Question
C++ Cash Register Using parallel Arrays Can someone help fix code for output file (sales)?? --------------------------------------------------- Instead of opening and closing the input file for
C++ Cash Register Using parallel Arrays
Can someone help fix code for output file (sales)??
---------------------------------------------------
Instead of opening and closing the input file for each requested item, open the stock.txt file, read all the values for the 9 stock items into three parallel arrays, then close the input file. When the use gives you a stock number you should search the arrays for the match and the corresponding description and price.
I am providing for you a file called Stock.txt that contains information regarding the 9 different stock items carried by FUNNY STUFF RETAIL INC. The file is a repeating sequence of three records such that the first record represents an item code number, the second record represents the description of each item and the third record contains the current selling price. Then the sequence repeats. Your program is to simulate the check out register allowing the check out clerk to input item codes to obtain the current selling prices and descriptions. If an incorrect code is entered, the clerk should be notified by an appropriate message on the screen. If the item is found, the clerk will enter the number of items with that description and price and continue to the next item. When all items have been entered the clerk terminates the transaction by entering an item code of 0 (zero). The program will build a sales slip called Sales.txt enumerating the item code, description, unit price, quantity purchased, and total extended price. The bill will be subtotaled and an 8% sales tax added for a final total. Amount tendered by the customer and change given will be finally added to the invoice. This invoice should be written out to an output file called Sales.txt. The final total and change should appear on the screen as well. Your output file should line up all columns and output formatted for dollars and cents on money amounts, using setprecision, showpoint, and fixed. Make sure you format your output to the screen as well.
----------------------------------------
stock.txt:
1234 Stockings 12.39 2865 Pajamas 17.99 4020 Dress 23.00 3640 Sweater 20.50 5109 Shorts 56.99 4930 TV 88.20 6600 ToothBrush 94.55 5020 AluminumPan 16.79 2336 Pencils 4.55
code:
#include
#include
#include
using namespace std;
int main()
{
// Declare Files
ifstream inputfile;
ofstream outputfile;
cout << fixed << showpoint << setprecision(2);
outputfile << fixed << showpoint << setprecision(2);
// Declare Output Variables;
int fileItemNum[9];
double filePrice[9];
char fileDescription[9][50];
// Declare Variables
double tax;
double change;
double payment;
double subtotal=0;
double price;
double itemInfo;
int itemNum;
int quantity;
char test[50];
char found = 'n';
double total = 0;
int counter = 0;
int count=0;
inputfile.open ("Stock.txt");
int fileLength = 0;
//while (inputfile >> test)
//{
// fileLength++;
inputfile.close();
inputfile.open("Stock.txt");
while (count < 9 && inputfile >> fileItemNum [count] && inputfile >> fileDescription [count] && inputfile >> filePrice [count])
count++;
inputfile.close();
cout << "Enter an item number. Type 0 to finish." << endl;
cin >> itemNum;
while (itemNum !=0)
{
found = 0;
for (counter =0;counter <9; counter++ )
{
if (itemNum == fileItemNum[counter])
{
found = 'y';
cout << fileItemNum [counter]<< " " << fileDescription[counter]<< " " << filePrice[counter]<< endl;
cout << "How many do you want to buy?" << endl;
cin >> quantity;
while (quantity <=0)
{
cout << "Invaild Entry! Try again." << endl;
cin >> quantity;
}
subtotal = subtotal+(quantity*filePrice[counter]);
cout << "Subtotal: " << subtotal << endl;
}
}
if (found == 'n')
{
cout << "Item could not be found. Try again." << endl;
}
cout << "Enter an item number. Type 0 to finish." << endl;
cin >> itemNum;
outputfile.open ("Sales.txt");
outputfile << "-----------------" << endl;
outputfile << "Item Number: " << fileItemNum << endl;
outputfile << "Description: " << fileDescription << endl;
outputfile << "Price: $" << filePrice << endl;
outputfile << "Quantity: " << quantity << endl;
outputfile << "Subtotal: $" << subtotal << endl;
//outputfile.close();
}
//outputfile.open ("Sales.txt");
tax = (subtotal*.08);
cout << "Tax: $" << tax << endl;
total=subtotal+tax;
cout << "Total: $" << total << endl;
cout << "Enter customer payment." << endl;
cin >> payment;
while (payment < total)
{
cout << "More money is needed. Try again." << endl;
cin >> payment;
}
change = payment-total;
cout << "Change: $" << change << endl;
//old outputfile quantity location
outputfile << "-----------------" << endl;
outputfile << "Total: $" << total << endl;
outputfile << "Change: $" << change << endl;
outputfile.close ();
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
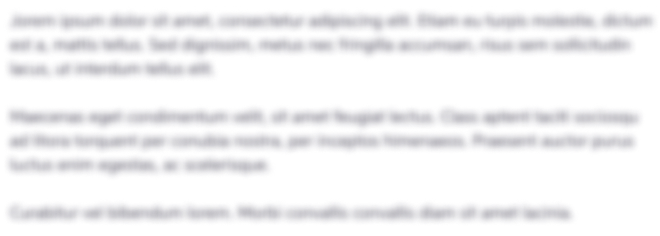
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started