Question
c++ // Code from: // Data Structures and Algorithms in C++, Goodrich, Tamassia, and Mount, 2nd Ed., 2011. // #pragma once #include using namespace std;
c++
// Code from:
// Data Structures and Algorithms in C++, Goodrich, Tamassia, and Mount, 2nd Ed., 2011.
//
#pragma once
#include
using namespace std;
template
template
class SNode { // singly linked list node
private:
E elem; // linked list element value
SNode
friend class SLinkedList
};
template
class SLinkedList { // a singly linked list
public:
SLinkedList(); // empty list constructor
~SLinkedList(); // destructor
bool empty() const; // is list empty?
E& front(); // return front element
void addFront(const E& e); // add to front of list
void removeFront(); // remove front item list
int size() const; // list size
private:
SNode
int n; // number of items
};
template
SLinkedList
: head(NULL), n(0) { }
template
bool SLinkedList
{
return head == NULL; // can also use return (n == 0);
}
template
E& SLinkedList
{
if (empty()) throw length_error("empty list");
return head->elem;
}
template
SLinkedList
{
while (!empty()) removeFront();
}
template
void SLinkedList
SNode
v->elem = e; // store data
v->next = head; // head now follows v
head = v; // v is now the head
n++;
}
template
void SLinkedList
if (empty()) throw length_error("empty list");
SNode
head = old->next; // skip over old head
delete old; // delete the old head
n--;
}
template
int SLinkedList
return n;
}
Use the template class SlinkedList discussed in class to implement a singly linked list of characters that store all the characters read from a line of text. The line of text will contain upper and lowe:r case letters, digits, punctuation signs and white spaces. Add two member functions, countVowel and countConsonant that count the number of vowels in the list, respectively the number of consonants in the list. For example, if the line of text given as a input is "Is dark at 6?!", the link list looks like: The number of vowels is 3and the number of consonants s sStep by Step Solution
There are 3 Steps involved in it
Step: 1
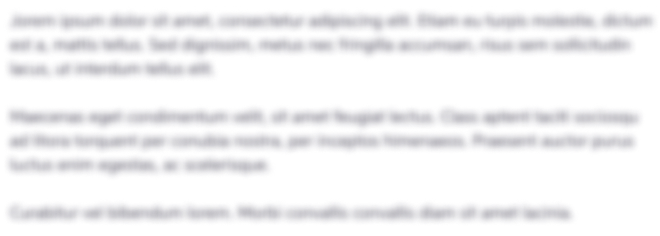
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started