Question
Below are the lexer, shank, and token files. There are errors in the lexer file so please fix those errors. The shank file is the
Below are the lexer, shank, and token files. There are errors in the lexer file so please fix those errors. The shank file is the main method file. There is a shank.txt as well. The main goal is to make sure the lexer must read all the tokens in the shank.txt file and print out those tokens. Make sure the entire lexer and state machine are still in the lexer file. It is missing an exception, so please add an exception as shown in the rubric below. The rubric below tells what must be added in the lexer. Show the entire written code and attached is rubric as well.
Lexer.java
package mypack;
import java.util.ArrayList; import java.util.List;
import mypack.Token.TokenType;
import java .util.HashMap;
public class Lexer { private static final int INTEGER_STATE = 1; private static final int DECIMAL_STATE = 2; private static final int IDENTIFIER_STATE = 3; private static final int ERROR_STATE = 4; private static final int SYMBOL_STATE = 5; private static final int STRING_STATE = 6; private static final int CHAR_STATE = 7; private static final int COMMENT_STATE = 8;
private static final char EOF = (char) -1;
private static String input; private static int index; private static char currentChar; private static int lineNumber = 1; private static int indentLevel = 0; private static int lastIndentLevel = 0;
private static HashMap
put("while", TokenType.WHILE); put("if", TokenType.IF); put("else", TokenType.ELSE); put("print", TokenType.PRINT);
}};
private static HashMap
}}; public Lexer(String input) { Lexer.input = input; index = 0; currentChar = input.charAt(index); }
private void nextChar() { index++; if (index >= input.length()) { currentChar = EOF; } else { currentChar = input.charAt(index); } }
private void skipWhiteSpace() { while (Character.isWhitespace(currentChar)) { nextChar(); } }
private int getIndentLevel() { int level = 0; int i = index; char c = input.charAt(i); while (c == ' ' || c == '\t') { if (c == '\t') { level += 1; } else if (c == ' ') { level += 1; } i++; if (i >= input.length()) { break; }
public List
while (currentChar != EOF) { switch (currentState()) { case INTEGER_STATE: integerState(tokens); break; case DECIMAL_STATE: decimalState(tokens); break; case IDENTIFIER_STATE: identifierState(tokens); break; case ERROR_STATE: errorState(tokens); break; case SYMBOL_STATE: symbolState(tokens); break; case STRING_STATE: stringState(tokens); break; case CHAR_STATE: charState(tokens); break; case COMMENT_STATE: commentState(tokens); break; default: break; } } return tokens; }
private void s(List
}
private static int currentState() { if (Character.isDigit(currentChar)) { return INTEGER_STATE; } else if (Character.isLetter(currentChar)) { return IDENTIFIER_STATE; } else if (currentChar == '.') { return DECIMAL_STATE; } else if (Character.isLetter(currentChar)) { return STRING_STATE; } else if (Character.isLetter(currentChar)) { return CHAR_STATE; }else if (Character.isLetter(currentChar)) { return COMMENT_STATE; }else if (Character.isDigit(currentChar)) { return SYMBOL_STATE; } else { return ERROR_STATE; } } private static void integerState(List
private static void symbolState(List
private static void stringState(List
Shank.java
package mypack;
import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.util.List;
public class Shank { public static void main(String[] args) { if (args.length != 1) { System.out.println("Error: Exactly one argument is required."); System.exit(0); } String filename = args[0];
try { List
Token.java
package mypack;
public class Token { public enum TokenType { WORD, NUMBER, SYMBOL }
public TokenType tokenType; private String value; public Token(TokenType type, String val) { this.tokenType = type; this.value = val; } public TokenType getTokenType() { return this.tokenType; } public String toString() { return this.tokenType + ": " + this.value; } }
Shank.txt
Fibonoacci (Iterative)
define add (num1,num2:integer var sum : integer) variable counter : integer Finonacci(N) int N = 10; while counter
GCD (Recursive)
define add (int a,int b : gcd) if b = 0 sum = a sum gcd(b, a % b)
GCD (Iterative)
define add (inta, intb : gcd) if a = 0 sum = b if b = 0 sum = a while counter a != b if a > b a = a - b; else b = b - a; sum = a; variables a,b : integer a = 60 b = 96 subtract a,b
Step by Step Solution
There are 3 Steps involved in it
Step: 1
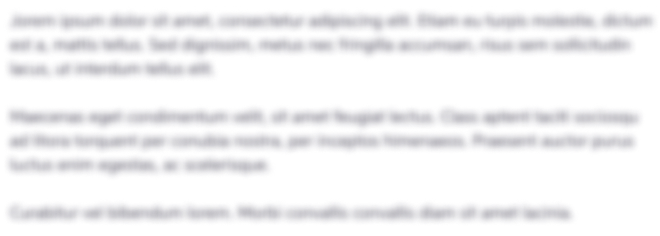
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started