Question
C++ | Class Members Your class should only have one private data field of type string . This variable will store a string in encrypted
C++ | Class Members
Your class should only have one private data field of type string. This variable will store a string in encrypted format. Do not store any other data in your EncryptedString object. Note that data fields are sometimes called member variables or data members.
Your class should have the following public methods (member functions):
- A default (no argument) constructor that stores an empty string in the data field.
- A constructor that takes a string as a parameter and stores the encrypted version of the parameter in the data field. This method should not actually do any "work". It should call the set method to encrypt and store the string.
- A method called set that takes a string as a parameter. It should encrypt the string parameter and store the result in the object. See the Encryption Algorithm section below for information on encrypting the characters of the string. Important:This method should discard any illegal characters in the string parameter. Remember that only alphabetic characters (upper and lower case letters A through Z) and the space (blank) character are allowed in an EncryptedString. The easiest way to remove any illegal characters is to start with an empty string. Then add characters as you encrypt them, leaving out the illegal characters. Important: Removing a character from a string should make the string shorter. Just replacing a character with a space is not the same as removing it.
- A method called get that returns a decrypted version of the string that is stored in the object. The returned value should be exactly the same as the original string that was stored but with any illegal characters removed. This method should not change the encrypted string stored in the object.
Your method prototypes should be:
EncryptedString(); EncryptedString( string str ); void set( string str ); string get();
Notice that inside an EncryptedString object, only the encrypted version of a string is stored. Code outside the class will only see "normal" (unencrypted) strings. Each EncryptedString object is responsible for encrypting the string to be stored, and decrypting the string before it is returned.
Normally, you would want to prevent code from outside the class from "seeing" the encrypted version of the string. However, for debugging purposes I want you to add one more public method to your class:
- A method called getEncrypted that returns the string in encrypted format. This method should not change the encrypted string stored in the object.
The method prototype should be:
string getEncrypted();
Note: If you know what a constant method is, make get() and getEncrypted() constant methods.
Encryption algorithm
- The space character is not encrypted. A space is stored as a space.
- An alphabetic character is replaced by its predecessor in the ASCII code sequence. For example, the predecessor of C (ASCII code 67) is B (ASCII code 66). Exceptions: For encryption purposes, the predecessor of A is Z, and the predecessor of a is z.
For example, the string Hi Mom! would be encrypted as Gh Lnl.
Hint: If you do not know how to find the next character in the ASCII code sequence, try out this program:
int main() { char ch = A; cout << ch << endl; cout << (ch + 1) << endl; return 0; }
Decryption algorithm
- The space character is not decrypted. A space is stored as a space.
- An alphabetic character is replaced by its successor in the ASCII code sequence. For example, the successor of C (ASCII code 67) is D (ASCII code 68). Exceptions: For decryption purposes, the successor of Z is A, and the successor of z is a.
Coding note: In my opinion, using character constants like 'A' makes your code much easier to read (as opposed to use ASCII codes like 65). I do not plan on memorizing the entire ASCII table.
Driver
Write a small driver program to test your class. Your program should exercise every class method and display enough information to convince me that your class is working correctly.
Requirements:
- Your program must be split into 3 files:
- the driver (test) program,
- a class header file (class definition with data member definitions and method prototypes only),
- and class implementation file containing class method definitions.
- The driver program for each programming project should begin with "header comments" containing: - Your name - Programming assignment number - A short description of the program - Course - The date For example: // Programmer: your name // Project number: 1 // Project Desc: EncryptedString class // Course: COSC 2436 PF III Data Structures // Date: date of submission
- Use a standard indentation convention in your code (see examples in textbook).
- Use meaningful variable names.
- Do not use global variables.
- Your class methods should not contain any input or output operations. All input and output should be done in your driver.
- If you are using Visual Studio, do not create a Win32 Console Project or Win32 Project. Create an Empty Project. Do not use any non-standard include files (like stdafx).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
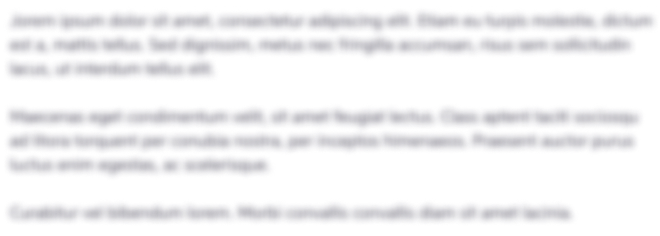
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started