Answered step by step
Verified Expert Solution
Question
1 Approved Answer
BINARYTREE: import java.util.ArrayList; public class BinaryTree { private String data; private BinaryTree leftChild; private BinaryTree rightChild; // A constructor that makes a Sentinel node public
BINARYTREE:
import java.util.ArrayList; public class BinaryTree { private String data; private BinaryTree leftChild; private BinaryTree rightChild; // A constructor that makes a Sentinel node public BinaryTree() { data = null; leftChild = null; rightChild = null; } // This constructor now uses sentinels for terminators instead of null public BinaryTree(String d) { data = d; leftChild = new BinaryTree(); rightChild = new BinaryTree(); } // This constructor is unchanged public BinaryTree(String d, BinaryTree left, BinaryTree right) { data = d; leftChild = left; rightChild = right; } // Get methods public String getData() { return data; } public BinaryTree getLeftChild() { return leftChild; } public BinaryTree getRightChild() { return rightChild; } // Set methods public void setData(String d) { data = d; } public void setLeftChild(BinaryTree left) { leftChild = left; } public void setRightChild(BinaryTree right) { rightChild = right; } // Return a list of all data within the leaves of the tree public ArrayListleafData() { ArrayList result = new ArrayList (); if (data != null) { if ((leftChild.data == null) && (rightChild.data == null)) result.add(data); result.addAll(leftChild.leafData()); result.addAll(rightChild.leafData()); } return result; } // Determines the height of the tree, which is the number of branches // in the path from the root to the deepest leaf. public int height() { // Check if this is a sentinel node if (data == null) return -1; return 1 + Math.max(leftChild.height(), rightChild.height()); } public boolean isTheSameAs(BinaryTree t) { return false; //. Replace this code with your own } }
BINARYTREETESTPROGRAM:
public class BinaryTreeTestProgram { public static void main(String[] args) { BinaryTree root; root = new BinaryTree("A", new BinaryTree("B", new BinaryTree("C", new BinaryTree("D"), new BinaryTree("E", new BinaryTree("F", new BinaryTree("G"), new BinaryTree("I")), new BinaryTree("H"))), new BinaryTree("J", new BinaryTree("K", new BinaryTree(), new BinaryTree("L", new BinaryTree(), new BinaryTree("M"))), new BinaryTree("N", new BinaryTree(), new BinaryTree("O")))), new BinaryTree("P", new BinaryTree("Q"), new BinaryTree("R", new BinaryTree("S", new BinaryTree("T"), new BinaryTree()), new BinaryTree("U")))); System.out.println("Tree Height = " + root.height()); System.out.println("Tree Leaves = " + root.leafData()); } }
BINARYTREETESTPROGRAM2 :
public class BinaryTreeTestProgram2 { public static void main(String[] args) { BinaryTree tree1, tree2, tree3, tree4, tree5; tree1 = new BinaryTree("A", new BinaryTree("B", new BinaryTree("C", new BinaryTree("D"), new BinaryTree("E", new BinaryTree("F", new BinaryTree("G"), new BinaryTree("I")), new BinaryTree("H"))), new BinaryTree("J", new BinaryTree("K", new BinaryTree(), new BinaryTree("L", new BinaryTree(), new BinaryTree("M"))), new BinaryTree("N", new BinaryTree(), new BinaryTree("O")))), new BinaryTree("P", new BinaryTree("Q"), new BinaryTree("R", new BinaryTree("S", new BinaryTree("T"), new BinaryTree()), new BinaryTree("U")))); tree2 = new BinaryTree("A", new BinaryTree("B", new BinaryTree("C", new BinaryTree("D"), new BinaryTree("E", new BinaryTree("F", new BinaryTree("Z"), //different letter here new BinaryTree("I")), new BinaryTree("H"))), new BinaryTree("J", new BinaryTree("K", new BinaryTree(), new BinaryTree("L", new BinaryTree(), new BinaryTree("M"))), new BinaryTree("N", new BinaryTree(), new BinaryTree("O")))), new BinaryTree("P", new BinaryTree("Q"), new BinaryTree("R", new BinaryTree("S", new BinaryTree("T"), new BinaryTree()), new BinaryTree("U")))); tree3 = new BinaryTree("A", new BinaryTree("B", new BinaryTree("C", new BinaryTree("D"), new BinaryTree("E", new BinaryTree("F", new BinaryTree("G"), new BinaryTree("I")), new BinaryTree("H"))), new BinaryTree()), // "J" tree removed new BinaryTree("P", new BinaryTree("Q"), new BinaryTree("R", new BinaryTree("S", new BinaryTree("T"), new BinaryTree()), new BinaryTree("U")))); tree4 = new BinaryTree("A", new BinaryTree("B", new BinaryTree("C", new BinaryTree("D"), new BinaryTree("E", new BinaryTree("F", new BinaryTree("G"), new BinaryTree("I")), new BinaryTree("H"))), new BinaryTree("J", new BinaryTree("K", new BinaryTree(), new BinaryTree("L", new BinaryTree(), new BinaryTree("M"))), new BinaryTree("N", new BinaryTree(), new BinaryTree("O")))), new BinaryTree("P", new BinaryTree("Q"), new BinaryTree("R", new BinaryTree("S", new BinaryTree("T"), new BinaryTree()), new BinaryTree()))); // "U" tree removed tree5 = new BinaryTree("A", new BinaryTree(), new BinaryTree()); // only single node tree System.out.println("Tree 1 is the same as Tree 1 = " + tree1.isTheSameAs(tree1)); // true System.out.println("Tree 1 is the same as Tree 2 = " + tree1.isTheSameAs(tree2)); // false System.out.println("Tree 1 is the same as Tree 3 = " + tree1.isTheSameAs(tree3)); // false System.out.println("Tree 1 is the same as Tree 4 = " + tree1.isTheSameAs(tree4)); // false System.out.println("Tree 1 is the same as Tree 5 = " + tree1.isTheSameAs(tree5)); // false System.out.println("Tree 5 is the same as Tree 1 = " + tree5.isTheSameAs(tree1)); // false System.out.println("Tree 5 is the same as Tree 5 = " + tree5.isTheSameAs(tree5)); // true } }
Picture Tools File Home Format Tell me what you ant to do co Autoser; Com 2 5 :? : Document1 - Word Insert Design Layout References Mailings Review View H elp H X Cut Calibri (Body) - 11 - A A Aa - E-E-F- FEE 2! 1 Paste * Format Painter B 1 U - abc x, x A - ay - A - E== - 2 - - Clipboard Paragraph Sign in - O x le stes TP Find - P Find - 3. Replace Subtitle Subtle Em.. Emphasis - Select - G Editing LR Copy cD bct 1 Normal 1 No Spac... Heading 1 Heading 2 Title Font Styles Editing a Document Recovery Word has recovered the following files. Save the ones you wish to keep. 2) In the tutorial code provided, there is a binary free class that represents the sentinel version of the binary tree data structure described in the notes (in the notes it is called Binary Tree 2, whereas here is called simply Binary Tree). A BinartTree Test Program class has been provided which creates the tree shown below and also prints out the height of the tree as well as the leaves of the tree Run the code to make sure that works correctly. Document1 (AutoRecovered] Version created from the last. 3/22/2018 4:56 PM Write a recursive instance method in the Binary Tree class called contains (String d) which returns a boolean indicating whether or not the tree contains the data passed in as a parameter. Add the following Ines to the end of the BinaryTreeTest Program to test your code: System.out.println("Tree centaine A r eet.contains ("A") //true System.out.println("Tree contains E- root. contains ("E") /true System.out.println("Tres contains ...root. contains (**): //tale ? Which file do I want to save? 3) write an recursive instance method in the Binaryfree class called IsTheSameAs(BinaryTree t) which returns a boolean indicating whether or not the tree is the same as the one passed in as a parameter. It should be the same if the nodes are the same and the data in each node matches. Use the supplied BinaryTree TestProgram2 program to test your code. Close Page 2 of 3 Owords OP D + 100% + 10096
Step by Step Solution
There are 3 Steps involved in it
Step: 1
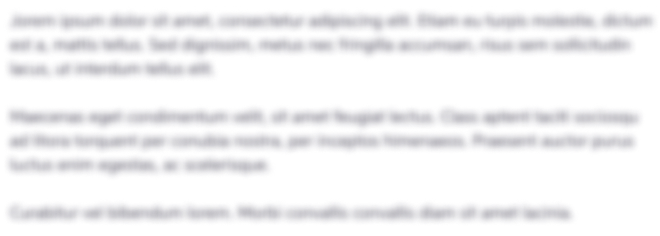
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started