Question
C++ Circular doubly linked list Write a program that manages songs playlists in media player applications. The program should allow typical media player functionalities such
C++
Circular doubly linked list
Write a program that manages songs playlists in media player applications. The program should allow typical media player functionalities such as playing the current song, previous song, next song, play the entire playlist, delete a song... To do that, the program creates a circular doubly linked list of Songs sorted by their names.
Each Song has two pointers to a Song, previous and next, and other private attributes as summarized below:
string name; // includes path
string artist; // artist name
string genre; // genre of song
double length; // length of song
Song * previous; // pointer to previous Song node
Song * next; // pointer to next Song node
The class SongPlayer has the following private attributes:
Song * head; // pointer to list head
Song * current; // pointer to current node being played. It is initialized to NULL // and is updated as new songs are played/deleted
SongPlayer has at least one constructor, one destructor, and the following member functions:
void addSong(string sName, string sArtist, string sGenre, double sLength)
This function creates a new Song and initializes its data to sName, sArtist, sGenre, and sLength. The function then inserts the new Song into the list while maintaining the list order. A message must be displayed whether the song was either successfully added to the list or not.
void playSong(string sName)
This function displays the details of the song whose name matches sName. Details include the number of the song in the playlist, the song name, the artist name, the genre and length. Make sure to include spaces when printing the song and artist names. You may assume that every new word starts with an uppercase. The number of the song is the number of the node. The function should also update the current pointer to point to this Song. If no such Song exists, an error message must be displayed.
void playCurrentSong()
This function displays the details of the current song, the song pointed to by the current pointer.
void playNextSong()
This function updates the current pointer to point to the next song in the list, if there is any, and then displays its details. If the current song is the last element in the list, the current pointer should be updated to point to the first song in the list and show the first song details.
void playPreviousSong()
This function updates the current pointer to point to the previous song in the list, if there is any, and then displays its details. If the current song is the first element in the list, the current pointer should be updated to point to the last song in the list.
void deleteSong(string sName)
This function searches the list for the song whose name matches sName and deletes it from the list. If the song to be deleted is the current song, the current pointer is updated to point to its previous Song or null if the list becomes empty.
void deleteCurrentSong()
This function deletes the current song and updates the current pointer to point to either its previous song or null if the list becomes empty.
void playAll (string sGenre)
This function prints the details of all songs of a specific genre sGenre. If no such songs exist, an error message must be displayed.
void playList()
This function traverses the list and prints the details of all songs in order up until the last song in the list.
void reversePlayList()
This function traverses the list and prints the details of all songs in reverse order (backwards).
void processTransactionFile()
This function reads a set of commands from the transaction file and executes the corresponding member functions.
After designing your Song and SongPlayer classes, you need to write the following in your main program:
int main()
{
SongPlayer player;
player.processTransactionFile( );
return 0;
}
A sample transaction file is shown below. You may use it to test your code: addSong C:/Users/Mayssaa/CS211/HW4/IDontWantToLiveForever TaylorSwift Wedding 4.17 addSong C:/Users/Mayssaa/CS211/HW4/CantStopTheFeeling JustinTimberlake Pop 4.46 addSong C:/Users/Mayssaa/CS211/HW4/BreathingUnderwater EmiliSande Wedding 3.51 addSong C:/Users/Mayssaa/CS211/HW4/SweetCaroline NeilDiamond Wedding 3.31 addSong C:/Users/Mayssaa/CS211/HW4/ThinkingOutLoud EdSheeran Wedding 4.56 addSong C:/Users/Mayssaa/CS211/HW4/TheWheelsOnTheBus LittleBabyBum Kids 2.17 addSong C:/Users/Mayssaa/CS211/HW4/IceCreamSong LittleBabyBum Kids 1.49 addSong C:/Users/Mayssaa/CS211/HW4/FrereJacques LesComptineDeGabriel Kids 1.33 addSong C:/Users/Mayssaa/CS211/HW4/HeadShoulderKneesAndToes ChuchuTV Kids 2.11 addSong C:/Users/Mayssaa/CS211/HW4/TakeTheATrain DukeEliington Jazz 3.14 addSong C:/Users/Mayssaa/CS211/HW4/TakeFive DaveBrubeck Jazz 5.30 addSong C:/Users/Mayssaa/CS211/HW4/FlyMeToTheMoon FrankSinatra Jazz 2.29 addSong C:/Users/Mayssaa/CS211/HW4/Perfect EdSheeran Pop 4.23 addSong C:/Users/Mayssaa/CS211/HW4/TooGoodAtGoodbyes SamSmith Pop 4.25 addSong C:/Users/Mayssaa/CS211/HW4/InCaseYouDidntKnow BrettYoung Country 3.57 addSong C:/Users/Mayssaa/CS211/HW4/EveryLittleThing CarlyPearce Country 3.05 addSong C:/Users/Mayssaa/CS211/HW4/LosingSleep ChrisYoung Country 3.04 playList playSong ThinkingOutLoud playPreviousSong playNextSong playNextSong playNextSong deleteCurrentSong playNextSong deleteSong Perfect deleteSong TooGoodAtGoodbyes deleteSong Hello playAll Pop playAll Classic reversePlayList |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
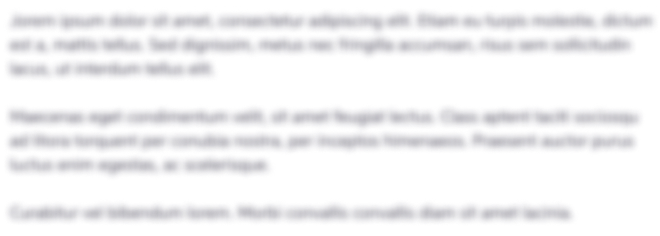
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started