Question
below is class structure for Appointment and VisitProcedure public class Appointment { //attributes private int month; private int day; private int year; private Pet pet;
below is class structure for Appointment and VisitProcedure
public class Appointment {
//attributes
private int month;
private int day;
private int year;
private Pet pet;
public Appointment(){
month = 0;
day = 0;
year = 0;
pet = new Pet();
}
/**
* getters and setters
*/
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public Pet getPet() {
return pet;
}
public void setPet(Pet pet) {
this.pet = pet;
}
public void Write(PrintStream ps){
ps.println(month);
ps.println(day);
ps.println(year);
ps.println(pet.getName());
ps.println(pet.getSpecies());
ps.println(pet.getGender());
}
public void Read(Scanner s){
month = s.nextInt();
day = s.nextInt();
year = s.nextInt();
s.nextLine(); //ignore newline before reading next line
pet.setName(s.nextLine());
pet.setSpecies(s.nextLine());
pet.setGender(s.nextLine());
}
/**
* returns details in JSON String format
*/
public String GetJSON(){
String s = "{ ";
s += "\"month\" : " + month + ", ";
s += "\"day\" : " + day + ", ";
s += "\"year\" : " + year + ", ";
s += "\"pet\" : " + pet.GetJSON() + " ";
s += "} ";
return s;
}
@Override
public String toString() {
return "Date: " + month + "/" + day + "/" + year + " " +
pet.toString();
}
}
---------------------------------------------------------------------------------------------------------------------------------------------------------------------
public class VisitProcedure {
//attributes
private Procedure procedure;
private double quantity;
private boolean isCoveredByInsurance;
private double pctCovered;
VisitProcedure() {
procedure = new Procedure();
quantity = 0;
isCoveredByInsurance = false;
pctCovered = 0;
}
/**
* parameterized constructor
*/
public Procedure getProcedure() {
return procedure;
}
/**
* getters and setters
*/
public void setProcedure(Procedure procedure) {
this.procedure = procedure;
}
public double getQuantity() {
return quantity;
}
public void setQuantity(double quantity) {
this.quantity = quantity;
}
public boolean isCoveredByInsurance() {
return isCoveredByInsurance;
}
public void setCoveredByInsurance(boolean isCoveredByInsurance) {
this.isCoveredByInsurance = isCoveredByInsurance;
}
public double getPctCovered() {
return pctCovered;
}
public void setPctCovered(double pctCovered) {
this.pctCovered = pctCovered;
}
double CalculateProcedureAmount() {
return procedure.getPrice() * quantity;
}
double CalculateProcedureAmountCovered() {
if(isCoveredByInsurance)
{
return CalculateProcedureAmount() * pctCovered;
}
return 0;
}
double CalculateProcedureAmountDue() {
// insurance by the method calculateProcedureAmountCovered()
return CalculateProcedureAmount() - CalculateProcedureAmountCovered();
}
void Write(PrintStream ps) {
ps.println(this.procedure.getName());
ps.println(this.procedure.getPrice());
ps.println(this.quantity);
ps.println(this.isCoveredByInsurance);
ps.println(this.pctCovered);
}
void Read(Scanner sc) {
this.procedure = new Procedure(sc.next(), sc.nextDouble());
this.quantity = sc.nextInt();
this.isCoveredByInsurance = sc.nextBoolean();
this.pctCovered = sc.nextDouble();
}
/**
* returns details in JSON String format
*/
String GetJSON() {
return "{"
+ "\"procedure\" : "
+ "{"
+ "\"name\" : " + this.procedure.getName()
+ "\"price\" : " + this.procedure.getPrice()
+ "},"
+ "\"quantity\" : " + this.quantity
+ "\"isCoveredByInsurance\" : " + this.isCoveredByInsurance
+ "\"pctCovered\" : " + this.pctCovered
+ "}";
}
public String toString() {
return "Name: " + this.procedure.getName() +
" Price: " + this.procedure.getPrice() +
" Quantity: " + this.quantity +
" Is Covered By Insurance: " + this.isCoveredByInsurance +
" Pct Covered: " + this.pctCovered;
}
} // end of class
Overview 1. Write a JAVA project that contains the definition of the Visit class Part 1 - Class Specifications Class- Visit Member Variables (all private Variable Veterina Data Tvpe Name of the veterinarian. Appointment data related to this visit. Array of VisitProcedure. String Appointment VisitProcedure Member Method Signatures and Descriptions (all public) Signature Default Constructor Description Default constructor. This method should initialize all member variables. The VisitProcedure array should be initialized to at least 4 elements. Hint: Make sure that new is called for ALL reference types. Be very careful initializing the array Only need get/set methods for name. Read the contents of all member variables from the given instance of Scanner. Assume that the Scanner is already open. See below for the file format. If the member is an array then you read in the number of elements in the array first then read in each element. For example, when reading in the VisitProcedure array data you should read in the number of elements first and use that number to allocate the array to the appropriate size. After Get/set methods void Read Scanner s) Overview 1. Write a JAVA project that contains the definition of the Visit class Part 1 - Class Specifications Class- Visit Member Variables (all private Variable Veterina Data Tvpe Name of the veterinarian. Appointment data related to this visit. Array of VisitProcedure. String Appointment VisitProcedure Member Method Signatures and Descriptions (all public) Signature Default Constructor Description Default constructor. This method should initialize all member variables. The VisitProcedure array should be initialized to at least 4 elements. Hint: Make sure that new is called for ALL reference types. Be very careful initializing the array Only need get/set methods for name. Read the contents of all member variables from the given instance of Scanner. Assume that the Scanner is already open. See below for the file format. If the member is an array then you read in the number of elements in the array first then read in each element. For example, when reading in the VisitProcedure array data you should read in the number of elements first and use that number to allocate the array to the appropriate size. After Get/set methods void Read Scanner s)Step by Step Solution
There are 3 Steps involved in it
Step: 1
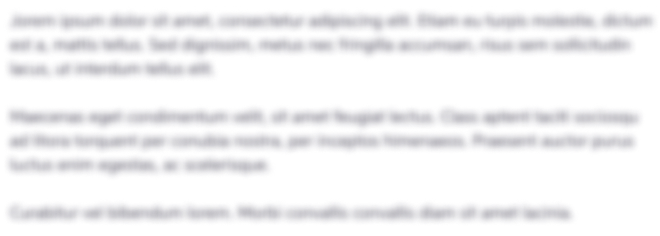
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started