Question
C++ : Can someone please explain what i am doing wrong here (in the top_three_trend function)? Any help would be greatly appreciated. Heres what I
C++ : Can someone please explain what i am doing wrong here (in the top_three_trend function)? Any help would be greatly appreciated. Heres what I have below:
// Fills the provided vector with the 3 most-tweeted hashtags,
// in order from most-tweeted to least-tweeted.
//
// If there are fewer than 3 hashtags, then the vector is filled
// with all hashtags (in most-tweeted to least-tweeted order).
//
// Must run in O(n) time.
void Trendtracker::top_three_trends(vector
// clear (max 3 each time)
T.clear();
// hold copy of original list
vector
copy = E;
// sort copy
int largest = 0; // index of largest at start
while (largest < copy.size()){
for (int i = 0; i < copy.size(); i++){
if (copy[largest].pop < copy[i].pop){
// swap to first index
Entry temp;
temp = copy[largest];
copy[largest] = copy[i];
copy[i] = temp;
}
}
largest++; // increment index of largest
}
// find top 3 in copy
//
int front = 0; // always first index
int back = copy.size() - 1; // initialized to last index
if (copy.size() >= 3){
back = front + 2;
for (int i = 0; i <= back; i++){
T.push_back(copy[i].hashtag);
}
} else {
for (int i = 0; i < copy.size(); i++){
T.push_back(copy[i].hashtag);
}
}
}
------------------------------------------------------------------------------------------------------------------------------------------------------------------------
// Header file
#ifndef TRENDTRACKER_H
#define TRENDTRACKER_H
#include
#include
using namespace std;
class Trendtracker
{
// For the mandatory running times below:
// n is the number of hashtags in the Trendtracker.
public:
// Creates a new Trendtracker tracking no hashtags.
//
// Must run in O(1) time.
Trendtracker();
// Inserts a hashtag (tweeted 0 times) into the Trendtracker.
// If the hashtag already is in Trendtracker, does nothing.
//
// Must run in O(n) time.
void insert(string ht);
// Return the number of hashtags in the Trendtracker.
//
// Must run in O(1) time.
int size();
// Adds 1 to the total number times a hashtag has been tweeted.
// If the hashtag does not exist in TrendTracker, does nothing.
//
// Must run in O(n) time.
void tweeted(string ht);
// Returns the number of times a hashtag has been tweeted.
// If the hashtag does not exist in Trendtracker, returns -1.
//
// Must run in O(n) time.
int popularity(string name);
// Returns a most-tweeted hashtag.
// If the Trendtracker has no hashtags, returns "".
//
// Must run in O(n) time.
string top_trend();
// Fills the provided vector with the 3 most-tweeted hashtags,
// in order from most-tweeted to least-tweeted.
//
// If there are fewer than 3 hashtags, then the vector is filled
// with all hashtags (in most-tweeted to least-tweeted order).
//
// Must run in O(n) time.
void top_three_trends(vector
private:
// A simple class representing a hashtag and
// the number of times it has been tweeted.
class Entry
{
public:
string hashtag;
int pop;
};
// Entries containing each hashtag and its popularity.
vector
};
#endif
--------------------------------------------------------------------------------------------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
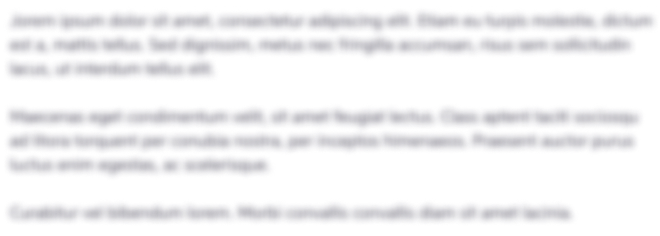
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started