Question
C++ create a simple version of the Memory Game. Program Description While this program will be an introduction to using structs, it is also a
C++ create a simple version of the Memory Game.
Program Description
While this program will be an introduction to using structs, it is also a review of overloaded function, dynamic memory, and the use of pointers. The implementation described in this document is not the only way to do such a program and it could be done with different functions, but these particular methods are designed to provide practice in specific concepts.
For this program there should be two structs defined. One will be used to store and pass a move and the other will be used to represent a cell on the playing board. The board should be dynamically created as an array of 16 of these cells.
For the symbols, use the eight characters A, B,C, D, E, F, G, H. They should be defined in a static array of chars in the function that creates the board.
For each turn, display the current state of the board, showing an X or a SPACE at each location. Each location starts with an X that is replaced with a SPACE when a match is found.
After showing the current state, get a move. Then redisplay the state, but at the moves location, show the correct symbol instead of an X. Then get second move and display the board with each of the two locations showing the correct symbol instead of an X.
Then check for a match; if there is a match update the board replacing the Xs with a SPACE for the two cards that were selected.
The game should end after the player has cleared the board by making eight matches.
Properly implement both header and source files for your functions. All input should be validated as integer and within range.
Program Requirements
What is expected to be included in the header file.
Include the function declarations and the following global definitions.
Global definitions
Define constants as needed, for example
ROW_SIZE = 4, BD_SIZE = 16, NUM_SYMS = 8, SPACE = , UNKNOWN = X
Define two structs
move contains two integers, one for row and one for column.
cell contains a bool found and a char value (the hidden letter)
Helper Functions
getInteger
O used to get an input value
O input parameters are min and max value
O get and validates an integer between min and max
O return the value
yes Or No
O used to respond to a question
o input parameter is a string message to display
o get input, verify if yes or no (y/n)
o return true for yes, false for no
isThere
O used to see if a location still has a card
O input parameters are the board and a move, both passed by safe reference
O checks if the card has been found or not
O return true if there is a card there, returns false if is removed
getIndex
O used to simplify access to the flattened board
O input parameter is a move passed by safe reference
O converts the row and column of the move, see flattening arrays doc
O returns the index 0-15
Game Functions
displayInstructions
ono input parameters
o displays the instructions for the game, simplified for a second round
ono return values
createBoard
O no input parameters
o dynamically creates a board of BOARD_SIZE cells
o sets all found members to false
o fills all values members from the set of possible values, two of each on board
o calls shuffle to randomize the locations
o returns the board
shuffle
O input parameter is a board (passed by pointer)
O randomly shuffles the board, see hints below
o no return values, board is updated by reference
getMove
O input is a reference to the move struct for the first move and the board passed safely
O get two integers(row, col), validate that they are in range(0-3)
O see if it is a valid location by calling is There
O update the move struct for the first move
O no return values
showBoard
o input parameters the board and move1; all passed by safe reference
o display the board as a 4x4 grid using nested for loops for each cell other than move1
if found is true display SPACE else UNKNOWN for move 1 display its value member
ono return values
showBoard
o input parameters the board, move1, move2; all passed by safe reference
o display the board as a 4x4 grid using nested for loops for cells other than move1 and move1
if found is true display SPACE else UNKNOWN for move1 and move2display their value members
ono return values
checkMatch
o input parameters the board, move1, move2; all passed by safe reference
o if the cell at move1 has the same value as the cell at move2 return true
o else return false
updateBoard
input parameters the board, move1, move2(moves are passed by safe reference)
update each the cells at move1 and move2 by changing found from false to true
ono return values
The main program should have two loops, the outer loop repeats with a new game as long as the player wants to keep playing, the inner loop continues until the game ends with 8 matches. There should be a counter of how many tries it took. The board and counter should reset between games. The main program needs variables for move1, move2, the board, and a counter of how many tries
Step by Step Solution
There are 3 Steps involved in it
Step: 1
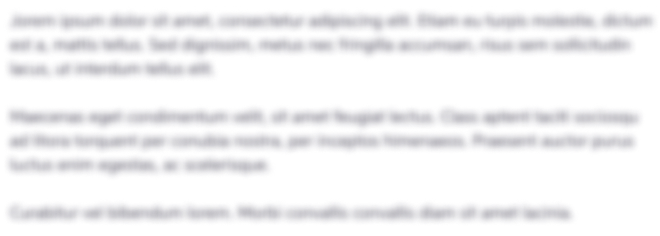
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started