Question
C++ Code provided. Need a function to check if a string contains properly balanced parenthesis. This function should return TRUE if a string containing braces,
C++
Code provided. Need a function to check if a string contains properly balanced parenthesis. This function should return TRUE if a string containing braces, parenthesis, and brackets is properly delimited. FALSE otherwise. Use stacks, queues or trees.
CODE:
#include
#include
using namespace std;
class Stack
{
private:
char *stackArray; // Pointer to the stack array
int stackSize; // The stack size
int top; // Indicates the top of the stack
public:
// Constructor
Stack(int);
// Copy constructor
Stack(const Stack &);
// Destructor
~Stack();
// Stack operations
void push(char);
void pop(char &);
bool isFull() const;
bool isEmpty() const;
char getTop();
};
//----------------------------------------------------------------
// Constructor: This constructor creates an empty stack.
// The size parameter is the size of the stack.
Stack::Stack(int size)
{
stackArray = new char[size];
stackSize = size;
top = -1;
}
//----------------------------------------------------------------
// Copy constructor
Stack::Stack(const Stack &obj)
{
// Create the stack array.
if (obj.stackSize > 0)
stackArray = new char[obj.stackSize];
else
stackArray = nullptr;
// Copy the stackSize attribute.
stackSize = obj.stackSize;
// Copy the stack contents.
for (int count = 0; count
stackArray[count] = obj.stackArray[count];
// Set the top of the stack.
top = obj.top;
}
//----------------------------------------------------------------
// Destructor
Stack::~Stack()
{
delete [] stackArray;
}
//----------------------------------------------------------------
// Member function push pushes the argument onto
// the stack.
void Stack::push(char val)
{
if (isFull())
{
cout
}
else
{
top++;
stackArray[top] = val;
}
}
//----------------------------------------------------------------
// Member function pop pops the value at the top of the stack
// off, and copies it into the variable passed as an argument
void Stack::pop(char &val)
{
if (isEmpty())
{
cout
}
else
{
val = stackArray[top];
top--;
}
}
//----------------------------------------------------------------
// Member function isFull returns true if the stack is full,
// or false otherwise.
bool Stack::isFull() const
{
bool status;
if (top == stackSize - 1)
status = true;
else
status = false;
return status;
}
//----------------------------------------------------------------
// Member function isEmpty returns true if the stack is
// empty, or false otherwise.
bool Stack::isEmpty() const
{
bool status;
if (top == -1)
status = true;
else
status = false;
return status;
}
char Stack:: getTop()
{return top;}
//----------------------------------------------------------------
// Function prototypes
void reverseString (string & s);
bool isBalanced(string s);
int main()
{
cout
string word; // To hold input string
cout
getline(cin, word);
cout
reverseString(word);
cout
cout
string str; // To hold input string
cout
getline(cin, str);
if (isBalanced(str))
{
cout
}
else
{
cout
}
return 0;
}
// function definitions
#includeStep by Step Solution
There are 3 Steps involved in it
Step: 1
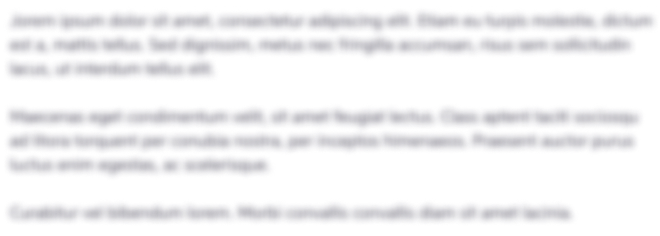
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started