Question
Background: C++ Language You find yourself wondering how your family can have such difficulty arranging a night out. Every Friday night, your family goes out
Background: C++ Language
You find yourself wondering how your family can have such difficulty arranging a night out. Every Friday night, your family goes out to eat. The discussion of where to eat, unfortunately, is often drawn out and sometimes becomes a little heated. Thinking about the NCAA Tournament, you get a brilliant idea of how to more efficiently make a selection.
Instead of fighting over several restaurants at once, you decide to write a program that eliminates one restaurant at a time by pitting two restaurants against each other until a single restaurant remains. Your family can now decide where to eat based on answering a series of yes/no questions. While this does not eliminate all of the arguing, it does simplify the decisions to be made.
Requirements:
Programming Language: C++
Part 1 - The Menu
Prompt the user to select one of several operations:
0 - Quit the program
1 - Display all restaurants
2 - Add a restaurant
3 - Remove a restaurant
4 - Cut the vector
5 - Shuffle the vector
6 - Begin the tournament
After any operation is complete (except for quitting), re-prompt the user to select another operation (return user to your menu)
You must require the user to input 0-6 to signify their menu choice
For part 1, only the quit option needs to work.
You may assume that you will be given an integer, but if an incorrect option is entered, print "INVALID SELECTION. Please try again." and re-prompt the user to select another operation (return user to your menu).
Part 2 - The Contenders
Add functionality to display, add, and remove restaurants. Each of these should be implemented using a function and you must use a vector to hold the list of restaurants. For this lab we will use a STRING CONTAINING THE NAME of the restaurant to represent the restaruant.
Display all restaurants
Display the restaurant names in their current order, on separate lines, prefaced with one tab ("\t").
Add a restaurant
Prompt the user for a restaurant name
If the restaurant name is not already in the vector, add it to the vector and say the restaurant name followed by " has been added."
If the restaurant name is already in the vector, do nothing to the vector but say "That restaurant is already on the list, you can not add it again."
Remove a restaurant
Prompt the user for a restaurant name
If the restaurant name is in the vector, remove it from the vector and say the restaurant name followed by " has been removed."
If the restaurant name is not in the vector, do nothing to the vector but say "That restaurant is not on the list, you can not remove it."
Both add and remove need to know (for different purposes) if the name given by the user is already in the list. This code should be in a "Find" function, not repeated in both places.
Part 3 - Mixing It Up
Add functionality for cut and shuffle. Each of these should be implemented using a function.
"Cut" the vector of restaurant names.
Prompt the user for a number
If the cut point is out of range say "The restaurants can not be cut there, there are only " current-number-of-restaurants " restaurants in the list."
In this program, "cut" is like the cut operation used in card games. Take the specified number of Restaurant names off of the top of the vector of restaurant names, and put them in the same order at the bottom. If the restaurant name list contains a, b, c, and d and the cut point is 3, then after the cut the list should be: d, a, b, and c.
Cut can be done by moving things around in the existing vector, but you might find that difficult. Instead, build a new vector as described and then return the newly assembled one from your cut function.
"Shuffle" restaurant names in the restaurant name vector.
In this program, "shuffle" is like the shuffle operation used in card games to mix up the cards. Split the "deck" in half, and rearrange the restaurants, alternating between the two halves, starting with the first restaurant in the second half. For instance, if the restaurant name vector contained the restaurant names: a, b, c, d, e, f, g and h, then after the shuffle, the vector would have the order e, a, f, b, g, c, h and d.
Implement this function from scratch. Do not use arcane #includes that have not been discussed in zybooks.
Do not allow the shuffle unless the number of restaurant names is a power of 2 (equal to 2^n for some value of n>=0). This must NOT be hard coded (i.e. do NOT use something like "if (n == 1 || n == 2 || n == 4 || n == 8...))". You must have an algorithm for solving this part. Say "The current tournament size (" current-list-length ") is not a power of two (2, 4, 8...)." followed by "A shuffle is not possible. Please add or remove resturants." if the length is not a power of two.
Like Cut, Shuffle can be done by moving things around in the existing vector, but you might find that difficult. Instead, build a new vector as described and then return the newly assembled one from your shuffle function.
Part 4 - The Battle
Now create at least one function and allow the user to run a tournament among the restaurants currently in the vector and report the winning restaurant name
Each match of the tournament involves displaying two restaurant names to the user, prompting her to choose her favorite, and retaining only the winning restaurant name for the next round. As noted above, you might find it easier to construct a new vector of candidates and replace the old one rather than moving things around in place.
You should expect the user to input either 1 or 2, signifying they prefer either the first or second restaurant, respectively.
For each round, take restaurant names in pairs and in order. Restaurant names should appear in exactly one match in a round.
If the user provides a response other than 1 or 2, re-prompt the user until valid information is entered.
Do not allow the tournament to begin unless the number of restaurant names is a power of 2, Use the same check you used for the shuffle function in Part 3.
Sample Output:
Welcome to the restaurant battle! Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 47 INVALID SELECTION. Please try again. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 2 What is the name of the restaurant you want to add? Super Taqueria Super Taqueria has been added. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 2 What is the name of the restaurant you want to add? Super Taqueria That restaurant is already on the list, you can not add it again. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 3 What is the name of the restaurant you want to remove? Noglu, Paris That restaurant is not on the list, you can not remove it. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 2 What is the name of the restaurant you want to add? Noglu, Paris Noglu, Paris has been added. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 2 What is the name of the restaurant you want to add? Cannon Center Cannon Center has been added. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 3 What is the name of the restaurant you want to remove? Cannon Center Cannon Center has been removed. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 2 What is the name of the restaurant you want to add? Costa Vida Costa Vida has been added. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 2 What is the name of the restaurant you want to add? Chuy's Chuy's has been added. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 1 Here are the current restaurants: "Super Taqueria" "Noglu, Paris" "Costa Vida" "Chuy's" Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 4 How many restaurants should be taken from the top and put on the bottom? 3 Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 1 Here are the current restaurants: "Chuy's" "Super Taqueria" "Noglu, Paris" "Costa Vida" Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 5 Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 1 Here are the current restaurants: "Noglu, Paris" "Chuy's" "Costa Vida" "Super Taqueria" Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 6 Round: 1 Type "1" if you prefer Noglu, Paris or type "2" if you prefer Chuy's Choice: 1 Type "1" if you prefer Costa Vida or type "2" if you prefer Super Taqueria Choice: 2 Round: 2 Type "1" if you prefer Noglu, Paris or type "2" if you prefer Super Taqueria Choice: 1 The winning restaurant is Noglu, Paris Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 3 What is the name of the restaurant you want to remove? Chuy's Chuy's has been removed. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 6 The current tournament size (3) is not a power of two (2, 4, 8...). A tournament is not possible. Please add or remove resturants. Please select one of the following options: 0 - Quit the program 1 - Display all restaurants 2 - Add a restaurant 3 - Remove a restaurant 4 - "Cut" the list of restaurants 5 - "Shuffle" the list of restaurants 6 - Begin the tournament Enter your selection now: 0 Goodbye!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
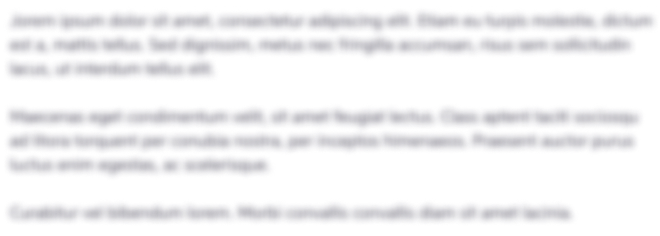
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started