discount. Your class should also adjust the total reported for preferred customers. For example, if the total would have been $80 but a preferred customer is getting $20 in discounts, then getTotal should report the total as $60 for that customer. Also keep track of the number of items on which a customer is getting a nonzero discount and the sum of these discounts, both as a total amount and as a percentage of the original bill. Include the extra methods that follow, which allow a client to ask about the discount. Return 0.0 if the customer is not a preferred customer or if no items were discounted.
public DiscountBill(Employee clerk, boolean preferred) | Constructs bill for given clerk |
public int getDiscountCount() | Returns the number of items that were discounted, if any |
public double getDiscountAmount() | Returns the total discount for this list of items, if any |
public double getDiscountPercent() | Returns the percent of the total discount as a percent of what the total would have been otherwise |
*****************
CLASSES:
*****************
public class Item { private String name; private double price; private double discount;
public Item(String name, double price, double discount) { // Constructor this.name = name; this.price = price; this.discount = discount; } // Accessor methods public String getName() { return name; } public double getPrice() { return price; } public double getDiscount() { return discount; } public String toString() { // toString method for printing the receipt return name + ": $" + price + " (-$" + discount + ")"; }
}
***************************************************************
public class Employee { String name; public Employee(String name) { this.name = name; } public String getName() { return name; } }
*******************************************************
public class GroceryBill { private double total_price; private int item_count; private Item receipt[]; private Employee clerk;
public GroceryBill(Employee clerk) { // Constructor with Employee parameter total_price = 0; item_count = 0; receipt = new Item[25]; this.clerk = clerk; } public void add(Item i) { // Mutator method for adding an item total_price += i.getPrice(); receipt[item_count] = i; item_count += 1; } // Accessor methods public double getTotal() { return total_price; } public int getItemCount() { return item_count; } public String getCashierName() { return clerk.getName(); } public void printReceipt() { // Printing the bill System.out.println(receiptToString()); System.out.println("Cashier Name: " + clerk.getName()); System.out.println("Total: $" + this.getTotal()); } public String receiptToString() { String receiptText = "Items: "; for(int i = 0; i < item_count; i++) { receiptText += receipt[i]; receiptText += " "; } return receiptText; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
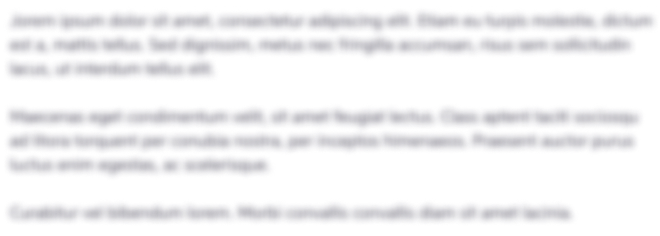
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started