Question
C++ C++ C++ C++ C++ Need help to finish. Please provide comment lines to help me out and a picture of the output so that
C++ C++ C++ C++ C++
Need help to finish. Please provide comment lines to help me out and a picture of the output so that I can see that it properly functions.
/******************************************************** * Description: * Displays the top 3 most "abundant" (see writeup) * numbers in the input sequence. The input sequence * is assumed to be valid natural numbers separated * by spaces and ending with 0. * * ABSOLUTELY NO ARRAY or `string`/`vector`/etc. usage * is allowed!
* You may only declare: bool, int, or unsigned int * No library functions (beyond cin/cout) are allowed. * * You may NOT modify any of the given template code. * This ensures our automated checkers can parse your * output. Failure to follow this direction will lead * to a score of 0 on this part of the assignment.
Input/Output example: If we provide the input 18 15 12 48 32 36 0 your program should produce the following output:
Abundant number count: 4 Top 3 most abundant numbers:
48 : 76
36 : 55
18 : 21
********************************************************/
#include
int main() { // n1 is the number with the highest abundance, a1 // n2 is the number with the 2nd-highest abundance, a2 // n3 is the number with the 3rd-highest abundance, a3 unsigned int n1 = 0, n2 = 0, n3 = 0; int a1 = 0, a2 = 0, a3 = 0;
// num_abundant counts how many numbers in the input // sequence are abundant int num_abundant = 0;
cout << "Enter a sequence of natural numbers, separated by spaces, "; cout << "and ending with 0."<< endl;
/*====================*/ /* Start of your code */
int val; // val will be used for user input numbers they choose int val_ab = 0; // will find which numbers are abundant cin >> val; while (val != 0) { for(int i = 1; i <= val/2; i++) { if(val % i == 0) { val_ab += i; } } } // Checking if abundant if (val_ab > val) { num_abundant++; } // Ordering top three abundant numbers if (val_ab > a1) { a3 = a2; n3 = n2; a2 = a1; n2 = n1; a1 = val_ab; n1 = val; } else if (val_ab > a2) { a3 = a2; n3 = n2; a2 = val_ab; n2 = val; } else if (val_ab > a3) { a3 = val_ab; n3 = val; } // Resetting values cin >> val; val_ab = 0; } /* End of your code */ /*==================*/ cout << "Abundant number count: " << num_abundant << endl; cout << "Top 3 most abundant numbers: " << endl; cout << n1 << " : " << a1 << endl; cout << n2 << " : " << a2 << endl; cout << n3 << " : " << a3 << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
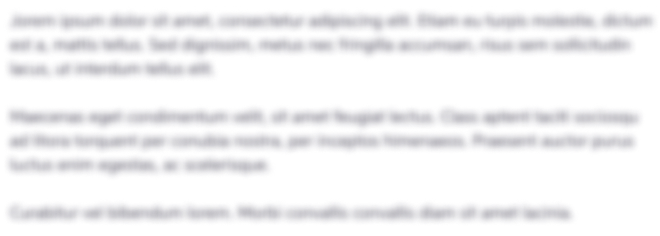
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started