Question
//books.txt Thomas Jefferson and the Tripoli Pirates:GENRE_HISTORY:Brian Kilmeade,Don Yaeger:ABEW2345:234 Component-oriented programming:GENRE_ENGINEERING:C. Szyperski,J. Bosch,W. Weck:HGNH4567:456 Microfabricated microneedles, a novel approach to transdermal drug delivery:GENRE_ENGINEERING:S. Henry,D. V.
//books.txt
Thomas Jefferson and the Tripoli Pirates:GENRE_HISTORY:Brian Kilmeade,Don Yaeger:ABEW2345:234 Component-oriented programming:GENRE_ENGINEERING:C. Szyperski,J. Bosch,W. Weck:HGNH4567:456 Microfabricated microneedles, a novel approach to transdermal drug delivery:GENRE_ENGINEERING:S. Henry,D. V. McAllister,M. G. Allen:HJKG2342:378 Nikola Tesla:GENRE_HISTORY:Sean Patrick:NJKG7456:987 English landscaping and literature, 1660-1840:GENRE_LITERATURE:E. Malins:MNBV3456:980 A history and theory of informed consent:GENRE_HISTORY:R. R. Faden,T. L. Beauchamp,N. M. King:HJGF7645:654 The Feminist Companion to Literature in English Women Writers From the Middle Ages to the Present:GENRE_LITERATURE:V. Blain,P. Clements,I. Grundy:JHGF9089:767 Climate and atmospheric history of the past 420,000 years:GENRE_HISTORY:J. R. Petit, J. Jouzel,D. Raynaud,N. I. Barkov,J. M. Barnola:FDST9878:675 The comparative method in evolutionary biology:GENRE_SCIENCE:P. H. Harvey,M. D. Pagel:BGHF8976:234 Human-computer interaction:GENRE_ENGINEERING:J. Preece,Y. Rogers,H. Sharp,D. Benyon,S. Holland:UYHG1223:889 Free radicals in biology and medicine:GENRE_SCIENCE:B. Halliwell,J. M. C. Gutteridge:HGHB8909:234 Electron transfers in chemistry and biology:GENRE_SCIENCE:R. A. Marcus,N. Sutin:LKJH2345:890 English landscaping and literature, 1660-1840:GENRE_LITERATURE:E. Malins:MNBV3456:980 Device electronics for integrated circuits:GENRE_ENGINEERING:R. S. Muller,T. I. Kamins,M. Chan,P. K. Ko:JHKG2343:654 An outline of English literature:GENRE_LITERATURE:G. C. Thornley,G. Roberts:YUTY9098:89 Gene Ontology:GENRE_SCIENCE:M. Ashburner,C. A. Ball,J. A. Blake,D. Botstein,H. Butler:KJHG8909:90 Nikola Tesla:GENRE_HISTORY:Sean Patrick:NJKG7456:987 Microfabricated microneedles, a novel approach to transdermal drug delivery:GENRE_ENGINEERING:S. Henry,D. V. McAllister,M. G. Allen:HJKG2342:378
//BookRecord class
package library.service.classes;//set up the package
import library.service.classes.BookGenre;
public class BookRecord{
int recordNo;
String title;
String []authors;
BookGenre genre;
static int cnt=10000;
int pages=0;
String tag;
/on-default constructor
public BookRecord(String title, String genre, String [] name,String tag,int pages){
this.setTitle(title);
this.setGenre(genre);
this.setAuthors(name);
this.setRecordNo();
this.setTag(tag);
this.setPages(pages);
}
//accessors
public String getTitle(){
return this.title;
}
public BookGenre getGenre(){
return this.genre;
}
public String [] getAuthors(){
String []ret = new String[this.authors.length];
for(int i=0;i ret[i]=this.authors[i]; } return ret; } public String getAuthorList(){ String ret=""; for(int i=0;i ret=ret + " " +this.authors[i]; } return ret; } public String getTag(){ return this.tag; } public int getPages(){ return this.pages; } public int getRecordNo(){ return this.recordNo; } //mutators public void setRecordNo(){ this.recordNo=BookRecord.cnt++; } public void setTitle(String title){ this.title=title; } public void setGenre(String genre){ this.genre=BookGenre.valueOf(genre); } public void setPages(int pages){ this.pages=pages; } public void setTag(String tag){ this.tag=tag; } public void setAuthors(String []authorList){ this.authors = new String[authorList.length]; for(int i=0;i this.authors[i]=authorList[i]; } } //toString method public String toString(){ String str=""; str = str + "=================================== "; str = str + "Record No:" + this.getRecordNo() + " "; str = str + "Tag:" + this.getTag() + " "; str = str + "Title:" + this.getTitle() + " "; str = str + "Genre: " + this.getGenre() + " "; str = str + "Authors: " + this.getAuthorList() + " "; str = str + "No. of Pages: " + this.getPages() + " "; str = str + "=================================== "; return str; } //toEquals method public boolean equals(BookRecord aRecord){//remember you should not compare the record no if(!this.getTitle().equals(aRecord.getTitle())) return false; if(!this.equalsHelper(aRecord)) return false; if(!(this.getPages() == aRecord.getPages())) return false; return true; } private boolean equalsHelper(BookRecord aRecord){ for(int i=0;i if(!this.authors[i].equals(aRecord.authors[i])){ //assume the authors will always be provided in the same order return false; } } return true; } } //BookGenre class package library.service.classes; public enum BookGenre {GENRE_HISTORY,GENRE_SCIENCE,GENRE_ENGINEERING,GENRE_LITERATURE}; //library class //Please modify this class using ArrayList/Vector package library.client.classes; import java.util.Scanner; import java.io.File; import java.io.IOException; import library.service.classes.BookGenre; import library.service.classes.BookRecord; class library{ /* You will use a Vector or ArrayList (upto you) to store the details of the books as a collection of BookRecord objects. Since you are using Vector/ArrayList, you dont have to worry about resizing. */ //Implement a class method called sortString() with the following signature: public ArrayList } //Implement a second class method called sortPages() with the following signature: public Vector } public void searchByGenre(BookGenre genre){ //loop through the book records and find the number of matches int noMatches=0; for(int i=0;i if(this.books[i].getGenre()==genre){ noMatches++; } } if(noMatches==0){ System.out.println("No books of this genre found"); return; } BookRecord [] searchResults = new BookRecord[noMatches]; int count=0; for(int i=0;i if(this.books[i].getGenre()==genre){ searchResults[count++]=this.books[i]; } } //sort the results according to the page lenght this.sortPages(searchResults,searchResults.length); //print out the results for(int i=0;i System.out.println(searchResults[i].toString()); } } public void searchTag(String tag){ { //implement the binary search /* This method will take in a tag value as a parameter. It should search the BookRecord objects in the Vector/ArrayList, using Binary Search, for a particular tag value and display the record (call the toString() method of the record). */ } System.out.println("No match found"); } /* Note that the book records in your Vector/ArrayList should be unique. However the text file may contain duplicate records. Therefore you will need to check for duplicated records before you insert them in the Vector/ArrayList. Every time you find a duplicate, print out the record. Two records are said to be identical if their title, authors and genre are the same. Assume that the authors for a particular title will always be provided in the input text file in the same order. */ public boolean searchForDuplicate(BookRecord aRecord){ } public void print(){//list the library for(int i=0;i System.out.println(this.books[i].toString()); } } library(){ this.books=new BookRecord[5];//intial size is 5 } public static void main(String []args){//instantiate the library //arg[0]: text file //arg[1]: resize factor int resizeFactor = Integer.parseInt(args[1]); library myLib = new library(); //read the the files from text files String []authors; BookRecord aRecord; Scanner scan; String str; try { File myFile=new File(args[0]); scan=new Scanner(myFile);//each line has the format title:genre:author-name-1,author-name-2..authorname-m while(scan.hasNextLine()){ str=scan.nextLine(); String []tok=str.split(":"); authors=tok[2].split(","); aRecord = new BookRecord(tok[0],tok[1],authors,tok[3],Integer.parseInt(tok[4])); //check for duplicate records if (!myLib.searchForDuplicate(aRecord)){ //create a BookRecord object and load it on the array myLib.books[myLib.noRecords] = aRecord; myLib.noRecords++; //System.out.println("No of records: " + myLib.noRecords); } else{ System.out.println("Found a duplicate"); String out=""; out = out + "=================================== "; out = out + "Tag:" + aRecord.getTag() + " "; out = out + "Title:" + aRecord.getTitle() + " "; out = out + "Genre: " + aRecord.getGenre() + " "; out = out + "Authors: " + aRecord.getAuthorList() + " "; out = out + "No. of Pages: " + aRecord.getPages() + " "; out = out + "=================================== "; System.out.println(out); } //check if the array needs to resize if(myLib.books.length == myLib.noRecords){ /eed to add additional space myLib.resize(resizeFactor); } } scan.close(); }catch(IOException ioe){ System.out.println("The file can not be read"); } //sort the array myLib.sortString(myLib.books,myLib.noRecords); //User interactive part String option1, option2; scan = new Scanner(System.in); while(true){ System.out.println("Select an option:"); System.out.println("Type \"S\" to list books of a genre"); System.out.println("Type \"P\" to print out all the book records"); System.out.println("Type \"T\" to search for a record with a specific tag"); System.out.println("Type \"Q\" to Quit"); option1=scan.nextLine(); switch (option1) { case "S": System.out.println("Type a genre. The genres are:"); for (BookGenre d : BookGenre.values()) { System.out.println(d); } option2=scan.nextLine(); //assume the use will type in a valid genre myLib.searchByGenre(BookGenre.valueOf(option2)); break; case "P": myLib.print(); break; case "Q": System.out.println("Quitting program"); System.exit(0); case "T": System.out.println("Input the tag of the book you want to search for:"); option2=scan.nextLine(); //assume the use will type in a valid tag myLib.searchTag(option2); break; default: System.out.println("Wrong option! Try again"); break; } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
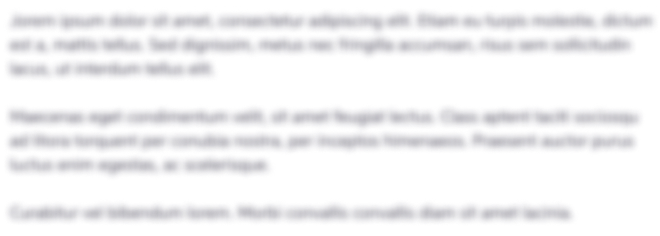
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started