Question
Below program oght to produce output that looks something like this: The array is: 4 3 6 9 3 9 5 4 1 9 This
Below program oght to produce output that looks something like this: The array is: 4 3 6 9 3 9 5 4 1 9 This array DOES contain 5. Sorted by Arrays.sort(): 1 3 3 4 4 5 6 9 9 9 Sorted by Sweep Sort: 1 3 3 4 4 5 6 9 9 9 Sorted by Selection Sort: 1 3 3 4 4 5 6 9 9 9 Sorted by Insertion Sort: 1 3 3 4 4 5 6 9 9 9 Unfortunately (or fortunately, for the purposes of this lab), each of the four subroutines in BuggySearchAndSort has a bug that can either produce incorrect results or an infinite loop. If you run the buggy program without modifying it, it will go into an infinite loop. Remember that to stop a running program in Eclipse, you can click the Stop button that looks like a small red square in the part of the window where the Console view appears: (If this button is gray instead of red, it means that no program is currently running in the visible console. However, there might be other consoles that are still running programs; to avoid having a bunch of programs, all running in infinite loops at the same time, you should make sure that a program has been terminated before starting another program run.) Your job in this lab is to use the Eclipse debugger to find each of the four bugs. In the written lab report that you will turn in, you should describe each bug, say how you fixed it, and explain briefly how you used the Eclipse debugger to find the bug. (Note: It's possible that you could find some or all of the bugs just by inspecting the code. However, the main purpose of this part of the lab is to get some experience with the debugger, so I would like you to do that rather than simply eyeball the code!)
import java.util.Arrays;
/**
* This class looks like it's meant to provide a few public static methods
* for searching and sorting arrays. It also has a main method that tests
* the searching and sorting methods.
*
* TODO: The search and sort methods in this class contain bugs that can
* cause incorrect output or infinite loops. Use the Eclipse debugger to
* find the bugs and fix them
*/
public class BuggySearchAndSort {
public static void main(String[] args) {
int[] A = new int[10]; // Create an array and fill it with small random ints.
for (int i = 0; i
A[i] = 1 + (int)(10 * Math.random());
int[] B = A.clone(); // Make copies of the array.
int[] C = A.clone();
int[] D = A.clone();
System.out.print("The array is:");
printArray(A);
if (contains(A,5))
System.out.println("This array DOES contain 5.");
else
System.out.println("This array DOES NOT contain 5.");
Arrays.sort(A); // Sort using Java's built-in sort method!
System.out.print("Sorted by Arrays.sort(): ");
printArray(A); // (Prints a correctly sorted array.)
bubbleSort(B);
System.out.print("Sorted by Bubble Sort: ");
printArray(B);
selectionSort(C);
System.out.print("Sorted by Selection Sort: ");
printArray(C);
insertionSort(D);
System.out.print("Sorted by Insertion Sort: ");
printArray(D);
}
/**
* Tests whether an array of ints contains a given value.
* @param array a non-null array that is to be searched
* @param val the value for which the method will search
* @return true if val is one of the items in the array, false if not
*/
public static boolean contains(int[] array, int val) {
for (int i = 0; i
if (array[i] == val)
return true;
else
return false;
}
return false;
}
/**
* Sorts an array into non-decreasing order. This inefficient sorting
* method simply sweeps through the array, exchanging neighboring elements
* that are out of order. The number of times that it does this is equal
* to the length of the array.
*/
public static void bubbleSort(int[] array) {
for (int i = 0; i
for (int j = 0; j
if (array[j] > array[j+1]) { // swap elements j and j+1
int temp = array[j];
array[j] = array[j+1];
array[j+1] = temp;
}
}
}
}
/**
* Sorts an array into non-decreasing order. This method uses a selection
* sort algorithm, in which the largest item is found and placed at the end of
* the list, then the second-largest in the next to last place, and so on.
*/
public static void selectionSort(int[] array) {
for (int top = array.length - 1; top > 0; top--) {
int positionOfMax = 0;
for (int i = 1; i =>
if (array[1] > array[positionOfMax])
positionOfMax = i;
}
int temp = array[top]; // swap top item with biggest item
array[top] = array[positionOfMax];
array[positionOfMax] = temp;
}
}
/**
* Sorts an array into non-decreasing order. This method uses a standard
* insertion sort algorithm, in which each element in turn is moved downwards
* past any elements that are greater than it.
*/
public static void insertionSort(int[] array) {
for (int top = 1; top
int temp = array[top]; // copy item that into temp variable
int pos = top - 1;
while (pos > 0 && array[pos] > temp) {
// move items that are bigger than temp up one position
array[pos+1] = array[pos];
pos--;
}
array[pos] = temp; // place temp into last vacated position
}
}
/**
* Outputs the ints in an array on one line, separated by spaces,
* with a line feed at the end.
*/
private static void printArray(int[] array) {
for (int i = 0; i
System.out.print(" ");
System.out.print(array[i]);
}
System.out.println();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
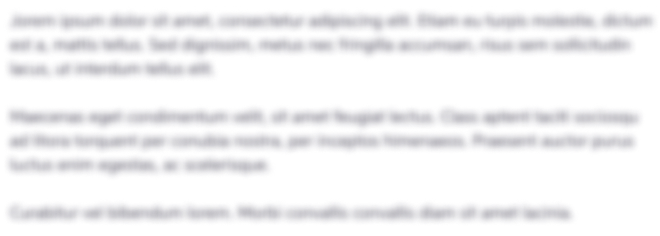
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started