Question
C++ coding program. A Restaurant manager wants you to create a program to keep track of all of his inventory and workers. You are given
C++ coding program.
A Restaurant manager wants you to create a program to keep track of all of his inventory and workers. You are given 4 files: Food.txt, Employee.txt, Condiment.txt, and PlasticItem.txt. Create an array of structs for each file to hold the entries.
Food.txt
1. Name
2. Number Remaining
3. Expiration Date (month:day:year)
4. Cost
Employee.txt
1. Name
2. Id
3. Salary
4. Hire Date (month:day:year)
5. Rating (1-10)
Condiment.txt
1. Name
2. Ounces Remaining
3. Expiration Date (month:day:year)
4. Cost
PlasticItem.txt
1. Name
2. Number Remaining
3. Cost
Write a C++ program to help the Fast Food store owner. Create four arrays of structs named Food, Employee, Condiment, and PlasticItem. The size of the array should be read in from the file. Structs should be created to hold the variables that wont fit a standard data type. The main() function of your program should be very simple. The main function should be a collection of variable declarations and function calls. You will need to use four different functions to read the data from the four files. You can add more functions if you want. You are to give the user the ability to print out each sections records (Food, Employee, Condiment, and PlasticItem). Do NOT use global variables.
In the first line of each file there will be a number indicating how many entries are in the file.
Food.txt
15 Tomato 20 07:02:2015 1.95 Cucumber 89 06:15:2014 .89 Bread 4 06:25:2017 2.69 Eggs 11 08:29:2015 2.33 Turkey 56 05:01:2010 1.72 Orange 16 04:19:2011 .12 Lettuce 40 09:05:2002 .30 HotDog 22 05:20:2010 .99 Chicken 9 03:11:2016 1.01 Bun 1 06:24:2013 1.89 Burger 0 03:29:2016 66.60 Rib 16 06:15:2013 1.23 Apple 18 08:21:2012 1.87 Banana 44 10:22:2013 1.95 Carrot 21 09:22:2013 1.99
Condiments.txt
14 Ketchup 20 01:16:2000 1.00 Mustard 40 05:14:2006 4.20 Relish 15 10:21:2015 5.50 SoySauce 98 09:08:2011 2.70 Mayonnaise 52 06:03:2013 1.60 BBQSauce 11 11:10:2004 2.00 HotSauce 70 12:06:2014 .95 Salsa 700 06:12:2003 8.00 Wasabi 15 07:18:2006 1.00 OysterSauce 12 08:22:2007 2.60 Horseradish 200 08:23:2015 1.95 TarterSauce 90 06:25:2016 2.10 FishSauce 33 04:23:2015 3.23 BrownSauce 20 08:26:2014 2.00
Employee.txt
16 Ellis 209 32750 04:01:2010 10 Patrick 676 55549 03:10:2013 3 Tommy 257 53383 06:21:2000 3 Alfie 694 27177 06:01:2005 8 Harley 971 27040 07:06:2006 2 Jeffery 690 63713 02:26:2008 3 Bently 769 70142 02:27:2015 2 Kellen 468 10978 07:13:2012 1 Ryan 894 95825 08:17:2015 7 Alexander 633 41944 06:06:2016 1 Zak 619 9499 03:22:2015 5 Ashton 987 72388 06:13:2013 9 Mason 275 63900 04:14:2017 8 Alex 460 39871 07:23:2016 7 Emery 601 51050 03:20:2010 3 Wade 748 29487 02:28:2014 5
PlasticItem.txt
10 Forks 1000 .20 Spoons 215 .65 TrashBags 15 1.60 Napkins 5000 .20 Cups 60 .95 Plates 101 1.26 Bowls 15 2.10 Vincent 96 .55 Straws 180 1.05 Containers 280 1.32
Sample Output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
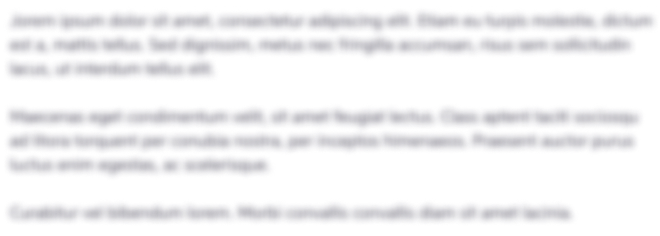
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started