Question
Business Case (Marketing, Project Manager, Senior Executives) There is a market for gaming software. One of the most popular card games is 21. We need
Business Case (Marketing, Project Manager, Senior Executives)
There is a market for gaming software. One of the most popular card games is "21". We need to write software that simulates this card game so players can play without actual cards. In addition to that, the gaming software can add features to the game "21" which are not available with physical cards.
Requirements (Marketing, Project Manager, Project Lead)
For simulating the card game "21", the following requirements must be implemented:
The sum of the cards must be as close to 21 as possible without going over.
All cards have integral (integer) values.
Introduce a high-definition mode to add granularity to the card draws. In this mode all cards have decimal (double floating point) values.
The card draws must be random within a certain range. These can be called "attack cards".
Introduce "dependent cards" which subtract from the card total to add complexity to the game. These cards are drawn at random within a certain range.
"Dependent cards" always have integral (integer) values.
Allow two players at a time.
The mode of the game has to be determined by the users before any objects are created.
For each game, the players can make a bet within a certain range.
Design (Project Lead, Senior Engineers)
Each Player
Each player has a name.
Each player has cash.
Each player has a list of dependent cards which are always integral regardless of the game mode.
Each player's dependent cards have a value between 1 and 5 inclusive.
Each player can be created with name set to an empty string, no dependent cards, and $1000.00 in cash.
Each player can be created with name passed by argument, no dependent cards, and $1000.00 in cash.
Each player can be created from another player.
Each player can be assigned all its data from another player through the = operator.
Each player provides accessor functions to set or get the name.
Each player provides a function to add dependent cards, deleting any existing list of dependent cards first.
Each player provides a function to add one dependent card to an existing list of dependent cards.
Each player provides a function to sum the dependent cards.
Each player provides functions to get cash and to add cash (could be negative).
Each player cleans itself up before destruction.
The Players
The players override the ==, < and > operators to see respectively if they are equal, if the first player has less points than the second, or if the first player has more points than the second.
The players override the << and >> to respectively add cash or remove cash from a player.
The Youth Player
In addition to those qualities for a player, the youth player has the following:
The youth player has a list of attack cards which can be integral (regular mode) or decimal (high definition mode).
The youth player's attack cards have a value between 1 and 10 inclusive.
The youth player's high definition attack cards will have values to three decimal places.
The youth player can be created with no attack cards.
The youth player can be created with a name passed by argument and no attack cards.
The youth player can be created from another youth player.
The youth player can be assigned all its data from another youth player through the = operator.
The youth player provides a function to add attack cards, deleting any existing list of attack cards first.
The youth player provides a function to add one attack card to an existing list of attack cards.
The youth player provides a function to sum the attack cards.
The youth player provides a function to sum all cards (sum the attack cards minus sum the dependent cards).
The youth player cleans itself up before destruction.
The Adult Player
The adult player has a list of attack cards which can be integral (regular mode) or decimal (high definition mode).
The adult player's attack cards have a value between 1 and 15 inclusive.
The adult player's high definition attack cards will have values to three decimal places.
The adult player can be created with no attack cards.
The adult player can be created with a name passed by argument and no attack cards.
The adult player can be created from another adult player.
The adult player can be assigned all its data from another adult player through the = operator.
The adult player provides a function to add attack cards, deleting any existing list of attack cards first.
The adult player provides a function to add one attack card to an existing list of attack cards.
The adult player provides a function to sum the attack cards.
The adult player provides a function to sum all cards (sum the attack cards minus sum the dependent cards).
The adult player cleans itself up before destruction.
The Adapter
The adapter must expose a non-templated interface to the main function.
The adapter must ask the user for the game-mode (low definition/high definition) and then create the players for the card game based on the mode.
The adapter then runs the game.
Implementation (Junior and Senior Engineers)
The Card Game "21"
As an engineer on this project, you have to implement the code for the card game "21".
Assume the players are known at compile-time: create and implement the classes and class relationships between the players.
Assume the players are known at run-time: create and implement the adapter for the game mode.
A card game has been created for you, with the assumption that the mode is known at compile-time. For this assignment, you will have to implement code where the mode is determined at run-time, instead of at compile time. This means you will have to implement a version of the adapter pattern to adapt a template-less interface to templated code.
Testing and Rework (Junior and Senior Engineers, Product Support)
Be sure to test your code for regular mode and high definition mode.
A correctly running card "21" program in high definition mode might look something like:
Would you like to play in low definition mode? [Y/N] n You will be playing in high definition mode. How much do you both want to bet? (min$10, max $300): $300 Noah, how many dependent cards to you want? 3 Noah, how many attack cards to you want? 4 Noah, the sum of your cards is 16.871 Do you want to add a dependent card? [Y,N]n Noah, the sum of your cards is 16.871 Do you want to add an attack card? [Y,N]n Noah, the sum of your cards is 16.871 Kenneth, how many dependent cards to you want? 4 Kenneth, how many attack cards to you want? 3 Kenneth, the sum of your cards is 13.253 Do you want to add a dependent card? [Y,N]n Kenneth, the sum of your cards is 13.253 Do you want to add an attack card? [Y,N]y Kenneth, the sum of your cards is 24.214 Noah has 16.871 points. Kenneth has 24.214 points. Kenneth has a sum of 24.214, which is over the limit. Kenneth has been disqualified for going over the limit. Noah has won. Noah has $1300. Kenneth has $700. How much do you both want to bet? (min$10, max $300): $300 Noah, how many dependent cards to you want? 2 Noah, how many attack cards to you want? 5 Noah, the sum of your cards is 21.2 Do you want to add a dependent card? [Y,N]y Noah, the sum of your cards is 18.2 Do you want to add an attack card? [Y,N]n Noah, the sum of your cards is 18.2 Kenneth, how many dependent cards to you want? 3 Kenneth, how many attack cards to you want? 3 Kenneth, the sum of your cards is 3.855 Do you want to add a dependent card? [Y,N]n Kenneth, the sum of your cards is 3.855 Do you want to add an attack card? [Y,N]y Kenneth, the sum of your cards is 9.75 Noah has 18.2 points. Kenneth has 9.75 points. Noah has won! Noah has $1600. Kenneth has $400. How much do you both want to bet? (min$10, max $300): $300 Noah, how many dependent cards to you want? 5 Noah, how many attack cards to you want? 3 Noah, the sum of your cards is 8.878 Do you want to add a dependent card? [Y,N]y Noah, the sum of your cards is 7.878 Do you want to add an attack card? [Y,N]y Noah, the sum of your cards is 18.772 Kenneth, how many dependent cards to you want? 2 Kenneth, how many attack cards to you want? 3 Kenneth, the sum of your cards is 1.328 Do you want to add a dependent card? [Y,N]n Kenneth, the sum of your cards is 1.328 Do you want to add an attack card? [Y,N]y Kenneth, the sum of your cards is 6.992 Noah has 18.772 points. Kenneth has 6.992 points. Noah has won! Noah has $1900. Kenneth has $100. How much do you both want to bet? (min$10, max $300): $300 Noah, how many dependent cards to you want? 4 Noah, how many attack cards to you want? 3 Noah, the sum of your cards is 6.853 Do you want to add a dependent card? [Y,N]n Noah, the sum of your cards is 6.853 Do you want to add an attack card? [Y,N]y Noah, the sum of your cards is 10.61 Kenneth, how many dependent cards to you want? 3 Kenneth, how many attack cards to you want? 3 Kenneth, the sum of your cards is 14.048 Do you want to add a dependent card? [Y,N]n Kenneth, the sum of your cards is 14.048 Do you want to add an attack card? [Y,N]n Kenneth, the sum of your cards is 14.048 Noah has 10.61 points. Kenneth has 14.048 points. Kenneth has won! Noah has $1600. Kenneth has $400. How much do you both want to bet? (min$10, max $300): $300 Noah, how many dependent cards to you want? 4 Noah, how many attack cards to you want? 5 Noah, the sum of your cards is 25.64 Do you want to add a dependent card? [Y,N]y Noah, the sum of your cards is 21.64 Do you want to add an attack card? [Y,N]n Noah, the sum of your cards is 21.64 Kenneth, how many dependent cards to you want? 3 Kenneth, how many attack cards to you want? 6 Kenneth, the sum of your cards is 28.949 Do you want to add a dependent card? [Y,N]y Kenneth, the sum of your cards is 24.949 Do you want to add an attack card? [Y,N]n Kenneth, the sum of your cards is 24.949 Noah has 21.64 points. Kenneth has 24.949 points. Noah has a sum of 21.64, which is over the limit. Kenneth has a sum of 24.949, which is over the limit. Both players are over the limit and have been disqualified. Noah has $1600. Kenneth has $400. How much do you both want to bet? (min$10, max $300): $300 Noah, how many dependent cards to you want? 3 Noah, how many attack cards to you want? 4 Noah, the sum of your cards is 15.516 Do you want to add a dependent card? [Y,N]n Noah, the sum of your cards is 15.516 Do you want to add an attack card? [Y,N]n Noah, the sum of your cards is 15.516 Kenneth, how many dependent cards to you want? 3 Kenneth, how many attack cards to you want? 2 Kenneth, the sum of your cards is -1.617 Do you want to add a dependent card? [Y,N]n Kenneth, the sum of your cards is -1.617 Do you want to add an attack card? [Y,N]y Kenneth, the sum of your cards is 9.009 Noah has 15.516 points. Kenneth has 9.009 points. Noah has won! Noah has $1900. Kenneth has $100. How much do you both want to bet? (min$10, max $300): $200 Noah, how many dependent cards to you want? 3 Noah, how many attack cards to you want? 4 Noah, the sum of your cards is 10.67 Do you want to add a dependent card? [Y,N]n Noah, the sum of your cards is 10.67 Do you want to add an attack card? [Y,N]y Noah, the sum of your cards is 16.503 Kenneth, how many dependent cards to you want? 3 Kenneth, how many attack cards to you want? 2 Kenneth, the sum of your cards is 9.634 Do you want to add a dependent card? [Y,N]n Kenneth, the sum of your cards is 9.634 Do you want to add an attack card? [Y,N]y Kenneth, the sum of your cards is 24.611 Noah has 16.503 points. Kenneth has 24.611 points. Kenneth has a sum of 24.611, which is over the limit. Kenneth has been disqualified for going over the limit. Noah has won. Player Kenneth is out of the game. Noah has $2100. Kenneth has $-100. The game is over. Noah has $2100.00 Kenneth has $-100.00
Step by Step Solution
There are 3 Steps involved in it
Step: 1
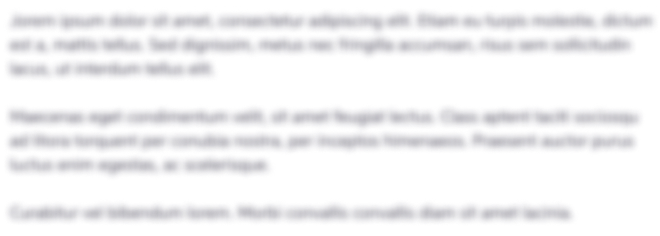
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started