Question
By hand. Sort the sequence 5, 6, 2, 9, 5, 1, 4, 1, 3 using quick sort, by using the code below. Please show steps,
By hand. Sort the sequence 5, 6, 2, 9, 5, 1, 4, 1, 3 using quick sort, by using the code below. Please show steps, thank you!
----------------------------------------------------------------------------------------------------
template
void quicksort( vector
//Use quick sort if we have at least four elements.
if( left + 3 <= right ){
const Comparable & pivot = median3( a, left, right );
//Begin partitioning
int i = left, j = right - 1;
for( ; ; ){
while( a[ ++i ] < pivot ) { }
while( pivot < a[ --j ] ) { }
if( i < j )
std::swap( a[ i ], a[ j ] );
else
break;
}
std::swap( a[ i ], a[ right - 1 ] ); // Restore pivot
quicksort( a, left, i - 1 ); // Sort small elements
quicksort( a, i + 1, right ); // Sort large elements
}
else // Do an insertion sort on the subarray
insertionSort( a, left, right );
}
template
const Comparable & median3( vector
int center = ( left + right ) / 2;
if( a[ center ] < a[ left ] )
std::swap( a[ left ], a[ center ] );
if( a[ right ] < a[ left ] )
std::swap( a[ left ], a[ right ] );
if( a[ right ] < a[ center ] )
std::swap( a[ center ], a[ right ] );
// Place pivot at position right - 1
std::swap( a[ center ], a[ right - 1 ] );
return a[ right - 1 ];
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
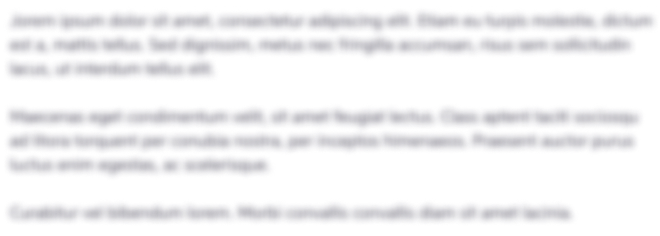
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started