Question
C++ Code Help! Problem: Write a complete adapter class that implements the sequence ADT using an STL vector object. Main.cpp #include stdafx.h #include #include #include
C++ Code Help!
Problem: Write a complete adapter class that implements the sequence ADT using an STL vector object.
Main.cpp
#include "stdafx.h"
#include
#include
#include
#include
#include
#include
#include
using namespace std;
int Elem; //Sequence base element type
class VectorSequence
{
public:
//iterator declaration
typedef vector::iterator Iterator;
//default constructor
VectorSequence();
//sequnce size
int size() const;
//is the list empty?
bool empty() const;
//beginning position
Iterator begin();
//last position
Iterator end();
//insert at front
void insertFront(const Elem& e);
//insert at rear
void insertBack(const Elem& e);
//insert e before position p
void insert(const Iterator& p, const Elem&e);
//remove first element
void eraseFront();
//remove last element
void eraseBack();
//remove elemet at position p
void erase(const Iterator& p);
//get position form index
Iterator atIndex(int i);
//get index form the position
int indexOf(const Iterator &p);
//...
private:
vector SeqVector;
};
// default constructor
VectorSequence::VectorSequence()
{
}
//The function size() that returns the number of elements stored in vector is defined below.
// define size ()
int VectorSequence::size() const
{
// return size() of the vector as size of the sequence
return SeqVector.size();
}
//The function empty() that returns true or false based on whether the vector is empty or not empty
//is defined below.
// define empty()
bool VectorSequence::empty() const
{
// call empty () in vector
return SeqVector.empty();
}
//The function begin() that returns the position of the first element in the vector is defined below.
//define begin()
VectorSequence::Iterator VectorSequence::begin()
{
// call begin() in vector
return SeqVector.begin();
}
//The function end() that returns the position of the last element in the vector is defined below.
//define end()
VectorSequence::Iterator VectorSequence::end()
{
//return just beyond the last position using end() in vector
return --SeqVector.end();
}
// define insertFront()
void VectorSequence::insertFront(const Elem& e)
{
// call insert() in vector
SeqVector.insert(SeqVector.begin(), e);
}
//The function insertBack() that takes an element as an argument and inserts it at the last position
//of the Vector SeqVector, the index of which is equal to the size of the Vector is defined below.
// define insertBack()
void VectorSequence::insertBack(const Elem& e)
{
// call push_back() in vector
SeqVector.push_back(e);
}
//The function insert() that takes an element and a position as arguments and inserts the element
//at the entered position of the Vector SeqVector is defined below.
// define insert()
void VectorSequence::insert(const Iterator& p, const Elem& e)
{
// call insert() in vector
SeqVector.insert(p, e);
}
define erase()
void VectorSequence::erase(const Iterator& p)
{
// call erase() in vector
SeqVector.erase(p);
}
//The function eraseFront() that deletes the first element of the Vector, that is the element present
//at index 0, is defined below.
// define eraseFront()
void VectorSequence::eraseFront()
{
Iterator p = SeqVector.begin();
// call erase() in vector
SeqVector.erase(p);
}
//The function eraseBack() that deletes the last element of the Vector, that is the element present
//at index size - 1, is defined below.
// define eraseBack()
void VectorSequence::eraseBack()
{
// call pop_back () in vector
SeqVector.pop_back();
}
// define atIndex()
VectorSequence::Iterator VectorSequence::atIndex(const int index)
{
// initialize position to beginning
Iterator position = SeqVector.begin();
//With the help of a for loop, increment the value of the position.
for (int i = 0; i< index; i++)
{
// increment position
position++;
}
// return position
return position;
}
// define indexOf()
int VectorSequence::indexOf(const Iterator &position)
{
// initialize index to beginning
int i = 0;
//With the help of a for loop, increment the value of index.
for (Iterator q = SeqVector.begin(); q != position; q++)
{
// increment index
i++;
}
// return index
return i;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
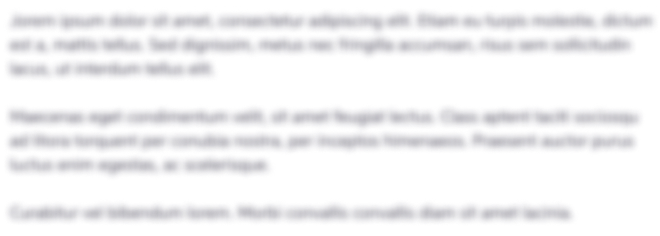
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started