Question
C++ Create a single-file application that will read zero or more32-bit hex values and then print a detailed description of thefloating point value that they
C++
Create a single-file application that will read zero or more32-bit hex values and then print a detailed description of thefloating point value that they represent by extracting and showingthe sign, exponent, and significand from the 32-bit value in themanner described below.
This file must contain (at least) two functions: main() andprintBinFloat(uint32_t x). Your main must have a read-loop thatcalls printBinFloat to do the decoding and printing.
- Do not define any global or static-class variables.
- Do not define or use any floating point variables oroperations.
- printBinFloat(uint32_t x) must use the C bitwise operators tointerpret, extract, and shift the fields of the IEEE number asneeded to render the output as described below. (In other words,you must not use things like the bitset STL template class.)
- You must use std::cin and std::cout (the iostreams) for readingand printing all of your data (in other words, you may not use notprintf/scanf/fstream/read/write....)
Read your input from stdin (aka std::cin).
To read a 32-bit hexadecimal value from stdin use the std::hexmanipulator with the std::cin stream as shown below:
uint32_t x;std::cin >> std::hex >> x;
Write your output to stdout (aka std::cout).
printBinFloat must format and print each value precisely in thefollowing manner.
0x3f800000 = 0011 1111 1000 0000 0000 0000 0000 0000sign: 0 exp: 0x00000000 (0) sig: 0x00000000+1.00000000000000000000000
In this particular example, the hex value 3f800000 was read andthe values of its fields are shown as well as its actual binaryvalue.
For each input value, the five output lines printed are:
- The first line displays the input value as a 32-bit hex valueand again in binary (in groups of 4-bits.)
- The sign bit is zero. (Therefore the floating point value ispositive.)
- The exponenet is zero (seen printed as a 32-bit signed integerand as a signed decimal value in parenthesis.)
- The significand is printed as a 32-bit unsigned hex value. Inthis case it is zero.
- The full value of the number is printed last in binary.
Positive infinity must be displayed like this: +inf and negativeinfinity: -inf as shown below:
0x7f800000 = 0111 1111 1000 0000 0000 0000 0000 0000sign: 0 exp: 0x00000080 (128) sig: 0x00000000+inf0xff800000 = 1111 1111 1000 0000 0000 0000 0000 0000sign: 1 exp: 0x00000080 (128) sig: 0x00000000-inf
The positive and negative zero values must be printed likethis:
0x00000000 = 0000 0000 0000 0000 0000 0000 0000 0000sign: 0 exp: 0xffffff81 (-127) sig: 0x00000000+00x80000000 = 1000 0000 0000 0000 0000 0000 0000 0000sign: 1 exp: 0xffffff81 (-127) sig: 0x00000000-0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
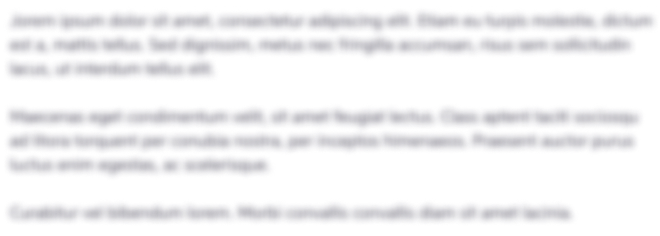
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started