Question
BlackJack game for project in python Sample Run: Given Code: Objects.py #!/usr/bin/env python3 import random CARDS_CHARACTERS = {Spades: , Hearts: , Diamonds: , Clubs: }
BlackJack game for project in python
Sample Run:
Given Code:
Objects.py
#!/usr/bin/env python3 import random CARDS_CHARACTERS = {"Spades": "", "Hearts": "", "Diamonds": "", "Clubs": ""}
class Card: def __init__(self, rank, suit): self.rank=rank self.suit=suit @property def value(self): if(self.rank=="Ace"): return 11 elif(self.rank=="Jack" or self.rank=="Queen" or self.rank=="King"): return 10 else: return int(self.rank) def displayCard(self): return self.rank+" of "+self.suit+" "+CARDS_CHARACTERS[self.suit] class Deck: def __init__(self): suits=["Spades", "Hearts", "Diamonds", "Clubs"] ranks=["Ace", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King"] self.__deck=[] for i in suits: for j in ranks: self.__deck.append(Card(j, i)) @property def count(self): return len(self.__deck) def shuffle(self): random.shuffle(self.__deck) def dealCard(self): cardPos=random.randint(0, self.count) dealt=self.__deck[cardPos] del self.__deck[cardPos] return dealt class Hand: def __init__(self): self.__cards=[] @property def count(self): return len(self.__cards) @property def points(self): point=0 for i in self.__cards: point+=i.value return point def addCard(self, card): self.__cards.append(card) def displayHand(self): for i in self.__cards: print(i.displayCard())
def main(): print("Cards - Tester") print()
#test sorting of the cards testcardsList = [Card("Ace","Spades"), Card("Queen","Hearts"), Card("10","Clubs"), Card("3","Diamonds"), Card("Jack","Hearts"), Card("7","Spades")] testcardsList.sort() print("TEST CARDS LIST AFTER SORTING.") for c in testcardsList: print(c) print()
# test deck print("DECK") deck = Deck() print("Deck created.") deck.shuffle() print("Deck shuffled.") print("Deck count:", len(deck)) print()
# test hand hand = Hand() for i in range(10): hand.addCard(deck.dealCard())
print("SORTED HAND") for c in sorted(hand): print(c)
print() print("Hand points:", hand.points) print("Hand count:", len(hand)) print("Deck count:", len(deck))
if __name__ == "__main__": main()
Given Code:
Blackjack.py
#!/usr/bin/env python3
from objects import Card, Deck, Hand
class Blackjack: def __init__(self, startingBalance): self.money = startingBalance self.deck = Deck() self.playerHand = Hand() self.dealerHand = Hand() self.bet = 0
def displayCards(self,hand, title): ''' Print the title and display the cards in the given hand in a sorted order''' print(title.upper()) for c in sorted(hand): print(c) print()
def handle_winner(self, winner, message): ''' print the player's hand's points print the message Update self.money according to the winner ''' pass #To be implemented in Phase 2 def getBet(self): ''' Method to update self.bet by prompting the user for the bet amount, making sure bet is less than self.money. ''' pass #To be implemented in Phase 2 def setupRound(self): ''' Setup the round by doing these steps: Call getBet to initialize self.bet, initialize self.deck to a new Deck object and shuffle it initialize self.dealerHand and self.playerHand to new Hand objects deal two cards to the playerHand, and one card to the dealerHand finally, print dealerHand and playerHand using displayCards method ''' pass # To be implemented in Phase 2
def play_playerHand(self): ''' Method to implement player playing his hand by 1. Prompting the user to indicate Hit (h) or Stand (s) 2. If user picks stand, end the player play by returning 3. If user picks hit, deal a card to the playerHand. check if with the latest addition, the hand busts (has > 21 points), if so return otherwise, prompt the player again whether to hit or stand. 4. Print playerHand points ''' pass # To be implemented in Phase 2 def play_dealerHand(self): ''' Method to play the dealer's hand. Continue to deal cards till the points of the dealerHand are less than 17. Print the dealer's hand before returning''' pass # To be implemented in Phase 3 def playOneRound(self): ''' Method implements playing one round of the game 1. Checks if playerHand is a Blackjack, if so handles that case 2. Lets player play his hand if it busts, declares player loser 3. Else lets dealer play his hand. 4. If dealer busts, declares the player to be the winner 5. Otherwise declares the winner based on who has higher points: if Player > dealer, player is the winner else if player
def main(): print("BLACKJACK!") print("Blackjack payout is 3:2")
# initialize starting money money = 100 print("Starting Balance:", money)
blackjack = Blackjack(money) # start loop again = 'y' while again.lower() == 'y':
print("Setting up a round...") blackjack.setupRound()
print("Playing Player Hand...") blackjack.play_playerHand()
print() again = input("Play again? (y): ").lower() print() if again != "y": break
print("Bye!")
# if started as the main module, call the main function if __name__ == "__main__": main()
Sample Run:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
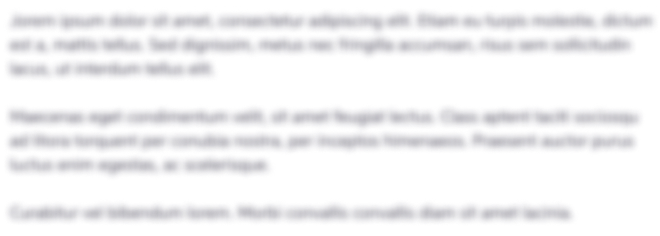
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started