Question
Bubble Sort (Required Exercise) In this exercise the goal is to implement the Bubble Sort algorithm on a small array. If you are comfortable with
Bubble Sort (Required Exercise)
In this exercise the goal is to implement the Bubble Sort algorithm on a small array. If you are comfortable with loops and arrays, you can solve this by implementing the full algorithms. If you are not, you can "unroll" the loop and implement each step by hand by hard coding each pass through the array as a sequence of explicit checks and potential swaps.
The skeleton contains two methods:
-
A main method which will allow you to do your own testing, and give a working example.
-
A bubbleSort method where you'll do the actual implementation of the sorting algorithm.
The bubbleSort method takes in an int[] called data. This is the array that you are to sort. You are guaranteed for this exercise that data will have length 4. At the end of the method you should return data;. This line has already been added, so you can just leave it as is.
For the tests to ensure you are correctly implementing a Bubble Sort (and not some other sort, or using a library), you must print out the array using printArray(data) every time you swap elements in the array. You should not print it out any other time in the bubbleSort method.
------------------------------------------------------------------
Failed: At least one swap was incorrect. ==> expected: <[45, 33, 93, 55] [45, 33, 55, 93] [33, 45, 55, 93]> but was:
-----------------------------------------------------------------------------------------------------------
import java.util.Arrays;
// importing Arrays from the util package public class BubbleSort { private static void printArray(int[] a) { System.out.println(Arrays.toString(a)); } public static int[] bubbleSort(int[] data) { int num=data.length; for (int p=0; p
Step by Step Solution
There are 3 Steps involved in it
Step: 1
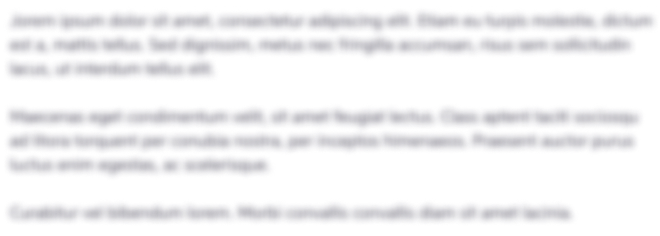
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started