Question
build TCP server in c (just need to fill the missing parts) /* http_server.c - http 1.0 server */ #include #include #include #include #include #include
build TCP server in c (just need to fill the missing parts)
/* http_server.c - http 1.0 server */
#include #include #include #include #include #include #include #include #include #include #include "config.h" #include "helpers.h"
/*------------------------------------------------------------------------ * Program: http server * * Purpose: allocate a socket and then repeatedly execute the following: * (1) wait for the next connection from a client * (2) read http request, reply to http request * (3) close the connection * (4) go back to step (1) * * Syntax: http_server [ port ] * * port - protocol port number to use * * Note: The port argument is optional. If no port is specified, * the server uses the port specified in config.h * *------------------------------------------------------------------------ */
int main(int argc, char *argv[]) { struct sockaddr_in serv_addr; /* structure to hold server's address */ int listen_socket, connection_socket; int port; pid_t pid; /* id of child process to handle request */ char response_buffer[MAX_HTTP_RESP_SIZE]; int status_code; char * status_phrase;
/* 1) Create a socket */ /* Check command-line argument for port and extract */ /* port number if one is specified. Otherwise, use default */
if (argc > 1) { /* if argument specified */ port = atoi(argv[1]); /* convert from string to integer */ } else { port = DEFAULT_PORT; } if (port <= 0) { /* test for legal value */ fprintf(stderr, "bad port number %d", port); exit(EXIT_FAILURE); }
/* 2) Set the values for the server address structure: serv_addr */ memset(&serv_addr,0,sizeof(serv_addr)); /* clear sockaddr structure */
/* 3) Bind the socket to the address information set in serv_addr */
/* 4) Start listening for connections */
/* Main server loop - accept and handle requests */
while (true) {
/* 5) Accept a connection */
/* Fork a child process to handle this request */
if ((pid = fork()) == 0) {
/*----------START OF CHILD CODE----------------*/ /* we are now in the child process */ /* child does not need access to listen_socket */ if ( close(listen_socket) < 0) { fprintf(stderr, "child couldn't close listen socket"); exit(EXIT_FAILURE); }
struct http_request new_request; // defined in httpreq.h /* 6) call helper function to read the request * * this will fill in the struct new_request for you * * see helper.h and httpreq.h */
/*** PART A: PRINT OUT * URI, METHOD and return value of Parse_HTTP_Request() */
/**** PART B ONLY *******/
/* 7) Decide which status_code and reason phrase to return to client */
//
The requested resource is available
The requested resource does not exist
The requested method isn't implemented (your server will only implement GET and HEAD methods)
The client sent an invalid request
// set the reply to send sprintf(response_buffer, "HTTP/1.0 %d %s ", /*** FILL IN ***/, /*** FILL IN ***/); printf("Sending response line: %s ", response_buffer); send(/*** FILL IN SOCKET ****/,response_buffer,strlen(response_buffer),0); // send resource if requested, under what condition will the server send an // entity body?
if (/** FILL IN CONDITION **/) Send_Resource(connection_socket, new_request.URI); else { // don't need to send resource. end HTTP headers send(/*** FILL IN SOCKET ***/, " ", strlen(" "), 0); } /***** END PART B ****/
/* child's work is done, close remaining descriptors and exit */
if ( close(connection_socket) < 0) { fprintf(stderr, "closing connected socket failed"); exit(EXIT_FAILURE); }
/* all done return to parent */ exit(EXIT_SUCCESS);
} /*----------END OF CHILD CODE----------------*/
/* back in parent process */ /* close parent's reference to connection socket, */ /* then back to top of loop waiting for next request */ if ( close(connection_socket) < 0) { fprintf(stderr, "closing connected socket failed"); exit(EXIT_FAILURE); }
/* if child exited, wait for resources to be released */ waitpid(-1, NULL, WNOHANG);
} // end while(true) }
#ifndef HELPERS_H #define HELPERS_H
#include
/*---------------------------------------------------------- * Function: bool Parse_HTTP_Request(int socket, struct http_request * request_values) * * Purpose: Reads HTTP request from a socket and fills in a data structure * with the request values (method and URI) * * Parameters: socket : the socket to read the request from * request_value : address of struct to write the values to * * Returns: true if successfull, false if request is not a valid HTTP request * *----------------------------------------------------------- */
extern bool Parse_HTTP_Request(int, struct http_request *);
/*---------------------------------------------------------- * Function: Is_Valid__Resource(char * URI) * * Purpose: Checks if URI is a valid resource * * Parameters: URI : the URI of the requested resource, both absolute * and relative URIs are accepted * * Returns: false : the URI does not refer to a resource on the server * true : the URI is available on the server * *----------------------------------------------------------- */
extern bool Is_Valid_Resource(char *);
/*---------------------------------------------------------- * Function: void Send_Resource(int socket, char * URI) * * Purpose: Sends the contents of the file referred to in URI on the socket * * Parameters: socket : the socket to send the content on * URI : the Universal Resource Locator, both absolute and * relative URIs are accepted * * Returns: void - errors will cause exit with error printed to stderr * *----------------------------------------------------------- */
extern void Send_Resource(int, char *);
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
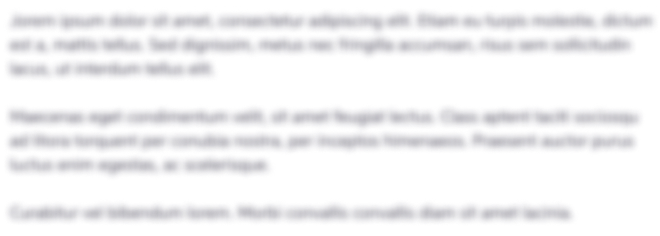
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started