Question
C++ Assuming you can read the input of a list of 1D, 2D and 3D points from std::cin, for example, x=8.6 x=0 y=0 x=6 x=1
C++
Assuming you can read the input of a list of 1D, 2D and 3D points from std::cin, for example,
x=8.6 x=0 y=0 x=6 x=1 y=2.4 z=3 x=3 y=4 x=7 x=5.2 y=5 z=5
where each line denotes one point.
Could you count how many 1D, 2D, and 3D points, respectively? Could you come up with a solution using Polymorphism?
Assuming you have the base class called Point, and the derived class Point1D, Point2D, and Point3D, where Point1D derives from Point, Point2D derives from Point1D, Point3D derives from Point2D.
In the Point class, you need to define a virtual function virtual int getDimension() const = 0 which returns the dimension.
In the class Point1D, Point2D, and Point3D you need to overwrite the function getDimension() to return the correct dimension. Maybe you also need to define the x y z coordinates and other necessary functions.
For the example input above, the following program:
int main()
{
Point* points_1[7];
read(points_1,7);
print(points_1,7);
return 0;
}
Will generate the following output:
#1D = 3, #2D = 2, #3D = 3
After modifying the classes stated before, you need to write the read and print functions defined in the functions.h file.
NOTES:
- Your program will use input redirection (you do not need to open the input file).
- Include the .h files when needed.
- Use inheritance when needed.
- Use abstract classes when needed.
- Use virtual functions when needed.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
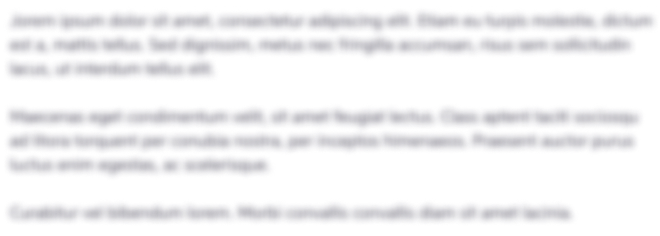
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started