Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/ * * * @author Y Nhi Tran * CIS 3 6 B , Practice Program 6 . 2 : Insertion sort * / import
@author Y Nhi Tran CIS B Practice Program : Insertion sort import java.util.Scanner; public class LabProgram private static int comparisons ; private static int swaps ; Read and return an array of integers. The first integer read is number of integers that follow. private static int readNums Scanner scnr new ScannerSystemin; int size scnrnextInt; Read array size int numbers new intsize; Create array for int i ; i size; i Read the numbers numbersi scnrnextInt; return numbers; Print the numbers in the array, separated by spaces No space or newline before the first number or after the last. private static void printNumsint nums for int i ; i nums.length; i System.out.printnumsi; if i nums.length System.out.print; System.out.println; Exchange numsj and numsk private static void swapint nums, int j int k int temp numsj; numsj numsk; numsk temp; Sort numbers TODO: Count comparisons and swaps. Output the array at the end of each iteration. public static void insertionSortint numbers int i; int j; for i ; i numbers.length; i j i; Count comparisons outer iteration comparisons; Insert numbersi into sorted part, stopping once numbersi is in correct position while j && numbersj numbersj Swap numbersj and numbersj swapnumbers j j ; j; Count swaps swaps ; Count comparisons, each swap is time comparisons ; Output the array at the end of each iteration printNumsnumbers; public static void mainString args Step : Read numbers into an array int numbers readNums; Step : Output the numbers array printNumsnumbers; System.out.println; Step : Sort the numbers array insertionSortnumbers; System.out.println; step TODO: Output the number of comparisons and swaps performed System.out.printlncomparisons: comparisons; System.out.printlnswaps: swaps; Please help me fix my code to have a correct comparisions output. Example below:
@author Y Nhi Tran
CIS B Practice Program : Insertion sort
import java.util.Scanner;
public class LabProgram
private static int comparisons ;
private static int swaps ;
Read and return an array of integers.
The first integer read is number of integers that follow.
private static int readNums
Scanner scnr new ScannerSystemin;
int size scnrnextInt; Read array size
int numbers new intsize; Create array
for int i ; i size; i Read the numbers
numbersi scnrnextInt;
return numbers;
Print the numbers in the array, separated by spaces
No space or newline before the first number or after the last.
private static void printNumsint nums
for int i ; i nums.length; i
System.out.printnumsi;
if i nums.length
System.out.print;
System.out.println;
Exchange numsj and numsk
private static void swapint nums, int j int k
int temp numsj;
numsj numsk;
numsk temp;
Sort numbers
TODO: Count comparisons and swaps. Output the array at the end of each iteration.
public static void insertionSortint numbers
int i;
int j;
for i ; i numbers.length; i
j i;
Count comparisons outer iteration
comparisons;
Insert numbersi into sorted part,
stopping once numbersi is in correct position
while j && numbersj numbersj
Swap numbersj and numbersj
swapnumbers j j ;
j;
Count swaps
swaps ;
Count comparisons, each swap is time
comparisons ;
Output the array at the end of each iteration
printNumsnumbers;
public static void mainString args
Step : Read numbers into an array
int numbers readNums;
Step : Output the numbers array
printNumsnumbers;
System.out.println;
Step : Sort the numbers array
insertionSortnumbers;
System.out.println;
step
TODO: Output the number of comparisons and swaps performed
System.out.printlncomparisons: comparisons;
System.out.printlnswaps: swaps;
Please help me fix my code to have a correct comparisions output.
Example below:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
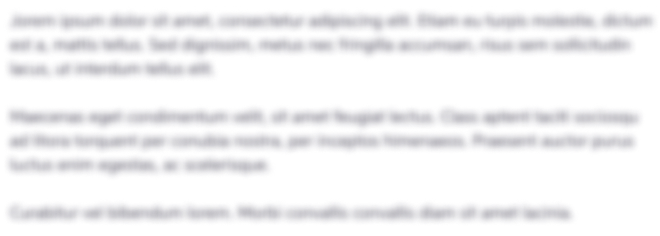
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started