Question
BELOW ARE THE FILES: LinkedList.cpp: #include #include #include LinkedList.h using namespace std; //--------------------------------------------------- //List Element Members //--------------------------------------------------- ListElement::ListElement(int _datum, ListElement * _next): datum (_datum), next
BELOW ARE THE FILES:
LinkedList.cpp:
#include
#include
#include "LinkedList.h"
using namespace std;
//---------------------------------------------------
//List Element Members
//---------------------------------------------------
ListElement::ListElement(int _datum, ListElement * _next):
datum (_datum), next (_next)
{}
int ListElement::getDatum () const
{
return datum;
}
ListElement const* ListElement::getNext () const
{
return next;
}
//---------------------------------------------------
//LinkedList Members
//---------------------------------------------------
LinkedList::LinkedList () :
head (0)
{}
void LinkedList::insertItem(int item)
{
ListElement *currPtr = head;
ListElement *prevPtr = NULL;
ListElement *newNodePtr; //points to a new node
while(currPtr != NULL && item > currPtr->datum)
{
prevPtr = currPtr;
currPtr = currPtr->next;
}
newNodePtr = new ListElement(item, currPtr);
if (prevPtr == NULL) // insert at the beginning
head=newNodePtr;
else
prevPtr->next = newNodePtr;
}
void LinkedList::makeList()
{
int InValue;
ListElement *currPtr;
ListElement *newNodePtr;
cout
cin >> InValue;
//Adding elements to end so that "next" will be NULL
newNodePtr=new ListElement(InValue, NULL);
head=newNodePtr; //assign head to the first Node
currPtr=head;
cin >> InValue;
while ( InValue != 999)
{
newNodePtr=new ListElement(InValue, NULL);
currPtr->next=newNodePtr; //link the new node to list
currPtr=newNodePtr; //move the currPtr so it is at end of list
cin >> InValue;
}
}
void LinkedList::appendItem (int item)
{
ListElement *currPtr;
ListElement *newNodePtr;
currPtr = head;
//Find the end of the list...
while (currPtr->next != NULL)
{
currPtr = currPtr->next;
}
//Now current-pointer points to the last node...
newNodePtr = new ListElement(item, NULL);
currPtr->next = newNodePtr;
}
void LinkedList::deleteItem (int item)
{
ListElement *delPtr;
ListElement *currPtr;
//Treat the first node as a special case... head needs to be updated
if (head->datum == item)
{
delPtr = head;
head = head->next;
}
else
{
currPtr = head;
//Look for the node to deleted
while (currPtr->next->datum != item)
{
currPtr = currPtr->next;
}
//Save its location
delPtr = currPtr->next;
//Route the list around the node to be deleted
currPtr->next = delPtr->next;
}
//Delete the node
delete delPtr;
}
void LinkedList::printList ()
{
ListElement *currPtr;
currPtr = head;
while (currPtr != NULL)
{
cout datum
currPtr = currPtr->next;
}
}
main.cpp:
#include
#include
#include"LinkedList.h"
using namespace std;
int readInt(string);
int main()
{
char choice;
int item;
LinkedList a;
cout
cout
cout
a.makeList();
choice='b';
while(choice != 'q')
{
cout
cout
cout
cout
cout
cout
cout
cout
cin>>choice;
switch(choice)
{
case 'i':
item=readInt("to insert:");
a.insertItem(item);
break;
case 'a':
item=readInt("to append to the end:");
a.appendItem(item);
break;
case 'd':
item=readInt("to delete:");
a.deleteItem(item);
break;
case 'p':
cout
a.printList();
break;
case 'q':
break;
default:
cout
break;
}
}
cout
return 0;
}
int readInt(string descr)
{
int item;
cout
cin >> item;
while (item
{
cout
cin >> item;
}
return item;
}
LinkedList.h:
// LinkedList.h
#ifndef LINKEDLIST_H
#define LINKEDLIST_H
#include
#include
using namespace std;
class LinkedList; // needed for friend line below
class ListElement
{
private:
int datum;
ListElement* next;
public:
ListElement (int, ListElement*);
int getDatum () const;
ListElement const* getNext () const;
friend class LinkedList; // gives LinkedList access to datum and next
};
class LinkedList
{
private:
ListElement* head;
public:
LinkedList ();
void insertItem (int);
void makeList ();
void appendItem (int);
void deleteItem (int);
void printList ();
};
#endif
BELOW IS THE SAMPLE OUTPUT:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
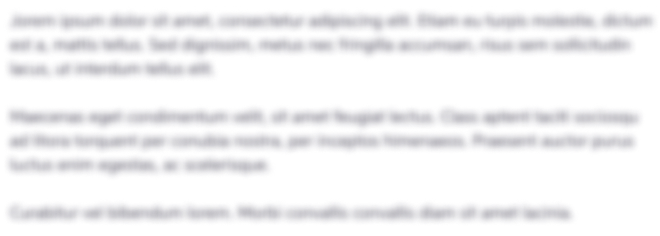
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started