Question
C++ Code Help: Problem: Implement the Stack and Queue interfaces with a unique class that is derived from class LinkedDeque using this code Fragment: ************
C++ Code Help:
Problem: Implement the Stack and Queue interfaces with a unique class that is derived from class LinkedDeque using this code Fragment:
************
typedef string Elem; // deque element type class LinkedDeque { // deque as doubly linked list public: LinkedDeque(); // constructor int size() const; // number of items in the deque bool empty() const; // is the deque empty? const Elem& front() const throw(DequeEmpty); // the first element const Elem& back() const throw(DequeEmpty); // the last element void insertFront(const Elem& e); // insert new first element void insertBack(const Elem& e); // insert new last element void removeFront() throw(DequeEmpty); // remove first element void removeBack() throw(DequeEmpty); // remove last element private: // member data DLinkedList D; // linked list of elements int n; // number of elements };
*******************************
//Main.CPP code:
#include "stdafx.h"
#include
#include
#include
#include
#include
#include
#include
using namespace std;
// define class
typedef string ElemType;
class LinkedDequeStack
{
// stack as a deque
public:
// constructor
LinkedDequeStack()
: LinkedDequeObj() { } // number of elements
// number of elements
int Size() const;
// check if stack is empty
bool Empty() const;
// the top element
const ElemType& Top() const throw(StackEmpty);
// push element onto stack
void Push(const ElemType& ele);
// pop the stack
void Pop() throw(StackEmpty);
private:
// deque of elements
LinkedDeque LinkedDequeObj;
};
// define size()
int LinkedDequeStack::Size() const
{
// return LinkedDequeObj.size()
return LinkedDequeObj.size();
}
// define Empty()
bool LinkedDequeStack::Empty() const
{
// return true or false
return LinkedDequeObj.empty();
}
// define Top()
const ElemType& LinkedDequeStack::Top() const throw(StackEmpty)
{
// check if the stack is empty
if (empty())
{
// throw exception
throw StackEmpty("Stack empty!");
}
// return LinkedDequeObj.back()
return LinkedDequeObj.back();
}
// define Push()
void LinkedDequeStack::Push(const ElemType& ele)
{
// call insertBack
LinkedDequeObj.insertBack(ele);
}
// define Pop()
void LinkedDequeStack::Pop() throw(StackEmpty)
{
// check if stack is empty
if (empty())
{
// throw exception
throw StackEmpty("Stack empty!");
}
// call removeBack()
LinkedDequeObj.removeBack();
}
// define class
typedef string ElemType;
class LinkedDequeQueue
{
// queue as a deque
public:
// constructor
LinkedDequeQueue()
: LinkedDequeObj() { }
// number of elements
int Size() const;
// check if queue is empty
bool Empty() const;
// the element in Front
const ElemType& Front() const throw(QueueEmpty);
// insert element in queue
void Enqueue(const ElemType& ele);
// remove element from queue
void Dequeue() throw(QueueEmpty);
private:
// deque of elements
LinkedDeque LinkedDequeObj;
};
// define size()
int LinkedDequeQueue::Size() const
{
// return LinkedDequeObj.size()
return LinkedDequeObj.size();
}
// define Empty()
bool LinkedDequeQueue::Empty() const
{
// return true or false
return LinkedDequeObj.empty();
}
// define Front()
const ElemType& LinkedDequeQueue::Front() const throw(QueueEmpty)
{
// check if the queue is empty
if (empty())
{
// throw exception
throw QueueEmpty(" Queue Empty! ");
}
// return LinkedDequeObj.back()
return LinkedDequeObj.back();
}
// define Enqueue()
void LinkedDequeQueue::Enqueue(const ElemType& ele)
{
// call insertBack
LinkedDequeObj.insertBack(ele);
}
// define Dequeue()
void LinkedDequeQueue::Dequeue() throw(QueueEmpty)
{
// check if queue is empty
if (empty())
{
// throw exception
throw QueueEmpty("Queue empty!");
}
// call removeFront()
LinkedDequeObj.removeFront();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
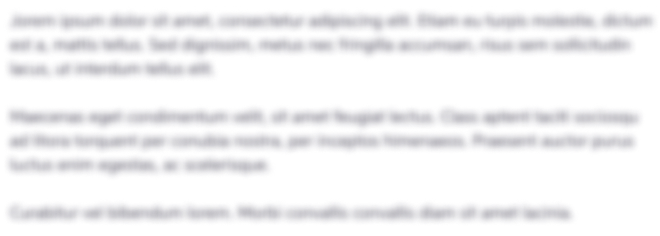
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started