Question
C++ 1) Output the title and the year released for each movie. 2) Output the lead actor's name next to the title and year release
C++
1) Output the title and the year released for each movie.
2) Output the lead actor's name next to the title and year release above. (Important hint, a movie may not have a lead actor, if it does not, output "no lead actor").
3) Write code to calculate and output the total number of actors who are no longer alive.
4) Write code to determine which actor has the most number of characters in their name? Hint, string has the size() or length() method that indicates the number of characters.
Notes:
numActors will contain the number of actors in the actors array.
leadActor and actors will be NULL if no actors are in the Movie. Also, numActors will be zero in this case.
#include
//Function to get Movies Movie** getShows(int &numMovies) { Movie **ms = new Movie*[6]; ms[0] = new Movie; ms[0]->actors = new Actor[2]; ms[0]->actors[0].actName = "Burt"; ms[0]->actors[0].gender = 'm'; ms[0]->actors[0].isAlive = false; ms[0]->leadActor = ms[0]->actors; ms[0]->actors[1].actName = "Lonie"; ms[0]->actors[1].gender = 'f'; ms[0]->actors[1].isAlive = true; ms[0]->numActors = 2; ms[0]->title = "Cannonball"; ms[0]->yrReleased = 1981; ms[1] = new Movie; ms[1]->actors = new Actor[4]; ms[1]->actors[0].actName = "Mark H."; ms[1]->actors[0].gender = 'm'; ms[1]->actors[0].isAlive = true; ms[1]->actors[1].actName = "Carrie F."; ms[1]->actors[1].gender = 'f'; ms[1]->actors[1].isAlive = false; ms[1]->actors[2].actName = "Harrison F."; ms[1]->actors[2].gender = 'm'; ms[1]->actors[2].isAlive = true; ms[1]->actors[3].actName = "Alec G."; ms[1]->actors[3].gender = 'm'; ms[1]->actors[3].isAlive = false; ms[1]->numActors = 4; ms[1]->leadActor = ms[1]->actors + 2; ms[1]->title = "Star Wars"; ms[1]->yrReleased = 1976; ms[2] = new Movie; ms[2]->actors = new Actor[1]; ms[2]->actors[0].actName = "Spoiled Actor"; ms[2]->actors[0].gender = 'm'; ms[2]->actors[0].isAlive = true; ms[2]->numActors = 1; ms[2]->leadActor = ms[2]->actors; ms[2]->title = "A Troubled Man"; ms[2]->yrReleased = 2014; ms[3] = new Movie; ms[3]->actors = NULL; ms[3]->numActors = 0; ms[3]->leadActor = NULL; ms[3]->title = "Secret Life of Plants"; ms[3]->yrReleased = 1974; ms[4] = new Movie; ms[4]->actors = new Actor[3]; ms[4]->actors[0].actName = "Roger Rabbit"; ms[4]->actors[0].gender = 'm'; ms[4]->actors[0].isAlive = true; ms[4]->actors[1].actName = "Hoskins"; ms[4]->actors[1].gender = 'm'; ms[4]->actors[1].isAlive = true; ms[4]->actors[2].actName = "Kathleen T."; ms[4]->actors[2].gender = 'f'; ms[4]->actors[2].isAlive = true; ms[4]->numActors = 3; ms[4]->leadActor = ms[4]->actors + 1; ms[4]->title = "Who Framed the Rodent?"; ms[4]->yrReleased = 1984; ms[5] = new Movie; ms[5]->actors = new Actor[300]; ms[5]->actors[0].actName = "Charles H."; ms[5]->actors[0].gender = 'm'; ms[5]->actors[0].isAlive = true; for (int ii = 1; ii<300; ii++) { char *tn = new char[15]; sprintf_s(tn, 14, "Extra# %d", ii); ms[5]->actors[ii].actName = tn; ms[5]->actors[ii].gender = ((ii < 250 || ii % 3 != 0) ? 'm' : 'f'); ms[5]->actors[ii].isAlive = (ii < 287 || ii % 2 == 0); } ms[5]->numActors = 300; ms[5]->leadActor = ms[5]->actors; ms[5]->title = "Ben Hur"; ms[5]->yrReleased = 1965; numMovies = 6; return ms; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
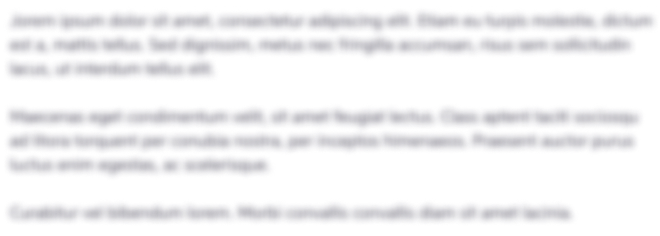
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started