Question
By using Python The lab consists of several tasks and will demonstrate the following concepts: Reading from a file Working with lists Using dot notation
By using Python
The lab consists of several tasks and will demonstrate the following concepts:
- Reading from a file
- Working with lists
- Using dot notation with strings
Part A (A Simple Shopping List)
The first task in this lab is to create a function, shopping_list() that reads a shopping list from a file, sorts the list, then prints the sorted list. The file is named lab5List.txt and should be placed in the same directory as your Python script. The list contains several items, one per line. The readlines() method reads all lines from a file and returns a list.
lab5List.txt :
A sample execution is shown, but the full list is not shown here:
>>> shopping_list() ['bread ', 'butter ', 'cheese ', 'chicken ']
Extra Challenge (not required but good experience)
Note that each item retains the newline character. What if we wanted to accomplish the same task, but without having the newline character on each line?
Repeat the first task, but programmatically remove the newline characters (one way: convert the list to a string and replace the new line character; another way: replace in each item in list and build a new list using .append()) from each item and print both versions:
>>> shopping_list() ['bread ', 'butter ', 'cheese ', 'chicken '] bread butter cheese chicken
Part B (Cat/Dog)
This task involves creating a function, cat_dog(cd_string), that accepts a string parameter containing only combinations of the letters 'C', 'D', 'c', and 'd' (representing 'cats' and 'dogs', respectively) and does the following two sub-tasks:
- counts the number of 'cats' and the number of 'dogs' in the string using the .count()method and then prints out the count of each
- makes use of the .replace() method to replace all the 'c' and 'C' characters with the digit 0, and all the 'd' and 'D' characters with the digit 1, thus creating a binary string. You should then print both strings for comparison
Two sample executions are shown below.
>>> cat_dog("ddcDcDCdDc") There are 4 cats and 6 dogs ddcDcDCdDc 1101010110
>>> cat_dog("CCDcdcDDcddcDC") There are 7 cats and 7 dogs CCDcdcDDcddcDC 00101011011010
Hints
- Remember that .upper() and .lower() are often useful when dealing with mixed case letters
- Most string methods return an altered copy of the string and don't modify the original
Part C (Calculating a Final Grade)
In this exercise, you must create a function, calc_final(), that can calculate and print the final mark for a student. The final mark is calculated from of several pieces of work, each with different base (i.e., the maximum possible mark) and weights, as shown in the table below. The final mark is taken as the sum of mark/base*weight for all the pieces of work. You should create 3 lists (one for the marks, one for the base marks, and one for the weights), then calculate and print the final mark using the three lists. Using the values in the table, the final mark is 71.5%. You may want to consider calculating the grade by hand before writing your function.
Mark | Base | Weight |
17 | 20 | 10 |
4 | 5 | 10 |
6.5 | 10 | 10 |
12 | 15 | 10 |
35 | 50 | 15 |
24 | 40 | 15 |
70 | 100 | 30 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
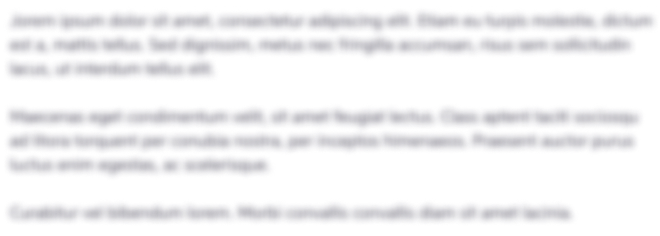
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started