Question
C# BINGO PROGRAM It must have these four classes, use buttons and it must be a visual c# windows forms application. The internalcardclass should check
C# BINGO PROGRAM
It must have these four classes, use buttons and it must be a visual c# windows forms application. The internalcardclass should check for Bingo by comparing two arrays and seeing if they both match.
InternalCardClass: Responsible for all actions related to the creation and updating of the Internal Representation of a single Bingo Card as well as checking for a Bingo
SelectedNumbersList class: Responsible for all actions related to the keeping track of and checking for Bingo card numbers that have already been used
RandomNumberGenerator: Encapsulates all data and methods associated with the generation of unique Random Numbers
please make sure the code is neat and easy to understand. please make sure to specify where the classes begin and end.
MainForm Class
___________________________________________________________________________________________________
The Four needed Classes:
The main form class this is essentially the driver program for the project; it contains all
the code behind for the form we will design, including the buttons on the Bingo card
An Internal Card class (InternalCardType2DimArray)
A Random Number Generator class (RNGType)
A Selected Numbers List class (SelectedNumbersListType).
_________________________________________________________________
Here is some given code I received that should help.
--RNG Class and Globals Class--
// Random Number Generator Class -- // Encapsulates all data and methods associated with the generation of Random Numbers
public class RNGType { // private SelectedNumbersListType SelectedNumbersListObj = new SelectedNumbersListType(); private Random RandomObj; // Type random object // private int nextRandomValue; // Next random value
// Constructor -- Creates and seeds a type random object public RNGType() { RandomObj = new Random(); // Creates and seeds (using current tithis) random object } // end RNGType
// Get Random Value // Gets next random value and ensures it is in the correct range for the column // involved // Returns a valid random number public int getRandomValue(char columnHeader) { int r; // Random number generated
switch (columnHeader) { case 'B': r = getNextUniqueRandomValue(1, 15); if (r 15) { MessageBox.Show("Program Error! Selected random number out of range 1-15", "Click to terminate program.", MessageBoxButtons.OK); return -1; } // end if break; case 'I': r = getNextUniqueRandomValue(16, 30); if (r 30) { MessageBox.Show("Program Error! Selected random number out of range 16-30", "Click to terminate program.", MessageBoxButtons.OK); return -1; } // end if break; case 'N': r = getNextUniqueRandomValue(31, 45); if (r 45) { MessageBox.Show("Program Error! Selected random number out of range 31-45", "Click to terminate program.", MessageBoxButtons.OK); return -1; } // end if break; case 'G': r = getNextUniqueRandomValue(46, 60); if (r 60) { MessageBox.Show("Program Error! Selected random number out of range 46-60", "Click to terminate program.", MessageBoxButtons.OK); return -1; } // end if break; case 'O': r = getNextUniqueRandomValue(61, 75); if (r 75) { MessageBox.Show("Program Error! Selected random number out of range 61-75", "Click to terminate program.", MessageBoxButtons.OK); return -1; } // end if break; default: MessageBox.Show("Program Error! Selected Letter no B I N G or O!", "Click to terminate program.", MessageBoxButtons.OK); return -1; } // end switch return r; } // end getRandomValue
// Creates the next set of random values (from 1 to diceToBeRolled values) public int getNextUniqueRandomValue(int minVal, int maxVal) { Boolean isUnique; int rn = 0; // random number obtained // Assume number is not unique isUnique = false;
while (isUnique == false) { rn = RandomObj.Next(minVal, maxVal); if (!Globals.selectedNumbersListObj.isNumberUsed(rn)) { isUnique = true; Globals.selectedNumbersListObj.setUsedNumber(rn); } // end if } // end while return rn; } // end getNextRandomValue } // end RNGType Class
// Globals Class // Used only to instantiate the Selected Numbers List Object which is used in two places
public class Globals { public static SelectedNumbersListType selectedNumbersListObj = new SelectedNumbersListType(); } // end Globals class ____________________________________________________________________________________________________
---Some other code that should generate the player visual layout of the bingo board---
private const int BINGOCARDSIZE = 5; private const int NUMBERSPERCOLUMN = 15; private const int MAXBINGONUMBER = 75;
private Button[,] newButton = new Button[BINGOCARDSIZE, BINGOCARDSIZE];
// Total width and height of a card cell int cardCellWidth = 75; int cardCellHeight = 75; int barWidth = 6; // Width or thickness of horizontal and vertical bars int xcardUpperLeft = 45; int ycardUpperLeft = 45; int padding = 20;
// Creates the Bingo Card for Play private void createCard() { // Dynamically Creates 25 buttons on a Bingo Board // Written by Bill Hall with Joe Jupin and FLF // This should be enough help for all of you to adapt this to your own needs // Create and Add the buttons to the form
Size size = new Size(75, 75); // if (gameCount > 0) size = new Size(40,40); Point loc = new Point(0, 0); int topMargin = 60;
int x; int y;
// Draw Column indexes y = 0; DrawColumnLabels();
x = xcardUpperLeft; y = ycardUpperLeft;
// Draw top line for card drawHorizBar(x, y, BINGOCARDSIZE); y = y + barWidth;
// The board is treated like a 5x5 array drawVertBar(x, y); for (int row = 0; row
if (row == BINGOCARDSIZE / 2 && col == BINGOCARDSIZE / 2) { newButton[row, col].Font = new Font("Arial", 10, FontStyle.Bold); newButton[row, col].Text = "Free Space"; newButton[row, col].BackColor = System.Drawing.Color.Orange; } else { newButton[row, col].Font = new Font("Arial", 24, FontStyle.Bold); newButton[row, col].Text = RNGObj.getRandomValue(bingoLetters[col]).ToString(); } // end if newButton[row, col].Enabled = true; newButton[row, col].Name = "btn" + row.ToString() + col.ToString();
// Associates the same event handler with each of the buttons generated newButton[row, col].Click += new EventHandler(Button_Click);
// Add button to the form pnlCard.Controls.Add(newButton[row, col]);
// Draw vertical delimiter x += cardCellWidth+padding; if(row == 0) drawVertBar(x-5, y); } // end for col // One row now complete
// Draw bottom square delimiter if square complete x = xcardUpperLeft-20; y = y + cardCellHeight+padding; drawHorizBar(x+25, y-10, BINGOCARDSIZE-10); } // end for row
// Draw column indices at bottom of card y += barWidth - 1; DrawColumnLabels(); Globals.selectedNumbersListObj.reset(); } // end createBoard
// Draws column indexes at top and bottom of card private void DrawColumnLabels() { Label lblColID = new Label(); lblColID.Font = new System.Drawing.Font("Microsoft Sans Serif", (float)24.0, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, 0); lblColID.ForeColor = System.Drawing.Color.Orange; lblColID.Location = new System.Drawing.Point(cardCellWidth, 0); lblColID.Name = "lblColIDBINGO"; lblColID.Size = new System.Drawing.Size(488, 32); lblColID.TabIndex = 0; lblColID.Text = "B I N G O"; pnlCard.Controls.Add(lblColID); lblColID.Visible = true; lblColID.CreateControl(); lblColID.Show(); } // end drawColumnLabels
// Draw the dark horizontal bar private void drawHorizBar(int x, int y, int cardSize) { int currentx; currentx = x;
Label lblHorizBar = new Label(); lblHorizBar.BackColor = System.Drawing.SystemColors.ControlText; lblHorizBar.Location = new System.Drawing.Point(currentx, y); lblHorizBar.Name = "lblHorizBar"; lblHorizBar.Size = new System.Drawing.Size((cardCellWidth + padding-1) * BINGOCARDSIZE, barWidth); lblHorizBar.TabIndex = 20; pnlCard.Controls.Add(lblHorizBar); lblHorizBar.Visible = true; lblHorizBar.CreateControl(); lblHorizBar.Show(); currentx = currentx + cardCellWidth; } // end drawHorizBar
// Draw dark vertical bar private void drawVertBar(int x, int y) { Label lblVertBar = new Label(); lblVertBar.BackColor = System.Drawing.SystemColors.ControlText; lblVertBar.Location = new System.Drawing.Point(x, y); lblVertBar.Name = "lblVertBar" + x.ToString(); lblVertBar.Size = new System.Drawing.Size(barWidth, (cardCellHeight + padding -1) * BINGOCARDSIZE); lblVertBar.TabIndex = 19; pnlCard.Controls.Add(lblVertBar); lblVertBar.Visible = true; lblVertBar.CreateControl(); lblVertBar.Show(); } // end drawVertBar
// This is the handler for all Bingo Card Buttons // It uses sender argument to determine which Bingo Card button was selected // The argument is of type object and must be converted to type button in // order to change its properties private void Button_Click(object sender, EventArgs e) { int bingoCount2D; int bingoCountWO2D;int selectedNumber; // number randomly selected
int rowID = convertCharToInt(((Button)sender).Name[3]); int colID = convertCharToInt(((Button)sender).Name[4]); MessageBox.Show("Cell[" + rowID + "," + colID + "] has been selected!"); int cellID = rowID * 3 + colID;
// Double check that clicked on button value matches called value selectedNumber = Convert.ToInt32(newButton[rowID, colID].Text); if (selectedNumber == nextCalledNumber) { newButton[rowID, colID].BackColor = System.Drawing.Color.Orange; internalCardRep2DArray.recordCalledNumber(rowID, colID); internalCardRepWO2DArray.recordCalledNumber(rowID, colID); Globals.selectedNumbersListObj.setUsedNumber(selectedNumber);
// Check for winner // Go here if player found the number called in his or her card // Check for winner for either internal representation bingoCount2D = internalCardRep2DArray.isWinner(rowID, colID); bingoCountWO2D = internalCardRepWO2DArray.isWinner(rowID, colID); if ((bingoCount2D > 0) && (bingoCountWO2D > 0)) { MessageBox.Show("You are a Winner!!", "Winner Found! " + "Bingos count = " + (bingoCount2D + bingoCountWO2D) / 2 + ". Game over!"); Close(); } // end inner if
playTheGame(); } else { MessageBox.Show("Called number does not match the one in the box you selected." + "Try again!", "Numbers Do Not Match"); } // end outer if } // end button clickhandler
Step by Step Solution
There are 3 Steps involved in it
Step: 1
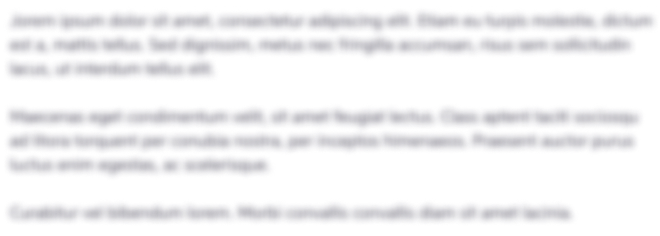
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started