Question
C++ Connect 4 Game (Classic, not Frenzy) Your solution must have these classes and at least these fields / methods. Your program must use the
C++
Connect 4 Game (Classic, not Frenzy)
Your solution must have these classes and at least these fields / methods. Your program must use the methods described in a meaningful fashion. You can create additional fields or methods but these the below should be core methods in your solution.
Chip class
fields:
color
methods:
Constructor
int getColor()
string toString() - returns an attractive string representation of itself
Board class
fields:
2D array of Chips - represents the game board (6 row by 7 column)
------ Example of part of a .h file with a 2D array -----
class Board
{
private:
static const int SIZE_COLUMNS = 7;
static const int SIZE_ROWS = 6;
int board[SIZE_ROWS][SIZE_COLUMNS];
};
----------------------------------------------------------
methods:
Constructor
string toString() - returns an attractive string representation of itself
This needs to be an attractive table representation of the game board with aligned rows / columns with characters representing blank cells, cells with a red chip in it and cells with a gold chip in it.
int dropChip(Chip, int) - drops a Chip object down a particular slot. Returns an int indicating success or not.
int isWinner() - returns code number representing no winner or player number of winner
Player class
fields:
name
color
methods:
Constructor
get / set methods for fields
string toString() - string representation of itself.
Example String representation of the board (X means blank, R means red chip, G means gold chip)
X X X X X X X
X X X X X X X
X X X X X G X
X X X X X R X
X X X X X R X
X R G G G R G
Main
Your program should create two Players and a Board. It should represent the initial Board to the screen.
You should then alternate between player 1 and player 2 and randomly drop chips down slots in the board. You should represent the board after each chip drop.
This should stop if there is a winner or if the board fills up.
You should be turning in a Chip.h, Chip.cpp, Board.h, Board.cpp, Player.h, Player.cpp and a gamedriver.cpp that has your main in it.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
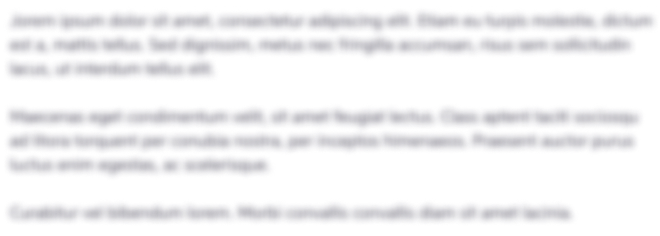
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started