Question
b) In class MyOrderedLinkedList, i. override method add(E e) (as required by class MyList) so as to insert the element into a new node in
b) In class MyOrderedLinkedList,
i. override method add(E e) (as required by class MyList) so as to insert the element
into a new node in an ordered list.
ii. rewrite methods addFirst(E e), addLast(E e) and add(int index, E e) so as to insert the element into a new node in an ordered list.
iii. implement methods contains(E e), get(int index), indexOf(E e), lastIndexOf(E e) and set(int index, E e). You may, of course, use your solutions from problem 1 above for these methods, making any changes necessary to the code that improves efficiency for an ordered list.
c) Add code to class TestMyOrderedLinkedList to demonstrate your implementations and that the list managed is an ordered list.
#MyList
public interface MyList
/** Add a new element at the end of this list*/ public void add(E e);
/**Add a new element at the specified index in this list*/ public void add(int index, E e);
/**Clear the list*/ public void clear();
/**Return true if this list contains the element*/ public boolean contains(E e);
/**Return the element from this list at the specified index*/ public E get(int index);
/**Return the index of the first matching element in this list. Return -1 if * no match.*/ public int indexOf(E e);
/**Return true if this list contains no elements*/ public boolean isEmpty();
/**Return the index of the last matching element in this list Return -1 if * no match.*/ public int lastIndexOf(E e);
/**Remove the first occurrence of the element o from this list. Shift any * subsequent elements to the left. Return true if the element is removed.*/ public boolean remove(E e);
/**Remove the element at the specified position in this list Shift any * subsequent elements to the left. Return the element that was removed from * the list.*/ public E remove(int index);
/**Replace the element at the specified position in this list with the * specified element and returns the new set.*/ public Object set(int index, E e);
/** Return the number of elements in this list */ public int size(); }
#MyOrderedLinkedList
public class MyOrderedLinkedList
private Node
/**Create a list from an array of objects*/ public MyOrderedLinkedList(E[] objects) { super(objects); }
// ************************************************************** // ************************************************************** /** Add a new element to the list in order.*/ // ************************************************************** // ************************************************************** public void add(E e) { } /**Return the head element in the list*/ public E getFirst() { if (size == 0) { return null; } else { return head.element; } }
/**Return the last element in the list*/ public E getLast() { if (size == 0) { return null; } else { return tail.element; } }
// ************************************************************** // ************************************************************** /**Must be rewritten to add a new element to the list in order.*/ // ************************************************************** // ************************************************************** public void addFirst(E e) { Node
if (tail == null) // the new node is the only node in list { tail = head; } }
// ************************************************************** // ************************************************************** /**Must be rewritten to add a new element to the list in order.*/ // ************************************************************** // ************************************************************** public void addLast(E e) { Node
if (tail == null) { head = tail = newNode; // The new node is the only node in list } else { tail.next = newNode; // Link the new with the last node tail = tail.next; // tail now points to the last node }
size++; // Increase size }
@Override // ************************************************************** // ************************************************************** /**Must be rewritten to add a new element to the list in order.*/ // ************************************************************** // ************************************************************** public void add(int index, E e) { if (index == 0) { addFirst(e); } else if (index >= size) { addLast(e); } else { Node
// ************************************************************** // ************************************************************** /**Must be rewritten to do nothing and return null.*/ // ************************************************************** // ************************************************************** public E removeFirst() { if (size == 0) { return null; } else { Node
// ************************************************************** // ************************************************************** /**Must be rewritten to do nothing and return null.*/ // ************************************************************** // ************************************************************** public E removeLast() { if (size == 0) { return null; } else if (size == 1) { Node
for (int i = 0; i < size - 2; i++) { current = current.next; }
Node
@Override /**Remove the element at the specified position in this list. Return the * element that was removed from the list.*/ public E remove(int index) { if (index < 0 || index >= size) { return null; } else if (index == 0) { return removeFirst(); } else if (index == size - 1) { return removeLast(); } else { Node
for (int i = 1; i < index; i++) { previous = previous.next; }
Node
@Override /**Override toString() to return elements in the list*/ public String toString() { StringBuilder result = new StringBuilder("[");
Node
return result.toString(); }
@Override /**Clear the list*/ public void clear() { size = 0; head = tail = null; }
@Override /**Return true if this list contains the element e*/ public boolean contains(E e) { System.out.println("Implementation left as an exercise"); return true; }
@Override /**Return the element at the specified index*/ public E get(int index) { System.out.println("Implementation left as an exercise"); return null; }
@Override /**Return the index of the head matching element in this list. Return -1 if * no match.*/ public int indexOf(E e) { System.out.println("Implementation left as an exercise"); return 0; }
@Override /**Return the index of the last matching element in this list. Return -1 if * no match.*/ public int lastIndexOf(E e) { System.out.println("Implementation left as an exercise"); return 0; }
@Override /**Replace the element at the specified position in this list with the * specified element.*/ public E set(int index, E e) { System.out.println("Implementation left as an exercise"); return null; }
@Override /**Override iterator() defined in Iterable*/ public java.util.Iterator
private void checkIndex(int index) { if (index < 0 || index >= size) { throw new IndexOutOfBoundsException("Index: " + index + ", Size: " + size); } }
private class LinkedListIterator implements java.util.Iterator
private Node
@Override public boolean hasNext() { return (current != null); }
@Override public E next() { E e = current.element; current = current.next; return e; }
@Override public void remove() { System.out.println("Implementation left as an exercise"); } } // end of LinkedListIterator
private static class Node
E element; Node
public Node(E element) { this.element = element; } } // end of Node }
#TestMyLinkedList
public class TestMyOrderedLinkedList { public static void main(String[] args) { new TestMyOrderedLinkedList(); }
public TestMyOrderedLinkedList() { // Create a list for strings MyOrderedLinkedList
// Add elements to the list list.add("America"); // Add it to the list System.out.println("(1) " + list);
list.add(0, "Canada"); // Add it to the beginning of the list System.out.println("(2) " + list);
list.add("Russia"); // Add it to the end of the list System.out.println("(3) " + list);
list.addLast("France"); // Add it to the end of the list System.out.println("(4) " + list);
list.add(2, "Germany"); // Add it to the list at index 2 System.out.println("(5) " + list);
list.add(5, "Norway"); // Add it to the list at index 5 System.out.println("(6) " + list);
list.add(0, "Poland"); // Same as list.addFirst("Poland") System.out.println("(7) " + list);
// Remove elements from the list list.remove(0); // Same as list.remove("Australia") in this case System.out.println("(8) " + list);
list.remove(2); // Remove the element at index 2 System.out.println("(9) " + list);
list.remove(list.size() - 1); // Remove the last element System.out.print("(10) " + list + " (11) ");
for (String s : list) { System.out.print(s.toUpperCase() + " "); }
list.clear(); System.out.println(" After clearing the list, the list size is " + list.size()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
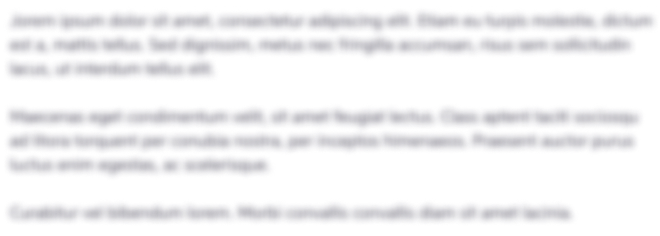
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started