Question
C++ Create a template class definition for the Set class implemented below and write a main driver program to allow for the instantiation of objects
C++
Create a template class definition for the Set class implemented below and write a main driver program to allow for the instantiation of objects from multiple class specializations. The driver program should show that the set operations work correctly for each class specialization.
class Set {
public:
//default constructor
Set();
Set( int [], int, char );
Set(Set &s);
//add element to set
void addElement (int element);
//remove element from set
void removeElement(int element);
//check for membership
bool isMember(int element);
//size of set
int size();
//get element i
int getElement (int i);
bool operator==(Set& s); //equality comparison
bool operator !=(Set& s); //inequality comparion
bool operator + (int element); //add element operator
Set operator + ( Set& s); // set union opreator
Set operator ^ ( Set& s);//to represent the intersection of two sets
bool operator - (int element); //remove element operator
Set operator - (Set& s); //aset difference operator
//accessor for name
char getName();
void setName(char nam);
private:
int *set;// pointer to a dynamically allocated array of integers. This array will contain the elements that comprise the set.
int pSize;// data member to represent the physical size of the array.
int numElements;//data member to represent the number of elements in the set.
char name;//data member to represent the name of the set. Note that a set is typically named using an uppercase letter of the English alphabet.
static const int DEFAULT_SIZE=10;// (static constant) data member to represent the default value used to dynamically allocate memory.
};
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
Set.cpp:
#include "Set.h"
#include
#include
#include
using namespace std;
//default constructor
Set::Set()
{
set=new int[DEFAULT_SIZE];
pSize=DEFAULT_SIZE;;// data member to represent the physical size of the array.
numElements=0;//data member to represent the number of elements in the set.
name='A';
}
Set::Set( int a[], int n, char nam)
{
set=new int[n+1];
pSize=n+1;// data member to represent the physical size of the array.
numElements=0;//data member to represent the number of elements in the set.
name=toupper(nam);
for(int i=0;i addElement(a[i]); } Set::Set(Set &s) { set=new int[s.size()]; pSize=s.size();// data member to represent the physical size of the array. numElements=0;//data member to represent the number of elements in the set. name=s.getName(); for(int i=0;i addElement(s.getElement(i)); } char Set::getName() { return name; } void Set::setName(char nam) { name=toupper(nam); } //add element to set void Set::addElement (int element) { if(isMember(element)==false) { for( int i =0; i { if(set[i] > element) { int valueToPush =set[i]; set[i] = element; element = valueToPush; } } set[numElements] = element; numElements++; } } //remove element from set void Set::removeElement(int element) { int index; if(isMember(element))//element found then remove from set { for(int i=0; i { if(set[i]==element) { for(int j=i; j<(numElements-1); j++) { set[j]=set[j+1]; } break; } } numElements--; } else { cout<<"Element not found in Set"< } } //check for membership bool Set::isMember(int element) { for(int i=0;i { if(set[i]==element)//element found then return true return true; } return false;//otherwise false } //size of set int Set::size() { return numElements; } //get element i int Set::getElement (int i) { return set[i]; } bool Set::operator==(Set& s) //equality comparison { if(size()!=s.size()) return false; else { for(int i=0;i { int val=s.getElement(i); if(set[i]!=val)//if val is not member of set { return false;//then return false } } return true;//then return false } } bool Set::operator !=(Set& s) //inequality comparion { if(size()!=s.size()) return true; else { for(int i=0;i { int val=s.getElement(i); if(set[i]!=val)//if val is not member of set { return true;//then return true } } return false;//then return false } } bool Set::operator - (int element) //remove element operator { if(isMember(element)) { removeElement(element); return true; } return false; } Set Set::operator - (Set& s) //aset difference operator { Set result;//create result set for(int i=0;i { int val=s.getElement(i); if(!isMember(val))//if val is not member of set { result.addElement(val);//then remove } } return result; } Set Set::operator ^ ( Set& s)//to represent the intersection of two sets { Set result;//create result set for(int j=0;j for(int i=0;i if (getElement(i) == s.getElement(j)) { s.addElement(getElement(i)); break; } } } return result; } bool Set::operator +(int element) //add element operator { if(!isMember(element)) { addElement(element); return true; } return false; } Set Set::operator +(Set& s)// set union opreator { Set result;//create result set for(int i=0;i { result.addElement(getElement(i)); } for(int i=0;i { result.addElement(s.getElement(i)); } return result;//return Combine two sets } SetDriver.cpp: // FILE COMMENT BLOCK //SetDriver.cpp // External references (i.e. include statements) #include #include #include #include // Class Definition #include "Set.h" using namespace std; // // Sample Driver Function // // Note that your program should re-display the // set each time the set is altered in any fashion! void printSet(Set s) { cout<<"Set "< for(int i=0;i { cout< } cout<<"}"< } int main() { // create two arrays of integers int a[]={1,4,2,5,3}; int b[]={3,4,5,6}; // Instantiate two sets {A, B} using each of the integer arrays previously declared Set s1(a,5,'a'); Set s2(b,4,'b'); printSet(s1); printSet(s2); // Declare a set C using the default constructor Set c; // Input elements into this set from external user input // using the >> operator as int ele; c.setName('c'); do { cout<<" Enter element to insert, -1 for exit: "; cin>>ele;// cin >> C; if(ele!=-1) c.addElement(ele); }while (ele!=-1); // Create a new set D from one of the above sets Set d(s1); d.setName('d'); printSet(c); printSet(d); // Add new element(s) to one of the existing sets. // Example: // A + element if(s1+7) cout<<" Element 7 add to set A successfully"< else cout<<" Element 7 alredy in set A"< printSet(s1); // Remove element(s) from one the existing sets. // Example: // C - element if(c-2) cout<<" Element 2 removed from set c successfully"< else cout<<" Element 2 not exist in the setc"< printSet(c); // Perform the set operations on any of the above objects // Note the operations must be invoked using the // appropriate operators. Example: // where set Z is the union of sets A and B. Set z; z.setName('z'); z = s1 + s2; printSet(z); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
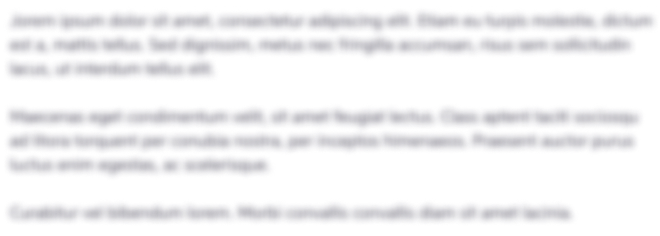
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started