Question
BmpProcessor.h struct BMP_Header { //TODO:Finish struct }; struct DIB_Header{ //TODO:Finish struct }; /** * read BMP header of a file. Useful for converting files from
BmpProcessor.h
struct BMP_Header { //TODO:Finish struct };
struct DIB_Header{ //TODO:Finish struct };
/** * read BMP header of a file. Useful for converting files from PPM to BMP. * * @param file: A pointer to the file being read * @param header: Pointer to the destination BMP header */ void readBMPHeader(FILE* file, struct BMP_Header* header);
/** * write BMP header of a file. Useful for converting files from PPM to BMP. * * @param file: A pointer to the file being written * @param header: The header to write to the file */ void writeBMPHeader(FILE* file, struct BMP_Header* header);
/** * read DIB header from a file. Useful for converting files from PPM to BMP. * * @param file: A pointer to the file being read * @param header: Pointer to the destination DIB header */ void readDIBHeader(FILE* file, struct DIB_Header* header);
/** * write DIB header of a file. Useful for converting files from PPM to BMP. * * @param file: A pointer to the file being written * @param header: The header to write to the file */ void writeDIBHeader(FILE* file, struct DIB_Header* header);
/** * make BMP header based on width and height. * The purpose of this is to create a new BMPHeader struct using the information * from a PPMHeader when converting from PPM to BMP. * * @param header: Pointer to the destination DIB header * @param width: Width of the image that this header is for * @param height: Height of the image that this header is for */ void makeBMPHeader(struct BMP_Header* header, int width, int height);
/** * Makes new DIB header based on width and height. Useful for converting files from PPM to BMP. * * @param header: Pointer to the destination DIB header * @param width: Width of the image that this header is for * @param height: Height of the image that this header is for */ void makeDIBHeader(struct DIB_Header* header, int width, int height);
/** * read Pixels from BMP file based on width and height. * * @param file: A pointer to the file being read * @param pArr: Pixel array to store the pixels being read * @param width: Width of the pixel array of this image * @param height: Height of the pixel array of this image */ void readPixelsBMP(FILE* file, struct Pixel** pArr, int width, int height);
/** * write Pixels from BMP file based on width and height. * * @param file: A pointer to the file being read or written * @param pArr: Pixel array of the image to write to the file * @param width: Width of the pixel array of this image * @param height: Height of the pixel array of this image */ void writePixelsBMP(FILE* file, struct Pixel** pArr, int width, int height);
PixelProcessor.h
struct Pixel{ //TODO:Finish struct };
/** * Shift color of Pixel array. The dimension of the array is width * height. The shift value of RGB is * rShift, gShiftbShift. Useful for color shift. * * @param pArr: Pixel array of the image that this header is for * @param width: Width of the image that this header is for * @param height: Height of the image that this header is for * @param rShift: the shift value of color r shift * @param gShift: the shift value of color g shift * @param bShift: the shift value of color b shift */ void colorShiftPixels(struct Pixel** pArr, int width, int height, int rShift, int gShift, int bShift);
PpmProcessor.h
struct PPM_Header{ //TODO:Finish struct };
/** * read PPM header of a file. Useful for converting files from BMP to PPM. * * @param file: A pointer to the file being read or written * @param header: Pointer to the destination PPM header */ void readPPMHeader(FILE* file, struct PPM_Header* header);
/** * write PPM header of a file. Useful for converting files from BMP to PPM. * * @param file: A pointer to the file being read or written * @param header: The header to write to the file
*/ void writePPMHeader(FILE* file, struct PPM_Header* header);
/** * make PPM header based on width and height. Useful for converting files from BMP to PPM. * * @param header: Pointer to the destination PPM header * @param width: Width of the image that this header is for * @param height: Height of the image that this header is for */ void makePPMHeader(struct PPM_Header* header, int width, int height);
/** * read Pixels from PPM file based on width and height. * * @param file: A pointer to the file being read * @param pArr: Pixel array to store the pixels being read * @param width: Width of the pixel array of this image * @param height: Height of the pixel array of this image */ void readPixelsPPM(FILE* file, struct Pixel** pArr, int width, int height);
/** * write Pixels from PPM file based on width and height. * * @param file: A pointer to the file being read or written * @param pArr: Pixel array of the image to write to the file * @param width: Width of the pixel array of this image * @param height: Height of the pixel array of this image */ void writePixelsPPM(FILE* file, struct Pixel** pArr, int width, int height);
HeaderUsageSample.c
/** * THIS IS NOT A BASE FILE. * * The following code is a minimal example showing how to apply a blue color shift to the ttt.bmp test file using * the functionality you will implement in BmpProcessor.h and PpmProcessor.h. It serves as an example of the syntax that * your implementation should support. This code will not function until you have completed all the functionality in the * header files. * * DO NOT SUBMIT THIS FILE WITH YOUR HOMEWORK. * * Completion time: 334 minutes * * @author Vatricia Edgar, Ruben Acuna * @version 1.0 */
//////////////////////////////////////////////////////////////////////////////// //INCLUDES #include
void main() { //START - HEADER FUNCTIONALITY MINIMAL EXAMPLE (DO NOT SUBMIT) struct BMP_Header BMP; struct DIB_Header DIB;
FILE* file_input = fopen("ttt.bmp", "rb"); readBMPHeader(file_input, &BMP); readDIBHeader(file_input, &DIB);
struct Pixel** pixels = (struct Pixel**) malloc(sizeof(struct Pixel*)*152); for(int p = 0; p
colorShiftPixels(pixels, 152, 152, 0, 0, 20); // should show yellow by nose.
FILE* file_output = fopen("ttt_output.bmp", "wb"); writeBMPHeader(file_output, &BMP); writeDIBHeader(file_output, &DIB); writePixelsBMP(file_output, pixels, 152, 152); fclose(file_output);
//END - HEADER FUNCTIONALITY MINIMAL EXAMPLE (DO NOT SUBMIT) }
Image Processing Summary: In this homework, you will be implementing a program that loads an image file, applies a simple filter to it, and saves it back to disk. You will also learn how to read and write real-world binary file formats, specifically BMP and PPM. 1 Background In this assignment you will write a program that applies a filter to an image. The image will be loaded from a local BMP or PPM file, specified by the user, and then be transformed using a color shift also specified by the user. The resulting file will be saved back to a BMP or PPM file that can be viewed on the host system. This document is separated into four sections: Background, Requirements, Loading Binary Files, Include Files, and Submission. You have almost finished reading the Background section already. In Requirements, we will discuss what is expected of you in this homework. In Loading Binary Files, we will discuss the BMP and PPM file formats and how to interact with binary files. Lastly, Submission discusses how your source code should be submitted on Canvas. 2 Requirements (36 points] Your program needs to implement loading a binary BMP or PPM file, applying a color shift to its pixel data, and saving the modified image back into a BMP or PPM file. The width and height may be of any size, but you can assume that you will be capable of storing the image data in memory. Assume that all BMP files are 24-bit uncompressed files" (i.e., compression method is BI_RGB), which have a 14-bit bmp header, a 40-bit dib header, and a variable length pixel array. This is shown in Figure 1] PPM files will also be in 24-bit. For testing, please only use the PPM and BMP files attached to the assignment. As a base requirement, your program must compile and run under Xubuntu (or another variant of Ubuntu) 18.04. Sample output for this program is shown in Figure 1 Specific Requirements: BMP Headers IO: Create structures for the headers of a BMP file (BMP header struct and DIB header struct) and functions which read and write them. [4 Points] PPM Headers 10: Create structures for the headers of a PPM file (PPM header struct) and functions which read and write them. [4 Points] Pixel 10: Create a structure which represents a single pixel (24-bit pixel struct) and the functions for reading and writing all pixels in both PPM and BMP files. [4 Point] Input and output file names: Read the input file name and output file name from the command line arguments. The user should be required to enter the input file name and this should be the first argument. The output file name is an "option," it is not required and it can be in any place in the option list. If an output filename is not provided, then you should create a default file called result, whose extension is based on the input file. The output file option is specified by -0" followed by the output file name. Validate that the input file exists and that both files are of the correct type (either bmp or ppm). [4 Points] * Commands might look like "./ImageProcessor ttt.bmp-r 56 -b 78 -g 45 -o nehoy menoy.ppm". * The input file is the file to read and copy. In case you think this is making the assignment unrealistic, think again: this is the default Windows 10 BMP file format. Image Processing Summary: In this homework, you will be implementing a program that loads an image file, applies a simple filter to it, and saves it back to disk. You will also learn how to read and write real-world binary file formats, specifically BMP and PPM. 1 Background In this assignment you will write a program that applies a filter to an image. The image will be loaded from a local BMP or PPM file, specified by the user, and then be transformed using a color shift also specified by the user. The resulting file will be saved back to a BMP or PPM file that can be viewed on the host system. This document is separated into four sections: Background, Requirements, Loading Binary Files, Include Files, and Submission. You have almost finished reading the Background section already. In Requirements, we will discuss what is expected of you in this homework. In Loading Binary Files, we will discuss the BMP and PPM file formats and how to interact with binary files. Lastly, Submission discusses how your source code should be submitted on Canvas. 2 Requirements (36 points] Your program needs to implement loading a binary BMP or PPM file, applying a color shift to its pixel data, and saving the modified image back into a BMP or PPM file. The width and height may be of any size, but you can assume that you will be capable of storing the image data in memory. Assume that all BMP files are 24-bit uncompressed files" (i.e., compression method is BI_RGB), which have a 14-bit bmp header, a 40-bit dib header, and a variable length pixel array. This is shown in Figure 1] PPM files will also be in 24-bit. For testing, please only use the PPM and BMP files attached to the assignment. As a base requirement, your program must compile and run under Xubuntu (or another variant of Ubuntu) 18.04. Sample output for this program is shown in Figure 1 Specific Requirements: BMP Headers IO: Create structures for the headers of a BMP file (BMP header struct and DIB header struct) and functions which read and write them. [4 Points] PPM Headers 10: Create structures for the headers of a PPM file (PPM header struct) and functions which read and write them. [4 Points] Pixel 10: Create a structure which represents a single pixel (24-bit pixel struct) and the functions for reading and writing all pixels in both PPM and BMP files. [4 Point] Input and output file names: Read the input file name and output file name from the command line arguments. The user should be required to enter the input file name and this should be the first argument. The output file name is an "option," it is not required and it can be in any place in the option list. If an output filename is not provided, then you should create a default file called result, whose extension is based on the input file. The output file option is specified by -0" followed by the output file name. Validate that the input file exists and that both files are of the correct type (either bmp or ppm). [4 Points] * Commands might look like "./ImageProcessor ttt.bmp-r 56 -b 78 -g 45 -o nehoy menoy.ppm". * The input file is the file to read and copy. In case you think this is making the assignment unrealistic, think again: this is the default Windows 10 BMP file format
Step by Step Solution
There are 3 Steps involved in it
Step: 1
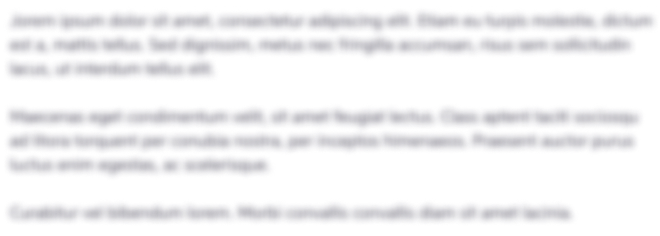
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started