Question
Below is my code in C++. This code is done using the singly linked list data structure. I need you to only add one more
Below is my code in C++. This code is done using the singly linked list data structure. I need you to only add one more thing in this code. Make a login system function which requires a username and password to enter the program. If the username and password is not correct, then the rest of the program should not start. You can only use singly linked list data structure.
#include
cout << "Node is null" << endl; return; } for (int i = 1; i <= loc; ++i) { temp = temp->next; if (i < loc) { head = head->next; } } head->next = temp->next; } Node* search(Node* head, string v) { if (head == NULL) { cout << "Node is empty returning null" << endl; return NULL; } int l = 1; while (head->next != NULL && head->data.name != v) { head = head->next; ++l; } cout << "Element found at location " << l << endl; return head; } void printLinkedList(Node* head) { if (head == NULL) { cout << "Head is null" << endl; return; } while (head->next != NULL) { cout << "Name: " << head->data.name << ", City: " << head->data.city << ", Immune Level: " << head->data.immune_level << ", Symptoms: " << head->data.symptoms << ", Severity: " << head->data.severity << ", Age: " << head->data.age << ", Admit No: " << head->data.admit_no << endl; head = head->next; } cout << "Name: " << head->data.name << ", City: " << head->data.city << ", Immune Level: " << head->data.immune_level << ", Symptoms: " << head->data.symptoms << ", Severity: " << head->data.severity << ", Age: " << head->data.age << ", Admit No: " << head->data.admit_no << endl; } void filewrite(Node * head) { ofstream fout; fout.open("patients.txt"); while (head->next != NULL) { fout << "Name: " << head->data.name << ", City: " << head->data.city << ", Immune Level: " << head->data.immune_level << ", Symptoms: " << head->data.symptoms << ", Severity: " << head->data.severity << ", Age: " << head->data.age << ", Admit No: " << head->data.admit_no << endl; head = head->next; } fout << "Name: " << head->data.name << ", City: " << head->data.city << ", Immune Level: " << head->data.immune_level << ", Symptoms: " << head->data.symptoms << ", Severity: " << head->data.severity << ", Age: " << head->data.age << ", Admit No: " << head->data.admit_no << endl; fout.close(); } Data inputPatients() { string name, city, symptoms, immune_level, severity; int admit_no, age; Data p; cout << "Enter Patient Name: "; cin.ignore(); getline(cin, name); cout << "Enter Patient City: "; getline(cin, city); cout << "Enter Patient Symptoms: "; getline(cin, symptoms); cout << "Enter Patient Immune Level: "; getline(cin, immune_level); cout << "Enter patient Severity: "; getline(cin, severity); cout << "Enter Patient Admit No.: "; cin >> admit_no; cout << "Enter Patient Age: "; cin >> age; p.name = name; p.city = city; p.symptoms = symptoms; p.immune_level = immune_level; p.severity = severity; p.admit_no = admit_no; p.age = age; cout << "Completed input operation" << endl; return p; } int main() { Node* head = NULL; Data patient; string nameToSearch; string oldName, newName; int op; cout << " 1-Add Patient 2-Delete Patient(Must have 3 patients in hospital to delete patients.) 3-Search by Name 4-Total Patients in Hospital 5-Print All Patients Record 6-Update Patient Name 7-Save Records in a file 8-Exit" << endl; while (cin >> op) { switch (op) { case 1: cout << "Enter Patient Details Below" << endl; patient = inputPatients(); head = append(head, patient); break; case 2: if (listLength(head) < 2) { cout << "Length is less then two. Terminating program" << endl; exit(1); } else { cout << "Enter location where you want to delete a patient"; int l; cin >> l; deletee(head, l - 1); } break; case 3: cout << "Enter name to search patient: "; cin.ignore(); getline(cin, nameToSearch); search(head, nameToSearch); break; case 4: cout << "You have " << listLength(head) << " Patients in your Hospital." << endl; break; case 5: printLinkedList(head); break; case 6: cin.ignore(); cout << "Enter old name "; getline(cin, oldName); cout << "Enter New Name "; getline(cin, newName); update(head, oldName, newName); break; case 8: exit(0); case 7: filewrite(head); break; default: cout << "Wrong option Selected" << endl; } cout << " 1-Add Patient 2-Delete Patient(Must have 3 patients in hospital to delete patients.) 3-Search by Name 4-Total Patients in Hospital 5-Print All Patients Record 6-Update Patient Name 7-Save Records in a file 8-Exit" << endl; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
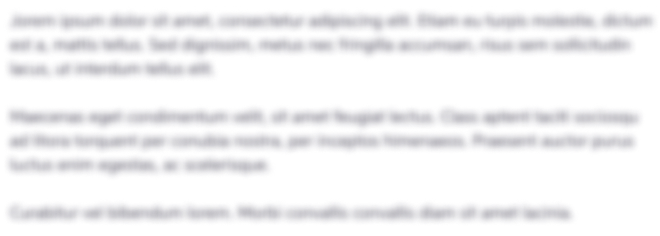
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started