Question
C++ Code: #include using namespace std; class Node { private: int val; Node* next; public: Node(); Node(int); display(); bool set_val(int); int get_val(); bool set_next(Node*); Node*
C++ Code:
#include
using namespace std;
class Node
{
private:
int val;
Node* next;
public:
Node();
Node(int);
display();
bool set_val(int);
int get_val();
bool set_next(Node*);
Node* get_next();
};
Node::Node()
{
val=0;
next=NULL;
}
Node::Node(int v)
{
val=v;
next=NULL;
}
Node::display()
{
/*cout
cout
cout
cout
cout
}
bool Node::set_val(int v)
{
val=v;
return true;
}
int Node::get_val()
{
return val;
}
bool Node::set_next(Node* n)
{
next=n;
return true;
}
Node* Node::get_next()
{
return next;
}
class MyList
{
private:
Node* start;
public:
MyList();
~MyList();
bool add(int);
bool remove(int);
bool is_present(int);
bool erase(int);
bool empty();
void display();
};
bool MyList::remove(int v)
{
Node* curr1=start;
if (!is_present(v))
return false;
if (start->get_val()==v)
{
Node* temp=start;
start=start->get_next();
delete temp;
return true;
}
while(curr1!=NULL)
{
Node* curr2=curr1->get_next();
if (curr2->get_val()==v)
{
curr1->set_next(curr2->get_next());
delete curr2;
return true;
}
curr1=curr1->get_next();
}
}
bool MyList::is_present(int v)
{
Node* curr=start;
while(curr!=NULL)
{
if (curr->get_val()==v)
return true;
curr=curr->get_next();
}
return false;
}
MyList::MyList()
{
start=NULL;
}
MyList::~MyList()
{
Node* curr=start;
while(curr!=NULL)
{
Node* temp=curr->get_next();
//cout get_val()
delete curr;
curr=temp;
}
}
bool MyList::add(int v)
{
Node* temp=new Node(v);
if (start==NULL)
{
start=temp;
return true;
}
temp->set_next(start);
start=temp;
return true;
}
void MyList::display()
{
Node* curr=start;
while(curr!=NULL)
{
cout get_val()
curr=curr->get_next();
}
cout
}
bool MyList::erase(int val)
{
}
bool MyList::empty()
{
}
int main()
{
MyList A;
A.add(2);
A.add(3);
A.add(4);
A.add(5);
A.add(6);
A.display();
}
Download the MyList class from the Oct 23 lecture. This is the Linked List design. Update the class to support the following features: (a) (1 point) An "erase" operation that deletes all elements in the list and makes it empty. (b) (2 points) An "is_duplicate" operation that checks if the input value is duplicate (meaning if there are multiple occurrences of the input value in the list). The function should return O if it is not a duplicate; otherwise it should return a total count of the number of occurrences of the input value in the list. (c) (1 point) A "remove_all" operation that removes all occurrences of a particular value (remember (d) (1 point) An "is_sorted" function that returns true if the list elements are sorted in ascending (e) (1 point) An "is_empty" function that returns true if the list is empty, false otherwise. that our list can contain duplicates) order, false otherwise. (f) (2 points) An insert function to insert a new element after a stated element in the list. If the stated element does not exist in the list, return false. For example, the class function bool insert(int v, int n) should insert the number v after the number n. The number n may or may not currently exist in the list. If it exists, insert v after n and return true, false otherwise. (g) (2 points). A function to remove duplicates. If a list contains the following elements , then after removing duplicates, it should only contain . Demonstrate that your code works using a main functionStep by Step Solution
There are 3 Steps involved in it
Step: 1
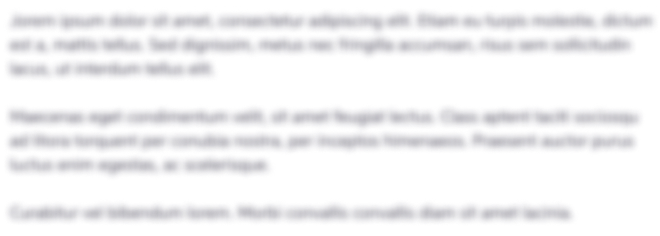
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started