Question
//Book.java package bookStore; public class Book extends Product { private String ISBN; public Book(int pID, String bISBN, int noOfProduct) { // TODO Auto-generated constructor stub
//Book.java
package bookStore;
public class Book extends Product {
private String ISBN;
public Book(int pID, String bISBN, int noOfProduct) {
// TODO Auto-generated constructor stub
super(pID, noOfProduct);
this.ISBN = bISBN;
}
public String getISBN() {
return ISBN;
}
public void setISBN(String iSBN) {
ISBN = iSBN;
}
}
//CD.java
package bookStore;
public class CD extends Product {
public CD(int pID, int noOfProduct) {
super(pID, noOfProduct);
// TODO Auto-generated constructor stub
}
}
//DVD.java
package bookStore;
public class DVD extends Product {
public DVD(int pID, int noOfProduct) {
super(pID, noOfProduct);
// TODO Auto-generated constructor stub
}
}
//Member.java
package bookStore;
public class Member {
private int id;
private String firstName;
private String lastName;
private double moneySpent;
public Member(int id, String fName, String lName) {
this.id = id;
this.firstName = fName;
this.lastName = lName;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public double getMoneySpent() {
return moneySpent;
}
public void setMoneySpent(double moneySpent) {
this.moneySpent = moneySpent;
}
}
//PremiumMember.java
package bookStore;
public class PremiumMember extends Member {
private double feeMonthly;
private String paymentMethod;
private boolean feePaidOnTime;
public PremiumMember(int id, String fName, String lName) {
super(id, fName, lName);
}
public double getFeeMonthly() {
return feeMonthly;
}
public void setFeeMonthly(double feeMonthly) {
this.feeMonthly = feeMonthly;
}
public String getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(String paymentMethod) {
this.paymentMethod = paymentMethod;
}
public boolean isFeePaidOnTime() {
return feePaidOnTime;
}
public void setFeePaidOnTime(boolean feePaidOnTime) {
this.feePaidOnTime = feePaidOnTime;
}
}
//Product.java
package bookStore;
public class Product {
private int count;
private int productID;
public Product(int pID, int noOfProduct) {
// TODO Auto-generated constructor stub
this.productID = pID;
this.count = noOfProduct;
}
public int getProductID() {
return productID;
}
public void setProductID(int productID) {
this.productID = productID;
}
public int getCount() {
return count;
}
public void setCount(int count) {
this.count = count;
}
}
//Store.java
package bookStore;
import java.util.ArrayList;
import java.util.Scanner;
public class Store {
public static ArrayList
public static ArrayList
public static Scanner sc = new Scanner(System.in);
private static void CreateInventory() {
int pID, noOfProduct;
String isBook, bISBN;
Book book;
CD cd;
DVD dvd;
System.out.println("Press Y if you want to store book in inventory");
System.out.println("Press N if you want to store CD in inventory");
System.out.println("Press NO if you want to store DVD in inventory");
isBook = sc.next();
while (true) {
System.out.println("Please enter product id");
pID = sc.nextInt();
System.out.println("Please enter number of product");
noOfProduct = sc.nextInt();
if (isBook.equalsIgnoreCase("Y")) {
System.out.println("Please enter book ISBN number");
bISBN = sc.next();
book = new Book(pID, bISBN, noOfProduct);
inventory.add(book);
} else if (isBook.equalsIgnoreCase("N")) {
cd = new CD(pID, noOfProduct);
inventory.add(cd);
} else {
dvd = new DVD(pID, noOfProduct);
inventory.add(dvd);
}
System.out.println("Press Y if you want to add another item in the inventory");
System.out.println("Press N if you do not want to add another item in the inventory");
isBook = sc.next();
if (isBook.equalsIgnoreCase("N")) {
break;
}
}
}
private static boolean findProduct(int pID) {
for (Product p : inventory) {
if (p.getProductID() == pID) {
return true;
}
}
return false;
}
private static boolean CheckInventory(int pID, int pCount) {
for (Product p : inventory) {
if (p.getProductID() == pID) {
if (p.getNoOfProducts() >= pCount) {
p.setNoOfProducts(p.getNoOfProducts() - pCount);
return true;
} else {
System.out.println("There are not enough products in the inventory.");
return false;
}
}
}
System.out.println("Product not found in inventory.");
return false;
}
private static void DistributeItem() {
int pID, pCount, id;
String premium = null, paymentMethod = null;
double fee = 0;
PremiumMember member = null;
System.out.println("Please enter product id");
pID = sc.nextInt();
if (findProduct(pID)) {
System.out.println("Please enter number of products to distribute");
pCount = sc.nextInt();
if (CheckInventory(pID, pCount)) {
System.out.println("Please enter member id");
id = sc.nextInt();
System.out.println("Please enter member first name");
String fName = sc.next();
System.out.println("Please enter member last name");
String lName = sc.next();
System.out.println("Press Y if the member is premium");
premium = sc.next();
member = new PremiumMember(id, fName, lName);
if (premium.equalsIgnoreCase("Y")) {
member.setFeeMonthly(fee);
member.setPaymentMethod(paymentMethod);
}
}
}
}
public static void main(String[] args) {
// Create array of Product class
CreateInventory();
while (true) {
// Distribute item from inventory
DistributeItem();
String check;
System.out.println("Do you want to distribute more items? Press Y for Yes or any other key for No");
check = sc.next();
if (!check.equalsIgnoreCase("Y")) {
break;
}
}
}
}
EDIT THE 7 CLASSES SO THE CODE CAN RUN AND HAVE AN OUTPUT. THERE ARE ERRORS THAT DO NOT MAKE THE CODE RUN. PLEASE HELP.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
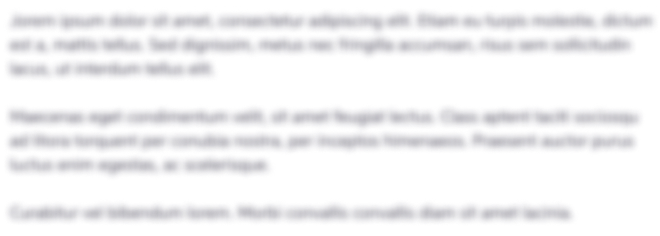
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started