Question
Below, you MUST complete all functions as described in the doc comment below each function declaration. ''' # This line pulls in the functions from
Below, you MUST complete all functions as described in the "doc comment" below each function declaration.
''' # This line pulls in the functions from your other script so that they can be # used in this script. # NOTE: If you don't open VSCode in the same directory as your project, # VSCode might show this as an error and may report the function calls # as errors, as well...they are not...they will execute correctly. from string_funcs import *
def simple_iterate(value_string): ''' This one should be pretty straight forward. Based on a comma-separated string of values (any value), you simply want to print out a line with each of the values using your print_value_line() funciton such that items are prefixed with an item counter. Best described with an example: if you are passed a value_string of "Beef,Jerky,Rules", you'd print out:
ITEM 1 Beef ITEM 2 Jerky ITEM 3 Rules
You'll want to:
1) "split" up the `value_string` by the comma 2) iterate over that list (likely using a `for in range` since you will want a counter to print) 3) call print_value_line() with the appropriate text and value...text will be "ITEM n+1" (assuming n is the index) and value will be...well...the item value.
''' print_header('Simple Iterate') ### PUT YOUR CODE BELOW HERE ###
def adding_machine(numbers): ''' This is really just a more useful variation on the prior function. Here you receive a comma-separated string of integers. You need to add them up, but show your work as you go. This is actually also similar to a problem that you did a while back with lists. Only here you are splitting a string to get your list and then using your string functions to print it out in a prettier fashion.
Here again, the best way to describe this is with an example. If you are passed a `numbers` string of "2,4,8,16", your program should output:
ITEM 3 Rules 0 plus 2 equals 2 2 plus 4 equals 6 6 plus 8 equals 14 14 plus 16 equals 30
So here, you'll want to:
1) Initialize a `subtotal` variable to 0 2) Split the numbers into a list and iterate over it 3) For each of the values in the list you'll: a) construct your "text" ... basically "subtotal plus value equals" b) convert the value to an int and add it to the current subtotal c) call print_value_line() with the text and the new subtotal ''' print_header('Adding Machine') ##### Your code goes below here #####
def shopping_cart(cart_list): ''' This is (unsurprisingly) the most complex, but also...not really. You'll get a LIST of comma-separated strings passed in as the `cart_list` parameter. Each of the strings in the `cart_list` will contain: * the description of an item * the price of an item * the quantity in your cart You want to show the items in the cart along with an extended price (qty * price). Then at the end you want to show the total in the cart.
Again an example is worth a lot:
Assume you are passed the following list: [ 'apple,1.39,2', 'orange,1.11,4', 'plum,0.89,1' ]
You want to display your shopping cart as follows:
apple (2 @ $1.39) 2.78 orange (4 @ $1.11) 4.44 plum (1 @ $0.89) 0.89 ------------------------------------------------------------ Grand Total 8.11
So finally here, you'll want to:
1) Initialize a `subtotal` variable to 0 2) For each of the items in `cart_list` you'll: a) split the item to get three parts (description, price and quantity) b) construct your "text" ... basically "description (quantity @ $price)" c) convert price to a float and quantity to an int d) calculate the extended price (just price * quantity) e) call print_float_line() with your text and your extended price f) add the extended price to your subtotal 3) After printing the items, call print_line('-') to print the line and 4) Call print_float_line one final time with the subtotal to display the "Grand Total"
As an OPTIONAL challenge, you might add a `print_currency_line()` function to string_funcs.py. In addition to printing the float value rounded to 2 decimal places, you could try to prefix the value with a "$" (without breaking the alignment).
''' print_header('Shopping Cart') ##### Your code goes below here #####
############################################################################### ### HERE IS THE "MAIN" PART OF THE SCRIPT... ### IT SIMPLY CALLS THE FUNCTIONS YOU COMPLETED ABOVE. ### NO NEED TO TOUCH ANY OF THE CODE BELOW, BUT DO LOOK AT THE EXPECTED ### OUTPUT TO MAKE SURE IT MATCHES MINE (OR AT LEAST IS CLOSE). ###############################################################################
simple_iterate("5,10,15,20,25") print() simple_iterate("John,Paul,George,Ringo,Bubba") print() adding_machine("5,5,5,5,5") print() adding_machine("192,168,0,1,10,0,0,1,172,16,0,1") print() cart = [ 'Yellow Sneaks,54.95,1', 'Orange Socks,12.99,2', 'Blue Pants,39.99,1', 'Green Shirt,17.95,4' ] shopping_cart(cart)
''' MY OUTPUT FOR THE ABOVE FUNCTION CALLS LOOKS AS FOLLOWS:
Simple Iterate ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ ITEM 1 5 ITEM 2 10 ITEM 3 15 ITEM 4 20 ITEM 5 25
Simple Iterate ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ ITEM 1 John ITEM 2 Paul ITEM 3 George ITEM 4 Ringo ITEM 5 Bubba
Adding Machine ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 0 plus 5 equals 5 5 plus 5 equals 10 10 plus 5 equals 15 15 plus 5 equals 20 20 plus 5 equals 25
Adding Machine ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 0 plus 192 equals: 192 192 plus 168 equals: 360 360 plus 0 equals: 360 360 plus 1 equals: 361 361 plus 10 equals: 371 371 plus 0 equals: 371 371 plus 0 equals: 371 371 plus 1 equals: 372 372 plus 172 equals: 544 544 plus 16 equals: 560 560 plus 0 equals: 560 560 plus 1 equals: 561
Shopping Cart ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ Yellow Sneaks (1 @ $54.95) 54.95 Orange Socks (2 @ $12.99) 25.98 Blue Pants (1 @ $39.99) 39.99 Green Shirt (4 @ $17.95) 71.80 ------------------------------------------------------------ Grand Total 192.72
'''
Step by Step Solution
There are 3 Steps involved in it
Step: 1
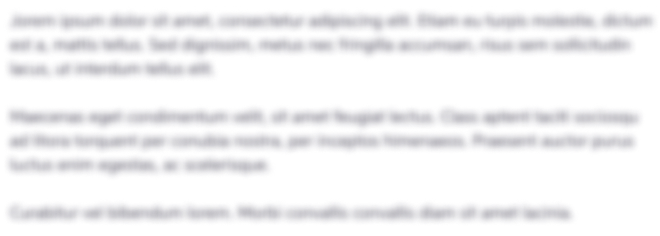
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started