Question
Been working on this for days after going over all lessons and cannot figure out Task 2 and 3. This is JavaScript. I have this
Been working on this for days after going over all lessons and cannot figure out Task 2 and 3. This is JavaScript.
I have this for task 1:
class Student {
constructor(name, email, community) {
this.name = name;
this.email = email;
this.community = community;
}
};
class Bootcamp {
constructor(name, level, students = []) {
this.name = name;
this.level = level;
this.students = students;
}
};
Objectives
- Demonstrate your knowledge of creating and using objects, classes, constructors, properties, and methods.
Instructions
- Read the instructions for this assignment before your end-of-week workshop and watch the video, but do not start on the assignment until you are at the workshop.
Setup
- Create two files: one namedReactWeek1Assignment.htmland one namedReactWeek1Assignment.js.
- InReactWeek1Assignment.html, enter this code:
Nucamp Community Coding Bootcamp
Nucamp Community Coding Bootcamp
Task 1
- InReactWeek1Assignment.js, you will create two classes:StudentandBootcamp.
- TheStudentclass should include:
- aconstructor;
- the constructor parameters: \"name\", \"email\", and \"community\";
- the constructor should assign the parameters' values to class properties of the same name using thethiskeyword.
- TheBootcampclass should include:
- a constructor;
- the constructor parameters: \"name\", \"level\", and a third parameter \"students\" which isan array initialized as empty(using default function parameters syntax);
- the constructor should assign the parameters values to class properties of the same name using thethiskeyword.
- Write the code for the Bootcamp class so that if it the \"students\" parameter is not passed in, it is by default initialized to be an empty array from within the constructor parameter list itself.
Task 2
In this task, you will add the methodregisterStudent()to theBootcampclass.
The methodregisterStudent():
- Should have a single parameter, which takes an object created from the Student class as its argument
- (so a name likestudentorstudentToRegisterwould be a sensible name to use as the parameter)
- Checks if any student with the same email address already exists in the Bootcamp'sstudentsarray.
- Use an Array method to verify if the student is already registered. There are multiple ways to make this verification, including a method you have learned this week. Research and find one way.
- Adds the passed-in students object to the end of the Bootcamp'sstudentsarrayonly if not already registered. There should be no duplicates in the students array.
- Using console.log, writes the outcome of the registration (e.g. \"Registering neo@matrix.com to the bootcamp Web Dev Fundamentals.\")
- At the end of the method,returnsthe current Bootcampstudentsarray.
Task 3
- Open the HTML page in your browser, then open the developer console on that page.
- in the developer console:
- Create a new bootcamp using the Bootcamp class. Follow the examples given in the video for this lesson, or create your own.
- Create one or more student object using the Student class. Follow the examples given in the video for this assignment, or create your own.
- Register the student object(s) to one of the bootcamp objects you created. Verify that registration succeeded.
- Verify that you cannot register the same student twice to a Bootcamp.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
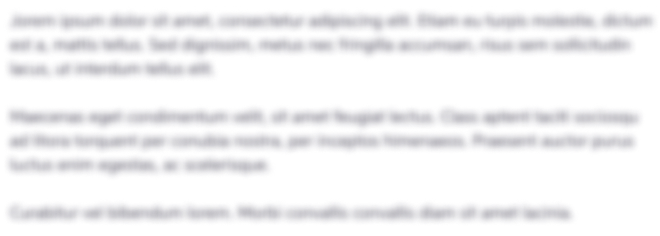
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started