Question
***C++ Code*** Write a menu-driven C++ application to do the activities shown in the associated example output. All of the activities involve reading the provided
***C++ Code***
Write a menu-driven C++ application to do the activities shown in the associated example output. All of the activities involve reading the provided file, names.txt, which gives babies' names in the U.S. and their popularity rankings for 11 decades beginning in 1900. In this file a popularity of 0 indicates a ranking that was 1000 or more. Each of the 4429 lines in this file starts with a baby's first name and is followed by eleven (11) integers representing the popularity ranks for that name for the 11 decades from 1900 through 2005.
There should be four (4) menu items the user may choose:
-Print histogram for a name: The histogram line should be longer the more popular that name is. So, a rank of 1 gets the most stars (100) and a rank of 999 gets only one star. Names whose ranks are zero (0) should show no stars since the zero implies a popularity of 1000 or more. You should aim to get 100 stars for rank 1 and 1 star for rank 999. This can be done by accessing a name's rank for a specific decade and plugging it into the expression: (1000 - rank) / 10 + 1. If the entered name is not found in the list of names, then an error is printed and the menu is redisplayed for another user selection. See the example output.
-Compare two names in a decade: The user should be prompted to enter each of the two names and the decade value (1 through 11). Handling of errors (a name is not found or an invalid entry for decade) is shown in the example output.
-Print top ten names for a decade: List the top 10 names for a given decade in order of decreasing popularity (or increasing rank: first 1, then 2, then 3, etc.). The user should be prompted for the decade in which to search. NOTE: This will display 20 names since for each popularity rank there are two name. See the example output.
-Quit (write anomalies): This menu choice causes the application to terminate. However, before terminating, the application must print all anomalies found in names.txt to an output file named anomalies.txt. The file's format is defined to have two names for every decade/rank pair. For example, if examining decade 7 (1960-1969) and the rank, 9, this would yield the names, Michelle and Thomas. Any pair of a decade and a rank should correspond to two names as in the previous example. However, there are anomalies in the file such that some decade/rank pairs have only one name and a few of these pairs have no name at all. Writing the anomalies to an output file means that your program will search the array of names looking for these anomalies and print a list of these problems with the file's format to the output file. As it turns out, there are 1,065 anomalies: in most cases, only one name with a particular rank in a decade or in a few cases, no names in a decade with a particular rank. Your code to do this will need to check each name in each decade for each rank in order to find all 1065 anomalies. The format of the two different kinds of anomalies you will write to the file named, anolamies.txt, follows: 23 - One name(Wilma) for 1900-1909, rank 139. 676 - No names for 1940-1949, rank 779. - Note: Write out one anomaly per line and number each anomaly written. In the two examples above, the first one was the 23rd anomaly found and the second was the 676th anomaly found. You may find them in a different order, but you numbers should go from 1 to 1065. - Also note, the first type of anomaly that the one name found is given in the description of that anomaly,
NOTE: Examine the example output with the goal of making your output look like the example output.
When the user is asked to enter an integer, assume that the user will not enter characters that are not a number. However, if the number entered is out of range, those errors should be validated. Once again, the example output shows the handling of such errors, including names entered by the user that are not found in the list. Ignore the case of user input; for example, "jOHn", "John", "JOHN", and "john" should all be the same name as far as the program is concerned.
Design: Use the following struct to store the data contained on one line of the file, names.txt:
const int NAME_LEN = 21; const int NUM_RANKS = 11; . . . struct Name { char name[NAME_LEN]; int rank[NUM_RANKS]; };
The function, loadNames, should be called to read the entire names.txt file and store the data from each line into a struct of type Name. The main function should pass an array of Name structs to loadNames so that it can simply read a line's data into the struct at the first element of this array, then read the second line's data into the second element, etc. This should be done for all 4429 lines of the names.txt file. Once loadNames has completed and returned to main, the file, names.txt, must never be read again during this execution of the application.
Be sure to use named constants for all numbers like 21, 11, 4429, etc.
Once the array of Name structs is populated by the loadNames function, then the function, main, can present the menu and get a selected menu choice from the user. Here is an example of the main program loop:
Name list[SIZE]; bool done = false; loadNames( list ); // main app loop do { switch( getMenuSelection() ) { case HISTOGRAM: displayHistogram( list ); break; case COMPARE: compareNames( list ); break; case TOPTEN: displayTopTenNames( list ); break; case EXIT: done = true; break; } // end main app loop } while( !done );
This example uses the constants:
const char HISTOGRAM = 'a'; const char COMPARE = 'b'; const char TOPTEN = 'c'; const char EXIT = 'd';
You should setup your main function in a similar fashion. Make sure you do NOT use recursion -- this is when a function either invokes itself or invokes another function that invokes the first one. Use loops to cycle back to the menu presentation after executing the user's choice. In the example above, the array of Name structs is created in main, populated by loadNames, and the passed to each major function in the list of menu choices. Once an individual task is complete, control returns to main where the application continues to offer the menu once again only if the user has not selected the terminate option.
You should notice that the Name struct uses a C-String to store the name found on a line of the text file. You must use this struct and the c-string field, name, exactly as defined above. Using a c-strings requires library functions like, strcpy, strcmp, etc. (see textbook). If you want to use other strings in the application, you are welcome to use the data type, string, for these -- but not for the Name stuct's name field. The string type is found in many examples in the textbook.
Your grade on this project will depend heavily on your implementation of a modular design. This implies that you use smaller functions to accomplish larger tasks. This means writing functions to do tasks that are repeated, such as searching for a name, changing a name's case, organizing a line of a histogram, etc. This also means creating functions to hold meaningful subdivisions of the work. Examples of these are the functions called for each of the menu cases in the switch statement above.
As you organize the structure of your solution, remember: NO recursion allowed, every function should have ONLY one return statement, break is only used in a switch statement (NOT to terminate loops), and continue and exit are never used in this course
Again, the file, names.txt, must read the file once only. This would be done by the loadNames function called by main before the main program loop ever begins.
Use named constants wisely. Some examples were given above. You will want others.
Every function should have a prolog comment that makes very clear what the task of the function is. Be sure the main prolog COMPLETELY and CLEARLY summarizes all the functionality of this application. Finally, use inline comments to make your code simple to read and understand. Again, make your execution resemble the example output as closely as possible.
Turn in, nameApp.cpp via Canvas before the due date/time.
HINTS:
Start this project ASAP, if not sooner. Read through the requirements (above) and the example output and list any questions you have along the way. Re-read both documents to answer questions; email me questions you cannot answer for yourself. Divide the problem into parts using the menu items: part a (print a histogram), part b (compare two names), etc. Do one part at a time. Write utility (helper) functions to keep from having redundant code.
Beginning and Completing this Project
Use stepwise refinement as your method of design and implementation. What this means is that you carve out a small part of the project, proceed to design it and then implement it. When this part is implemented, you should be able to compile it and run it. After testing the completed part, choose another chunk to design and add its implementation to what you already have. Now compile and test what you have completed so far. When you have gone back to design and implement all parts of the problem, you will be ready to do the final testing of the entire application.
For this project, here are the parts I would isolate and the order in which I would design, implement and test:
Read file into an array of Name structs
Create the array in main, pass it to the loadNames function and let that function populate the contents of each Name struct in the array using the data from successive lines of the text file, names.txt
Test this, by printing out random names and their popularity rankings
For example, if main named the array, list, then upon returning from the loadNames function, do something like: cout << "Element[333] = " << list[333].name << " and its rank[10] = " << list[333].rank[10] << endl;
High-level menu and user-interface
Before we can choose a menu option, we need to be able to present the user a menu, get the user's choice and then do what the user asked, then loop back to the point where we re-display the menu and wait for another selection to be made.
So we need a loop to iterate until the user selects the D (quit) option
The loop should contain a switch statement that directs our application to perform the correct tasks for each of the other options as well
Since the user began the application, we can assume the user wants to see the menu at least once ? this implies a do..while loop! So how about:
do { // here we display the menu, get a selection and process it } while ( !done );
done can be a boolean variable that we initialize to false, then all we have to do is set it to true when the D option is chosen, signalling that the user is now DONE! A switch statement can help us divide up how this code will direct the traffic
do { displayMenu(); choice = getUsersChoice(); switch( choice ) { case 'A': displayHistogram( list ); break; case 'B': compareTwoNames( list ); break; case 'C': displayTopTenNames( list ); break; case 'D': writeAnomaliesToFile( list ); done = true; break; } } while ( !done );
NOTE: when you first implement this, the functions called inside the switch statement should ONLY BE STUBS so that you do not work at implementing them until later. A stub function is one that would merely print a message such as: "Reached stub for compareTwoNames." until you get around to fully implementing it later.
You should now be able to run nameApp and see that the menu and the interface loop are working properly.
Menu Option A: Displaying a Histogram
Design and implement the function, displayHistogram, and its helper functions -- like a search function that is passed the list and the name entered by the user, and returns the index of where the name was found in the list or -1 if the name is not found; another helper function might be one that composes a single line of the histogram given a particular rank value.
Since you will have to get a name from the user and search for it in the array, it would be a good idea to write these as two separate functions
Use the information that your search provides: if search can find the name, then have it return the index of this name in the array so that you can easily access the rank array for that struct element
Now you should be able to run nameApp, choose A and see a histogram for the name you enter.
You should also be able to quit (but withOUT writing the file anomalies YET!)
ONLY when everything above of working smoothly, go on to the next part
Menu Option B: Comparing Two Names
Design an implement the function, compareTwoNames( list ), and any helper function
Note that this function needs two names from the user: you wrote a helper function for this when working on the previous part
Note that you must also search for each name before continuing: the search too was written for the previous part!
You will also need a decade value from the user: here is another helper that will be needed in the third (C) part!
You should now be able to run nameApp and do parts A and B and quit.
Menu Option C: Top Ten Names
Design and implement this part by designing and implementing the function that will do this work
Don't forget to DESIGN: use pseudocode to try various ideas
Proceed with the design, implementation and testing of this newest part
Make sure the first two parts are still working
Menu Option D: Finding File Anomalies and Quit
Now design and implement the function to find and write the anomalies to a text file
Call this function and set the do..while loop boolean, done, to true
Now you can test the entire application
Example output code:
Welcome to nameApp. Use menu options to look at various statistics for names popular between 1900 and 2005. Select a menu option: a - Print histogram for a name b - Compare two names in a decade c - Print top ten names for a decade d - Quit (write anomalies) Your selection: a Enter a name: waLTer Histogram for name, Walter 11: *************************************************************************************************** 10: **************************************************************************************************** 15: *************************************************************************************************** 22: ************************************************************************************************** 27: ************************************************************************************************** 52: *********************************************************************************************** 80: ********************************************************************************************* 107: ****************************************************************************************** 158: ************************************************************************************* 245: **************************************************************************** 310: ********************************************************************** Select a menu option: a - Print histogram for a name b - Compare two names in a decade c - Print top ten names for a decade d - Quit (write anomalies) Your selection: a Enter a name: thelMa Histogram for name, Thelma 38: ************************************************************************************************* 28: ************************************************************************************************** 35: ************************************************************************************************* 67: ********************************************************************************************** 143: ************************************************************************************** 237: ***************************************************************************** 391: ************************************************************* 711: ***************************** 0: 0: 0:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
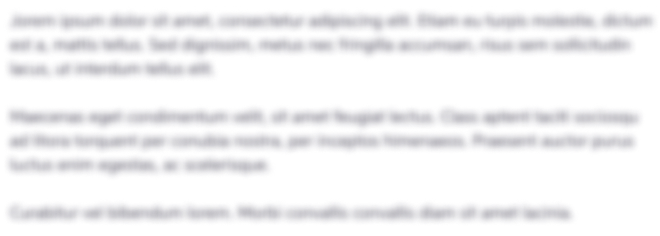
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started