Question
C# coding I need help with an error that makes no sense. It says I made a variable but never used it. Which I did
C# coding
I need help with an error that makes no sense. It says I made a variable but never used it. Which I did in the next few lines. Can you look at it and give me the corrected code. Formated too please.
Instructions for assignment.
In Chapter 6, you continued to modify the GreenvilleRevenue program. Now, modify the program so that the major functions appear in the following individual methods:
- GetContestantNumber - This method gets and returns a valid number of contestants and is called twice once for last years number of contestants and once for this years value
- DisplayRelationship - This method accepts the number of contestants this year and last year and displays one of the three messages that describes the relationship between the two contestant values
- GetContestantData - This method fills the array of competitors and their talent codes
- GetLists - This method continuously prompts for talent codes and displays contestants with the corresponding talent until a sentinel value is entered
An example of the program is shown below:
Enter number of contestants last year >> 2 Enter number of contestants this year >> 3 Last year's competition had 2 contestants, and this year's has 3 contestants Revenue expected this year is $75.00 The competition is bigger than ever! Enter contestant name >> Matt Talent codes are: S Singing D Dancing M Musical instrument O Other Enter talent code >> S Enter contestant name >> Sarah Talent codes are: S Singing D Dancing M Musical instrument O Other Enter talent code >> D Enter contestant name >> Bruno Talent codes are: S Singing D Dancing M Musical instrument O Other Enter talent code >> M The types of talent are: Singing 1 Dancing 1 Musical instrument 1 Other 0
In order to prepend the $ to currency values, the program will need to use the CultureInfo.GetCultureInfo method. In order to do this, include the statement using System.Globalization; at the top of your program and format the output statements as follows: WriteLine("This is an example: {0}", value.ToString("C", CultureInfo.GetCultureInfo("en-US")));
MY CODE:
using System;
using static System.Console;
using System.Globalization;
class GreenvilleRevenue
{
static void Main()
{
const int ENTRANCE_FEE = 25;
const int MIN_CONTESTANTS = 0;
const int MAX_CONTESTANTS = 30;
int numThisYear;
int numLastYear;
int revenue;
string[] names = new string[MAX_CONTESTANTS];
char[] talents = new char[MAX_CONTESTANTS];
char[] talentCodes = { 'S', 'D', 'M', 'O' };
string[] talentCodesStrings = { "Singing", "Dancing", "Musical instrument", "Other" };
int[] counts = { 0, 0, 0, 0 }; ;
numLastYear = GetContestantNumber("last", MIN_CONTESTANTS, MAX_CONTESTANTS);
numThisYear = GetContestantNumber("this", MIN_CONTESTANTS, MAX_CONTESTANTS);
revenue = numThisYear * ENTRANCE_FEE;
Console.WriteLine("Last year's competition had {0} contestants, and this year's has {1} contestants",
numLastYear, numThisYear);
Console.WriteLine("Revenue expected this year is {0}", revenue.ToString("C"));
DisplayRelationship(numThisYear, numLastYear);
GetContestantData(numThisYear, names, talents, talentCodes, talentCodesStrings, counts);
GetLists(numThisYear, talentCodes, talentCodesStrings, names, talents, counts);
}
//This method gets and returns a valid number of contestants and is called twice once
//for last years number of contestants and once for this years value
public static int GetContestantNumber(string when, int min, int max)
{
string entryString = string.Empty;
int num = 0;
Console.Write("Enter number of contestants {0} year >> ", when);
GetContestantNum(ref num);
while (num max)
{
Console.WriteLine("Number must be between {0} and {1}", min, max);
Console.Write("Enter number of contestants {0} year >> ", when);
GetContestantNum(ref num);
}
return num;
}
//This method accepts the number of contestants this year and last year and displays
//one of the three messages that describes the relationship between the two contestant values
public static void DisplayRelationship(int numThisYear, int numLastYear)
{
if (numThisYear > 2 * numLastYear)
Console.WriteLine("The competition is more than twice as big this year!");
else
if (numThisYear > numLastYear)
Console.WriteLine("The competition is bigger than ever!");
else
if (numThisYear
Console.WriteLine("A tighter race this year! Come out and cast your vote!");
}
//This method fills the array of competitors and their talent codes
public static void GetContestantData(int numThisYear, string[] names, char[] talents, char[] talentCodes, string[] talentCodesStrings, int[] counts)
{
int x = 0;
bool isValid;
while (x
{
Console.Write("Enter contestant name >> ");
names[x] = Console.ReadLine();
Console.WriteLine("Talent codes are:");
for (int y = 0; y
Console.WriteLine(" {0} {1}", talentCodes[y], talentCodesStrings[y]);
Console.Write(" Enter talent code >> ");
talents[x] = GetChar();
isValid = false;
while (!isValid)
{
for (int z = 0; z
{
if (talents[x] == talentCodes[z])
{
isValid = true;
++counts[z];
}
}
if (!isValid)
{
Console.WriteLine("{0} is not a valid code", talents[x]);
Console.Write(" Enter talent code >> ");
talents[x] = GetChar();
}
}
++x;
}
}
//This method continuously prompts for talent codes
//and displays contestants with the corresponding talent until a sentinel value is entered
public static void GetLists(int numThisYear, char[] talentCodes, string[] talentCodesStrings, string[] names, char[] talents, int[] counts)
{
int x;
char QUIT = 'Z';
char option;
bool isValid;
int pos = 0;
bool found;
Console.WriteLine(" The types of talent are:");
for (x = 0; x
Console.WriteLine("{0, -20} {1, 5}", talentCodesStrings[x], counts[x]);
Console.Write(" Enter a talent type or {0} to quit >> ", QUIT);
option = GetChar();
while (option != QUIT)
{
isValid = false;
for (int z = 0; z
{
if (option == talentCodes[z])
{
isValid = true;
pos = z;
}
}
if (!isValid)
Console.WriteLine("{0} is not a valid code", option);
else
{
Console.WriteLine(" Contestants with talent {0} are:", talentCodesStrings[pos]);
found = false;
for (x = 0; x
{
if (talents[x] == option)
{
Console.WriteLine(names[x]);
found = true;
}
}
if (!found)
Console.WriteLine("No contestants had talent {0}", talentCodesStrings[pos]);
}
Console.Write(" Enter a talent type or {0} to quit >> ", QUIT);
option = GetChar();
}
}
#region Helper Private Methods
//Tryparse is used for checking the valid number datatype
private static void GetContestantNum(ref int num)
{
string entryString = string.Empty;
bool isValidNumber = false;
while (!isValidNumber)
{
entryString = Console.ReadLine();
isValidNumber = int.TryParse(entryString, out num);
if (!isValidNumber)
{
Console.WriteLine("Invalid number: {0} Please try again!", entryString);
}
}
}
//Tryparse used for checking the character datatype
private static char GetChar()
{
char validChar = '0';
bool isValidChar = false;
var charInput = string.Empty;
while (!isValidChar)
{
charInput = Console.ReadLine();
isValidChar = Char.TryParse(charInput, out validChar);
if (!isValidChar)
{
Console.WriteLine("Invalid character - Please try again by entering one character!");
}
}
return validChar;
}
#endregion
}
This is what the error says and what I see.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
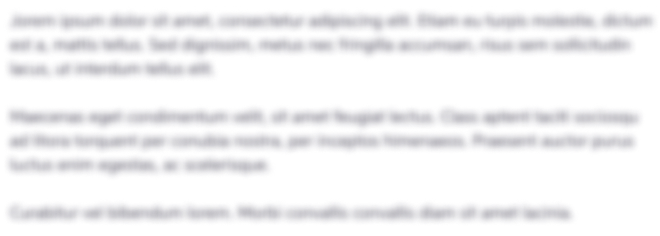
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started