Question
You are asked to write a c program that deals with the online purchase of TVs. The purchase order is handled first come first served
You are asked to write a c program that deals with the online purchase of TVs. The purchase order is handled first come first served basis. When a customer places an order to buy a TV, the program should search for that item in the database, and if it is found the purchase should be processed according to the instructions given below. TVs available in the shop are kept in a text file called TVs.txt. At the beginning of the program, the details of the TVs are read from TVs.txt and stored in a LinkedList. Then the list of the TVs ordered by customers is saved in a queue. Then the program should take the order from the beginning (head) of the queue and search for it in the LinkedList. If the item is found in the list delete it and put this TV in a stack to be able to retrieve the last TV sold. The main() function handles all interactions with the user and other functions:
• Calls a function named readers()which opens a text file TVs.txt (a sample text file is shown below) for reading and storing names of TVs from the file to a LinkedList in order of name (insertion should happen in alphabetical order). • It then repeatedly calls the menu () function to display user options, get the user selection returned by the menu() function, and uses a switch (or if ..else if) statement to process user requests by calling the appropriate function(s). Details of options in menu function:
(1) Display the current stock of TVs– here you display the contents of the LinkedList
(2) Add a new TV to stock – you need to insert a new TV to LinkedList
(3) Display next order information – displays the next TV in the order list (first node of the queue)
(4) Display all orders – displays all nodes of the queue
(5) Add order to queue – adds new order to the end of the queue
(6) Process the next order – Processes the first order in the queue. This function searches for this TV in the LinkedList and deletes (if found) from LinkedList and puts it into a stack, deletes it from the queue as well.
(7) Cancel the last order – It cancels the last processed order. It inserts the TV (top of the stack) back into LinkedList (TV is not added back into queue).
(8) Display info of last order – displays the information of the last processed order (top of the stack).
(9) Update TV file – updates the TVs.txt with the remaining TVs in the list (including the TVs added in option2).
(10) Quit program.
Step by Step Solution
3.39 Rating (149 Votes )
There are 3 Steps involved in it
Step: 1
include include include include typedef struct TV char name20 struct TV next TV typedef struct TV head TV tail Queue typedef struct TV top Stack Stack createStack Stack stack mallocsizeofStack stackto...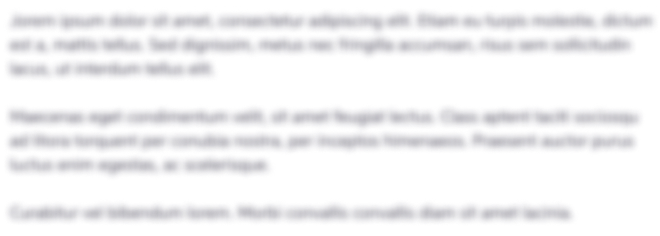
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started