Question
Below is the code for a program that uses a recursive function call to calculate a power. /*-------------------------------------------------------- * Name: Micah Harvey * Lab assignment:
Below is the code for a program that uses a recursive function call to calculate a power.
/*--------------------------------------------------------
* Name: Micah Harvey
* Lab assignment: 1
* Date: May 20, 2013
* Instructor: Micah Harvey
* Description: This program calculates the value of a power of a given base using recursive function call. *--------------------------------------------------------*/
#include "msp430.h"
#include "stdio.h"
//function prototypes
long int exponential(int, int);
int main(void)
{
WDTCTL = WDTPW + WDTHOLD; //stop the watchdog timer
int value = 12; //the base
int power = 4; //the exponent, must be positive and 1 or greater
long int answer = exponential(value, power); //find the power
printf("%d raised to the %d power is %ld", value, power, answer);
return 0;
}
//This function recursively calculates a power given a base and a power long int exponential(int value, int power)
{
int answer; //the solution
if (power > 1) //if the current call has power > 1 than call again
{
power--;
answer = value*exponential(value, power);
}
else //else the power = 1 and the answer is the base
{
answer = value;
}
return answer; //return the answer
}
PROBLEM: Write a C program to create a loop for printing a message 5 times. The counter value, used in the loop, should also be printed with the message. If the counter equals 2, you should print an extra message. The output should be like this:
Sample message, Counter = 1
Sample message, Counter = 2
Extra message, Counter = 2
Sample message, Counter = 3
Sample message, Counter = 4
Sample message, Counter = 5
You must first write the code in notepad and create a flowchart for the code. When you have completed that step, you must create an organized directory, subdirectory, workspace, and project for the code (your name/lab1). Once organized, you must properly compile and view the output. During demonstration, you should be able to inspect variables, set watchpoints, set and monitor breakpoints, monitor registers and memory, and show the output.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
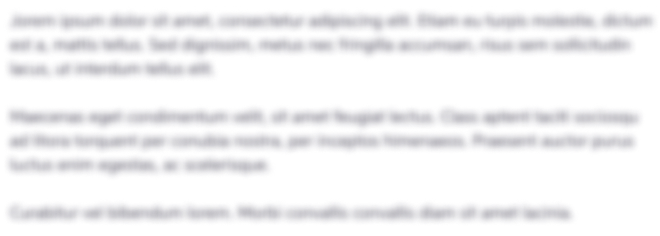
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started